Big Transfer Image Classification Model Quantization pipeline with NNCF¶
This Jupyter notebook can be launched after a local installation only.
This tutorial demonstrates the Quantization of the Big Transfer Image Classification model, which is fine-tuned on the sub-set of ImageNet dataset with 10 class labels with NNCF. It uses BiT-M-R50x1/1 model, which is trained on ImageNet-21k. Big Transfer is a recipe for pre-training image classification models on large supervised datasets and efficiently fine-tuning them on any given target task. The recipe achieves excellent performance on a wide variety of tasks, even when using very few labeled examples from the target dataset. This tutorial uses OpenVINO backend for performing model quantization in NNCF.
Table of contents:¶
%pip uninstall -q -y openvino-dev openvino openvino-nightly
%pip install -q "openvino-nightly" "nncf>=2.7.0" "tensorflow-hub>=0.15.0" "tensorflow_datasets"
%pip install -q "scikit-learn>=1.3.2"
WARNING: Skipping openvino-nightly as it is not installed.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
Note: you may need to restart the kernel to use updated packages.
import os
import sys
import numpy as np
from pathlib import Path
from openvino.runtime import Core
import openvino as ov
import nncf
import logging
sys.path.append("../utils")
from nncf.common.logging.logger import set_log_level
set_log_level(logging.ERROR)
from sklearn.metrics import accuracy_score
import tensorflow as tf
import tensorflow_datasets as tfds
import tensorflow_hub as hub
tfds.core.utils.gcs_utils._is_gcs_disabled = True
os.environ['NO_GCE_CHECK'] = 'true'
INFO:nncf:NNCF initialized successfully. Supported frameworks detected: torch, tensorflow, onnx, openvino
2024-02-09 23:12:32.801218: I tensorflow/core/util/port.cc:110] oneDNN custom operations are on. You may see slightly different numerical results due to floating-point round-off errors from different computation orders. To turn them off, set the environment variable TF_ENABLE_ONEDNN_OPTS=0. 2024-02-09 23:12:32.834847: I tensorflow/core/platform/cpu_feature_guard.cc:182] This TensorFlow binary is optimized to use available CPU instructions in performance-critical operations. To enable the following instructions: AVX2 AVX512F AVX512_VNNI FMA, in other operations, rebuild TensorFlow with the appropriate compiler flags.
2024-02-09 23:12:33.475171: W tensorflow/compiler/tf2tensorrt/utils/py_utils.cc:38] TF-TRT Warning: Could not find TensorRT
core = Core()
tf.compat.v1.logging.set_verbosity(tf.compat.v1.logging.ERROR)
os.environ['TF_CPP_MIN_LOG_LEVEL'] = '2'
# For top 5 labels.
MAX_PREDS = 1
TRAINING_BATCH_SIZE = 128
BATCH_SIZE = 1
IMG_SIZE = (256, 256) # Default Imagenet image size
NUM_CLASSES = 10 # For Imagenette dataset
FINE_TUNING_STEPS = 1
LR = 1e-5
MEAN_RGB = (0.485 * 255, 0.456 * 255, 0.406 * 255) # From Imagenet dataset
STDDEV_RGB = (0.229 * 255, 0.224 * 255, 0.225 * 255) # From Imagenet dataset
Prepare Dataset¶
datasets, datasets_info = tfds.load('imagenette/160px', shuffle_files=True, as_supervised=True, with_info=True,
read_config=tfds.ReadConfig(shuffle_seed=0))
train_ds, validation_ds = datasets['train'], datasets['validation']
2024-02-09 23:12:36.325702: E tensorflow/compiler/xla/stream_executor/cuda/cuda_driver.cc:266] failed call to cuInit: CUDA_ERROR_COMPAT_NOT_SUPPORTED_ON_DEVICE: forward compatibility was attempted on non supported HW
2024-02-09 23:12:36.325736: I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:168] retrieving CUDA diagnostic information for host: iotg-dev-workstation-07
2024-02-09 23:12:36.325741: I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:175] hostname: iotg-dev-workstation-07
2024-02-09 23:12:36.325909: I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:199] libcuda reported version is: 470.223.2
2024-02-09 23:12:36.325927: I tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:203] kernel reported version is: 470.182.3
2024-02-09 23:12:36.325931: E tensorflow/compiler/xla/stream_executor/cuda/cuda_diagnostics.cc:312] kernel version 470.182.3 does not match DSO version 470.223.2 -- cannot find working devices in this configuration
def preprocessing(image, label):
image = tf.image.resize(image, IMG_SIZE)
image = tf.cast(image, tf.float32) / 255.0
label = tf.one_hot(label, NUM_CLASSES)
return image, label
train_dataset = (train_ds.map(preprocessing, num_parallel_calls=tf.data.experimental.AUTOTUNE)
.batch(TRAINING_BATCH_SIZE).prefetch(tf.data.experimental.AUTOTUNE))
validation_dataset = (validation_ds.map(preprocessing, num_parallel_calls=tf.data.experimental.AUTOTUNE)
.batch(TRAINING_BATCH_SIZE).prefetch(tf.data.experimental.AUTOTUNE))
# Class labels dictionary with imagenette sample names and classes
lbl_dict = dict(
n01440764='tench',
n02102040='English springer',
n02979186='cassette player',
n03000684='chain saw',
n03028079='church',
n03394916='French horn',
n03417042='garbage truck',
n03425413='gas pump',
n03445777='golf ball',
n03888257='parachute'
)
# Imagenette samples name index
class_idx_dict = ['n01440764', 'n02102040', 'n02979186', 'n03000684',
'n03028079', 'n03394916', 'n03417042', 'n03425413',
'n03445777', 'n03888257']
def label_func(key):
return lbl_dict[key]
Plotting data samples¶
import matplotlib.pyplot as plt
# Get the class labels from the dataset info
class_labels = datasets_info.features['label'].names
# Display labels along with the examples
num_examples_to_display = 4
fig, axes = plt.subplots(nrows=1, ncols=num_examples_to_display, figsize=(10, 5))
for i, (image, label_index) in enumerate(train_ds.take(num_examples_to_display)):
label_name = class_labels[label_index.numpy()]
axes[i].imshow(image.numpy())
axes[i].set_title(f"{label_func(label_name)}")
axes[i].axis('off')
plt.tight_layout()
plt.show()
2024-02-09 23:12:36.722857: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_3' with dtype int64 and shape [1]
[[{{node Placeholder/_3}}]]
2024-02-09 23:12:36.723230: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1]
[[{{node Placeholder/_4}}]]
2024-02-09 23:12:36.934317: W tensorflow/core/kernels/data/cache_dataset_ops.cc:856] The calling iterator did not fully read the dataset being cached. In order to avoid unexpected truncation of the dataset, the partially cached contents of the dataset will be discarded. This can happen if you have an input pipeline similar to dataset.cache().take(k).repeat(). You should use dataset.take(k).cache().repeat() instead.
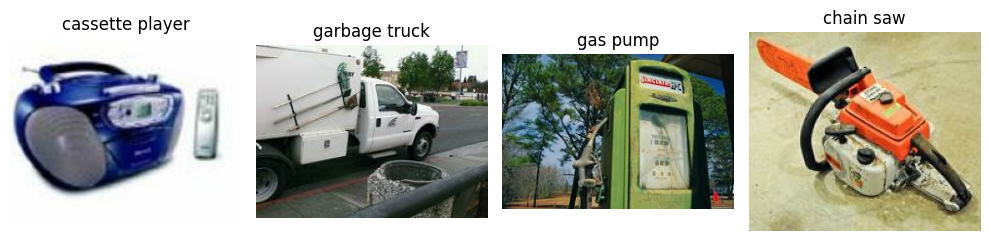
# Get the class labels from the dataset info
class_labels = datasets_info.features['label'].names
# Display labels along with the examples
num_examples_to_display = 4
fig, axes = plt.subplots(nrows=1, ncols=num_examples_to_display, figsize=(10, 5))
for i, (image, label_index) in enumerate(validation_ds.take(num_examples_to_display)):
label_name = class_labels[label_index.numpy()]
axes[i].imshow(image.numpy())
axes[i].set_title(f"{label_func(label_name)}")
axes[i].axis('off')
plt.tight_layout()
plt.show()
2024-02-09 23:12:37.419912: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1] [[{{node Placeholder/_4}}]] 2024-02-09 23:12:37.420269: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1] [[{{node Placeholder/_4}}]] 2024-02-09 23:12:37.609873: W tensorflow/core/kernels/data/cache_dataset_ops.cc:856] The calling iterator did not fully read the dataset being cached. In order to avoid unexpected truncation of the dataset, the partially cached contents of the dataset will be discarded. This can happen if you have an input pipeline similar to dataset.cache().take(k).repeat(). You should use dataset.take(k).cache().repeat() instead.
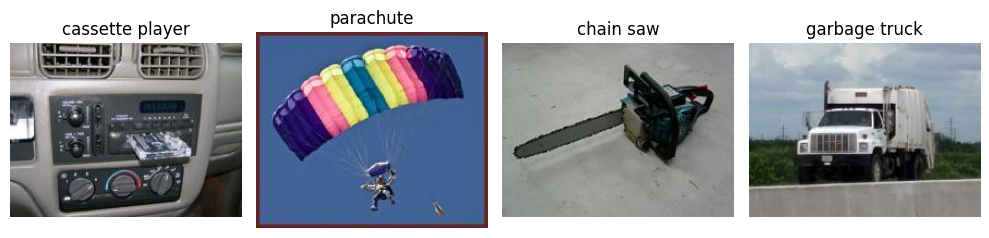
Model Fine-tuning¶
# Load the Big Transfer model
bit_model_url = "https://www.kaggle.com/models/google/bit/frameworks/TensorFlow2/variations/m-r50x1/versions/1"
bit_m = hub.KerasLayer(bit_model_url, trainable=True)
# Customize the model for the new task
model = tf.keras.Sequential([
bit_m,
tf.keras.layers.Dense(NUM_CLASSES, activation='softmax')
])
# Compile the model
model.compile(optimizer=tf.keras.optimizers.Adam(learning_rate=LR),
loss='categorical_crossentropy',
metrics=['accuracy'])
# Fine-tune the model
model.fit(train_dataset.take(3000),
epochs=FINE_TUNING_STEPS,
validation_data=validation_dataset.take(1000))
model.save("./bit_tf_model/", save_format='tf')
2024-02-09 23:12:46.261759: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1]
[[{{node Placeholder/_4}}]]
2024-02-09 23:12:46.262214: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_3' with dtype int64 and shape [1]
[[{{node Placeholder/_3}}]]
2024-02-09 23:12:46.814688: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/global_average_pooling2d/PartitionedCall_grad/global_average_pooling2d/PartitionedCall' with dtype float and shape [?,?,?,?]
[[{{node gradients/global_average_pooling2d/PartitionedCall_grad/global_average_pooling2d/PartitionedCall}}]]
2024-02-09 23:12:46.814757: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/global_average_pooling2d/PartitionedCall_grad/global_average_pooling2d/PartitionedCall_1' with dtype int32 and shape [2]
[[{{node gradients/global_average_pooling2d/PartitionedCall_grad/global_average_pooling2d/PartitionedCall_1}}]]
2024-02-09 23:12:46.873936: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall}}]]
2024-02-09 23:12:46.873995: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_1' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_1}}]]
2024-02-09 23:12:46.874031: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_2' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_2}}]]
2024-02-09 23:12:46.874063: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_3' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_3}}]]
2024-02-09 23:12:46.874094: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_4' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_4}}]]
2024-02-09 23:12:46.874125: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_5' with dtype float and shape [32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_5}}]]
2024-02-09 23:12:46.874156: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_6' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:46.874189: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_7' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:46.874221: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_8' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_8}}]]
2024-02-09 23:12:46.874252: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_9' with dtype float and shape [32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_9}}]]
2024-02-09 23:12:46.874284: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_10' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_10}}]]
2024-02-09 23:12:46.874317: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_11' with dtype float
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_11}}]]
2024-02-09 23:12:46.874350: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_12' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_12}}]]
2024-02-09 23:12:46.874382: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_13' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_13}}]]
2024-02-09 23:12:46.874414: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_14' with dtype float and shape [?,?,?,2048]
[[{{node gradients/group_norm/StatefulPartitionedCall_grad/group_norm/StatefulPartitionedCall_14}}]]
2024-02-09 23:12:47.623559: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall}}]]
2024-02-09 23:12:47.623635: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_1' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_1}}]]
2024-02-09 23:12:47.623673: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_2' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_2}}]]
2024-02-09 23:12:47.623706: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_6' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:47.623739: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_7' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:47.623770: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_8' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_8}}]]
2024-02-09 23:12:47.623818: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_9' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_9}}]]
2024-02-09 23:12:47.623851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_10' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_10}}]]
2024-02-09 23:12:47.623883: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_11' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_11}}]]
2024-02-09 23:12:47.623915: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_12' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_12}}]]
2024-02-09 23:12:47.623948: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_13' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_13}}]]
2024-02-09 23:12:47.623979: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_16' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_16}}]]
2024-02-09 23:12:47.624011: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_17' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_17}}]]
2024-02-09 23:12:47.624042: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_18' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_18}}]]
2024-02-09 23:12:47.624075: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_19' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_19}}]]
2024-02-09 23:12:47.624106: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_20' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_20}}]]
2024-02-09 23:12:47.624138: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_21' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_21}}]]
2024-02-09 23:12:47.624169: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_22' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_22}}]]
2024-02-09 23:12:47.624202: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_23' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_23}}]]
2024-02-09 23:12:47.624234: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_25' with dtype int32 and shape [4,2]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_25}}]]
2024-02-09 23:12:47.624265: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_26' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_26}}]]
2024-02-09 23:12:47.624297: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_29' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_29}}]]
2024-02-09 23:12:47.624329: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_30' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_30}}]]
2024-02-09 23:12:47.624363: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_31' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_31}}]]
2024-02-09 23:12:47.624394: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_32' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_32}}]]
2024-02-09 23:12:47.624429: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_33' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_33}}]]
2024-02-09 23:12:47.624463: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_34' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_34}}]]
2024-02-09 23:12:47.624497: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_35' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_35}}]]
2024-02-09 23:12:47.624531: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_38' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_38}}]]
2024-02-09 23:12:47.624565: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_39' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_39}}]]
2024-02-09 23:12:47.624598: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_40' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_40}}]]
2024-02-09 23:12:47.624631: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_41' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_41}}]]
2024-02-09 23:12:47.624664: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_42' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_42}}]]
2024-02-09 23:12:47.624699: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_43' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_43}}]]
2024-02-09 23:12:47.624732: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_44' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_44}}]]
2024-02-09 23:12:47.624766: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_45' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_45}}]]
2024-02-09 23:12:47.624799: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_46' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_46}}]]
2024-02-09 23:12:47.624833: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_50' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_50}}]]
2024-02-09 23:12:47.624867: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_51' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_51}}]]
2024-02-09 23:12:47.624901: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_52' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_52}}]]
2024-02-09 23:12:47.624934: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_53' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_53}}]]
2024-02-09 23:12:47.624968: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_54' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_54}}]]
2024-02-09 23:12:47.625001: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_55' with dtype float and shape [32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_55}}]]
2024-02-09 23:12:47.625036: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_56' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_56}}]]
2024-02-09 23:12:47.625070: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_57' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_57}}]]
2024-02-09 23:12:47.625103: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_60' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_60}}]]
2024-02-09 23:12:47.625137: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_61' with dtype float and shape [32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_61}}]]
2024-02-09 23:12:47.625170: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_62' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_62}}]]
2024-02-09 23:12:47.625203: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_63' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_63}}]]
2024-02-09 23:12:47.625237: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_64' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_64}}]]
2024-02-09 23:12:47.625271: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_65' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_65}}]]
2024-02-09 23:12:47.625304: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_66' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_66}}]]
2024-02-09 23:12:47.625338: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_67' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_67}}]]
2024-02-09 23:12:47.625371: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_68' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_68}}]]
2024-02-09 23:12:47.625415: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_72' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_72}}]]
2024-02-09 23:12:47.625452: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_73' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_73}}]]
2024-02-09 23:12:47.625504: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_74' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_74}}]]
2024-02-09 23:12:47.625538: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_75' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_75}}]]
2024-02-09 23:12:47.625572: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_76' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_76}}]]
2024-02-09 23:12:47.625606: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_77' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_77}}]]
2024-02-09 23:12:47.625639: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_78' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_78}}]]
2024-02-09 23:12:47.625673: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_79' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_79}}]]
2024-02-09 23:12:47.625706: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_82' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_82}}]]
2024-02-09 23:12:47.625739: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_83' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_83}}]]
2024-02-09 23:12:47.625773: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_84' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_84}}]]
2024-02-09 23:12:47.625806: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_85' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_85}}]]
2024-02-09 23:12:47.625840: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_86' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_86}}]]
2024-02-09 23:12:47.625874: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_87' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_87}}]]
2024-02-09 23:12:47.625908: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_88' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_88}}]]
2024-02-09 23:12:47.625941: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_89' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_89}}]]
2024-02-09 23:12:47.625974: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_91' with dtype int32 and shape [4,2]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_91}}]]
2024-02-09 23:12:47.626008: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_92' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_92}}]]
2024-02-09 23:12:47.626041: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_95' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_95}}]]
2024-02-09 23:12:47.626075: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_96' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_96}}]]
2024-02-09 23:12:47.626109: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_97' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_97}}]]
2024-02-09 23:12:47.626142: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_98' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_98}}]]
2024-02-09 23:12:47.626176: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_99' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_99}}]]
2024-02-09 23:12:47.626209: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_100' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_100}}]]
2024-02-09 23:12:47.626244: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_101' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_101}}]]
2024-02-09 23:12:47.626278: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_104' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_104}}]]
2024-02-09 23:12:47.626311: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_105' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_105}}]]
2024-02-09 23:12:47.626344: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_106' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_106}}]]
2024-02-09 23:12:47.626377: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_107' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_107}}]]
2024-02-09 23:12:47.626410: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_108' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_108}}]]
2024-02-09 23:12:47.626444: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_109' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_109}}]]
2024-02-09 23:12:47.626478: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_110' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_110}}]]
2024-02-09 23:12:47.626512: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_111' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_111}}]]
2024-02-09 23:12:47.626545: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_112' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_112}}]]
2024-02-09 23:12:47.626578: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_116' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_116}}]]
2024-02-09 23:12:47.626612: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_117' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_117}}]]
2024-02-09 23:12:47.626646: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_118' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_118}}]]
2024-02-09 23:12:47.626679: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_119' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_119}}]]
2024-02-09 23:12:47.626723: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_120' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_120}}]]
2024-02-09 23:12:47.626755: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_121' with dtype float and shape [32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_121}}]]
2024-02-09 23:12:47.626787: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_122' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_122}}]]
2024-02-09 23:12:47.626819: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_123' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_123}}]]
2024-02-09 23:12:47.626852: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_126' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_126}}]]
2024-02-09 23:12:47.626884: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_127' with dtype float and shape [32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_127}}]]
2024-02-09 23:12:47.626915: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_128' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_128}}]]
2024-02-09 23:12:47.626946: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_129' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_129}}]]
2024-02-09 23:12:47.626980: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_130' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_130}}]]
2024-02-09 23:12:47.627012: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_131' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_131}}]]
2024-02-09 23:12:47.627044: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_132' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_132}}]]
2024-02-09 23:12:47.627077: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_133' with dtype float and shape [?,?,?,2048]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_133}}]]
2024-02-09 23:12:47.627109: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_134' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_134}}]]
2024-02-09 23:12:47.627140: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_136' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_136}}]]
2024-02-09 23:12:47.627172: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_142' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_142}}]]
2024-02-09 23:12:47.627204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_143' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_143}}]]
2024-02-09 23:12:47.627236: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_144' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_144}}]]
2024-02-09 23:12:47.627267: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_145' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_145}}]]
2024-02-09 23:12:47.627299: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_146' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_146}}]]
2024-02-09 23:12:47.627331: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_147' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_147}}]]
2024-02-09 23:12:47.627364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_148' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_148}}]]
2024-02-09 23:12:47.627396: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_149' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_149}}]]
2024-02-09 23:12:47.627428: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_154' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_154}}]]
2024-02-09 23:12:47.627460: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_155' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_155}}]]
2024-02-09 23:12:47.627493: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_156' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_156}}]]
2024-02-09 23:12:47.627525: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_157' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_157}}]]
2024-02-09 23:12:47.627557: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_158' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_158}}]]
2024-02-09 23:12:47.627589: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_159' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_159}}]]
2024-02-09 23:12:47.627621: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_160' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_160}}]]
2024-02-09 23:12:47.627652: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_161' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_161}}]]
2024-02-09 23:12:47.627685: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_163' with dtype int32 and shape [4,2]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_163}}]]
2024-02-09 23:12:47.627718: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_164' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_164}}]]
2024-02-09 23:12:47.627750: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_167' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_167}}]]
2024-02-09 23:12:47.627782: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_168' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_168}}]]
2024-02-09 23:12:47.627813: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_169' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_169}}]]
2024-02-09 23:12:47.627845: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_170' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_170}}]]
2024-02-09 23:12:47.627877: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_171' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_171}}]]
2024-02-09 23:12:47.627909: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_172' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_172}}]]
2024-02-09 23:12:47.627942: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_173' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_173}}]]
2024-02-09 23:12:47.627974: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_176' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_176}}]]
2024-02-09 23:12:47.628005: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_177' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_177}}]]
2024-02-09 23:12:47.628038: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_178' with dtype float and shape [32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_178}}]]
2024-02-09 23:12:47.628070: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_179' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_179}}]]
2024-02-09 23:12:47.628102: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_180' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_180}}]]
2024-02-09 23:12:47.628134: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_181' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_181}}]]
2024-02-09 23:12:47.628166: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_182' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_182}}]]
2024-02-09 23:12:47.628197: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_183' with dtype float and shape [?,?,?,512]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_183}}]]
2024-02-09 23:12:47.628230: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_187' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_187}}]]
2024-02-09 23:12:47.628262: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_188' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_188}}]]
2024-02-09 23:12:47.628294: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_189' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_189}}]]
2024-02-09 23:12:47.628326: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_190' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_190}}]]
2024-02-09 23:12:47.628358: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_191' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_191}}]]
2024-02-09 23:12:47.628390: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_192' with dtype float and shape [32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_192}}]]
2024-02-09 23:12:47.628422: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_193' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_193}}]]
2024-02-09 23:12:47.628453: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_194' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_194}}]]
2024-02-09 23:12:47.628485: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_197' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_197}}]]
2024-02-09 23:12:47.628517: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_198' with dtype float and shape [32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_198}}]]
2024-02-09 23:12:47.628548: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_199' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_199}}]]
2024-02-09 23:12:47.628580: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_200' with dtype float
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_200}}]]
2024-02-09 23:12:47.628612: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_201' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_201}}]]
2024-02-09 23:12:47.628644: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_202' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_202}}]]
2024-02-09 23:12:47.628676: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_203' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block4/StatefulPartitionedCall_grad/block4/StatefulPartitionedCall_203}}]]
2024-02-09 23:12:49.091548: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall}}]]
2024-02-09 23:12:49.091625: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_1' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_1}}]]
2024-02-09 23:12:49.091662: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_2' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_2}}]]
2024-02-09 23:12:49.091696: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_6' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:49.091728: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_7' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:49.091760: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_8' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_8}}]]
2024-02-09 23:12:49.091791: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_9' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_9}}]]
2024-02-09 23:12:49.091823: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_10' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_10}}]]
2024-02-09 23:12:49.091855: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_11' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_11}}]]
2024-02-09 23:12:49.091886: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_12' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_12}}]]
2024-02-09 23:12:49.091917: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_13' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_13}}]]
2024-02-09 23:12:49.091949: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_16' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_16}}]]
2024-02-09 23:12:49.091980: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_17' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_17}}]]
2024-02-09 23:12:49.092010: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_18' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_18}}]]
2024-02-09 23:12:49.092043: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_19' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_19}}]]
2024-02-09 23:12:49.092074: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_20' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_20}}]]
2024-02-09 23:12:49.092105: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_21' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_21}}]]
2024-02-09 23:12:49.092136: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_22' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_22}}]]
2024-02-09 23:12:49.092166: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_23' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_23}}]]
2024-02-09 23:12:49.092199: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_25' with dtype int32 and shape [4,2]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_25}}]]
2024-02-09 23:12:49.092229: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_26' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_26}}]]
2024-02-09 23:12:49.092260: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_29' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_29}}]]
2024-02-09 23:12:49.092291: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_30' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_30}}]]
2024-02-09 23:12:49.092322: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_31' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_31}}]]
2024-02-09 23:12:49.092352: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_32' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_32}}]]
2024-02-09 23:12:49.092383: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_33' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_33}}]]
2024-02-09 23:12:49.092416: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_34' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_34}}]]
2024-02-09 23:12:49.092448: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_35' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_35}}]]
2024-02-09 23:12:49.092481: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_38' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_38}}]]
2024-02-09 23:12:49.092515: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_39' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_39}}]]
2024-02-09 23:12:49.092547: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_40' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_40}}]]
2024-02-09 23:12:49.092579: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_41' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_41}}]]
2024-02-09 23:12:49.092611: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_42' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_42}}]]
2024-02-09 23:12:49.092644: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_43' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_43}}]]
2024-02-09 23:12:49.092676: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_44' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_44}}]]
2024-02-09 23:12:49.092708: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_45' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_45}}]]
2024-02-09 23:12:49.092741: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_46' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_46}}]]
2024-02-09 23:12:49.092774: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_50' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_50}}]]
2024-02-09 23:12:49.092807: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_51' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_51}}]]
2024-02-09 23:12:49.092840: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_52' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_52}}]]
2024-02-09 23:12:49.092872: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_53' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_53}}]]
2024-02-09 23:12:49.092905: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_54' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_54}}]]
2024-02-09 23:12:49.092937: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_55' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_55}}]]
2024-02-09 23:12:49.092970: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_56' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_56}}]]
2024-02-09 23:12:49.093003: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_57' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_57}}]]
2024-02-09 23:12:49.093035: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_60' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_60}}]]
2024-02-09 23:12:49.093067: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_61' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_61}}]]
2024-02-09 23:12:49.093100: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_62' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_62}}]]
2024-02-09 23:12:49.093132: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_63' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_63}}]]
2024-02-09 23:12:49.093164: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_64' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_64}}]]
2024-02-09 23:12:49.093196: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_65' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_65}}]]
2024-02-09 23:12:49.093229: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_66' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_66}}]]
2024-02-09 23:12:49.093262: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_67' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_67}}]]
2024-02-09 23:12:49.093294: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_68' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_68}}]]
2024-02-09 23:12:49.093327: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_72' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_72}}]]
2024-02-09 23:12:49.093360: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_73' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_73}}]]
2024-02-09 23:12:49.093392: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_74' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_74}}]]
2024-02-09 23:12:49.093425: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_75' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_75}}]]
2024-02-09 23:12:49.093479: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_76' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_76}}]]
2024-02-09 23:12:49.093514: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_77' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_77}}]]
2024-02-09 23:12:49.093548: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_78' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_78}}]]
2024-02-09 23:12:49.093582: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_79' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_79}}]]
2024-02-09 23:12:49.093618: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_82' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_82}}]]
2024-02-09 23:12:49.093653: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_83' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_83}}]]
2024-02-09 23:12:49.093689: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_84' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_84}}]]
2024-02-09 23:12:49.093722: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_85' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_85}}]]
2024-02-09 23:12:49.093756: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_86' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_86}}]]
2024-02-09 23:12:49.093792: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_87' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_87}}]]
2024-02-09 23:12:49.093826: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_88' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_88}}]]
2024-02-09 23:12:49.093860: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_89' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_89}}]]
2024-02-09 23:12:49.093895: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_91' with dtype int32 and shape [4,2]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_91}}]]
2024-02-09 23:12:49.093929: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_92' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_92}}]]
2024-02-09 23:12:49.093963: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_95' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_95}}]]
2024-02-09 23:12:49.093997: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_96' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_96}}]]
2024-02-09 23:12:49.094031: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_97' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_97}}]]
2024-02-09 23:12:49.094066: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_98' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_98}}]]
2024-02-09 23:12:49.094100: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_99' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_99}}]]
2024-02-09 23:12:49.094136: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_100' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_100}}]]
2024-02-09 23:12:49.094171: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_101' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_101}}]]
2024-02-09 23:12:49.094205: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_104' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_104}}]]
2024-02-09 23:12:49.094240: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_105' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_105}}]]
2024-02-09 23:12:49.094275: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_106' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_106}}]]
2024-02-09 23:12:49.094309: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_107' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_107}}]]
2024-02-09 23:12:49.094343: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_108' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_108}}]]
2024-02-09 23:12:49.094377: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_109' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_109}}]]
2024-02-09 23:12:49.094412: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_110' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_110}}]]
2024-02-09 23:12:49.094446: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_111' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_111}}]]
2024-02-09 23:12:49.094481: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_112' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_112}}]]
2024-02-09 23:12:49.094516: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_116' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_116}}]]
2024-02-09 23:12:49.094550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_117' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_117}}]]
2024-02-09 23:12:49.094584: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_118' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_118}}]]
2024-02-09 23:12:49.094618: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_119' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_119}}]]
2024-02-09 23:12:49.094652: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_120' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_120}}]]
2024-02-09 23:12:49.094686: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_121' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_121}}]]
2024-02-09 23:12:49.094731: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_122' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_122}}]]
2024-02-09 23:12:49.094764: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_123' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_123}}]]
2024-02-09 23:12:49.094796: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_126' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_126}}]]
2024-02-09 23:12:49.094829: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_127' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_127}}]]
2024-02-09 23:12:49.094861: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_128' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_128}}]]
2024-02-09 23:12:49.094895: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_129' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_129}}]]
2024-02-09 23:12:49.094927: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_130' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_130}}]]
2024-02-09 23:12:49.094960: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_131' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_131}}]]
2024-02-09 23:12:49.094992: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_132' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_132}}]]
2024-02-09 23:12:49.095025: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_133' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_133}}]]
2024-02-09 23:12:49.095058: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_134' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_134}}]]
2024-02-09 23:12:49.095091: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_138' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_138}}]]
2024-02-09 23:12:49.095124: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_139' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_139}}]]
2024-02-09 23:12:49.095157: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_140' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_140}}]]
2024-02-09 23:12:49.095189: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_141' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_141}}]]
2024-02-09 23:12:49.095222: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_142' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_142}}]]
2024-02-09 23:12:49.095255: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_143' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_143}}]]
2024-02-09 23:12:49.095288: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_144' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_144}}]]
2024-02-09 23:12:49.095321: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_145' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_145}}]]
2024-02-09 23:12:49.095354: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_148' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_148}}]]
2024-02-09 23:12:49.095387: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_149' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_149}}]]
2024-02-09 23:12:49.095420: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_150' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_150}}]]
2024-02-09 23:12:49.095453: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_151' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_151}}]]
2024-02-09 23:12:49.095486: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_152' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_152}}]]
2024-02-09 23:12:49.095519: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_153' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_153}}]]
2024-02-09 23:12:49.095552: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_154' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_154}}]]
2024-02-09 23:12:49.095585: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_155' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_155}}]]
2024-02-09 23:12:49.095618: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_157' with dtype int32 and shape [4,2]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_157}}]]
2024-02-09 23:12:49.095651: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_158' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_158}}]]
2024-02-09 23:12:49.095684: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_161' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_161}}]]
2024-02-09 23:12:49.095716: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_162' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_162}}]]
2024-02-09 23:12:49.095749: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_163' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_163}}]]
2024-02-09 23:12:49.095782: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_164' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_164}}]]
2024-02-09 23:12:49.095815: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_165' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_165}}]]
2024-02-09 23:12:49.095848: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_166' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_166}}]]
2024-02-09 23:12:49.095881: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_167' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_167}}]]
2024-02-09 23:12:49.095914: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_170' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_170}}]]
2024-02-09 23:12:49.095947: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_171' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_171}}]]
2024-02-09 23:12:49.095980: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_172' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_172}}]]
2024-02-09 23:12:49.096013: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_173' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_173}}]]
2024-02-09 23:12:49.096045: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_174' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_174}}]]
2024-02-09 23:12:49.096078: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_175' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_175}}]]
2024-02-09 23:12:49.096111: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_176' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_176}}]]
2024-02-09 23:12:49.096143: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_177' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_177}}]]
2024-02-09 23:12:49.096176: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_178' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_178}}]]
2024-02-09 23:12:49.096209: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_182' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_182}}]]
2024-02-09 23:12:49.096242: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_183' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_183}}]]
2024-02-09 23:12:49.096275: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_184' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_184}}]]
2024-02-09 23:12:49.096307: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_185' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_185}}]]
2024-02-09 23:12:49.096340: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_186' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_186}}]]
2024-02-09 23:12:49.096373: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_187' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_187}}]]
2024-02-09 23:12:49.096406: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_188' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_188}}]]
2024-02-09 23:12:49.096439: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_189' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_189}}]]
2024-02-09 23:12:49.096473: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_192' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_192}}]]
2024-02-09 23:12:49.096505: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_193' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_193}}]]
2024-02-09 23:12:49.096536: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_194' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_194}}]]
2024-02-09 23:12:49.096569: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_195' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_195}}]]
2024-02-09 23:12:49.096601: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_196' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_196}}]]
2024-02-09 23:12:49.096635: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_197' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_197}}]]
2024-02-09 23:12:49.096667: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_198' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_198}}]]
2024-02-09 23:12:49.096701: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_199' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_199}}]]
2024-02-09 23:12:49.096733: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_200' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_200}}]]
2024-02-09 23:12:49.096766: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_204' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_204}}]]
2024-02-09 23:12:49.096799: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_205' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_205}}]]
2024-02-09 23:12:49.096832: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_206' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_206}}]]
2024-02-09 23:12:49.096865: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_207' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_207}}]]
2024-02-09 23:12:49.096897: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_208' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_208}}]]
2024-02-09 23:12:49.096929: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_209' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_209}}]]
2024-02-09 23:12:49.096961: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_210' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_210}}]]
2024-02-09 23:12:49.096993: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_211' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_211}}]]
2024-02-09 23:12:49.097027: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_214' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_214}}]]
2024-02-09 23:12:49.097059: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_215' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_215}}]]
2024-02-09 23:12:49.097092: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_216' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_216}}]]
2024-02-09 23:12:49.097124: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_217' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_217}}]]
2024-02-09 23:12:49.097156: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_218' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_218}}]]
2024-02-09 23:12:49.097188: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_219' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_219}}]]
2024-02-09 23:12:49.097221: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_220' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_220}}]]
2024-02-09 23:12:49.097254: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_221' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_221}}]]
2024-02-09 23:12:49.097286: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_223' with dtype int32 and shape [4,2]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_223}}]]
2024-02-09 23:12:49.097317: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_224' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_224}}]]
2024-02-09 23:12:49.097350: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_227' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_227}}]]
2024-02-09 23:12:49.097383: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_228' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_228}}]]
2024-02-09 23:12:49.097416: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_229' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_229}}]]
2024-02-09 23:12:49.097454: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_230' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_230}}]]
2024-02-09 23:12:49.097506: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_231' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_231}}]]
2024-02-09 23:12:49.097540: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_232' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_232}}]]
2024-02-09 23:12:49.097573: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_233' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_233}}]]
2024-02-09 23:12:49.097607: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_236' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_236}}]]
2024-02-09 23:12:49.097642: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_237' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_237}}]]
2024-02-09 23:12:49.097677: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_238' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_238}}]]
2024-02-09 23:12:49.097710: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_239' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_239}}]]
2024-02-09 23:12:49.097744: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_240' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_240}}]]
2024-02-09 23:12:49.097777: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_241' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_241}}]]
2024-02-09 23:12:49.097811: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_242' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_242}}]]
2024-02-09 23:12:49.097845: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_243' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_243}}]]
2024-02-09 23:12:49.097879: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_244' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_244}}]]
2024-02-09 23:12:49.097912: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_248' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_248}}]]
2024-02-09 23:12:49.097946: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_249' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_249}}]]
2024-02-09 23:12:49.097980: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_250' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_250}}]]
2024-02-09 23:12:49.098014: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_251' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_251}}]]
2024-02-09 23:12:49.098049: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_252' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_252}}]]
2024-02-09 23:12:49.098083: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_253' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_253}}]]
2024-02-09 23:12:49.098117: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_254' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_254}}]]
2024-02-09 23:12:49.098151: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_255' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_255}}]]
2024-02-09 23:12:49.098185: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_258' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_258}}]]
2024-02-09 23:12:49.098218: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_259' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_259}}]]
2024-02-09 23:12:49.098253: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_260' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_260}}]]
2024-02-09 23:12:49.098287: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_261' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_261}}]]
2024-02-09 23:12:49.098322: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_262' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_262}}]]
2024-02-09 23:12:49.098356: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_263' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_263}}]]
2024-02-09 23:12:49.098389: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_264' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_264}}]]
2024-02-09 23:12:49.098422: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_265' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_265}}]]
2024-02-09 23:12:49.098456: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_266' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_266}}]]
2024-02-09 23:12:49.098489: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_270' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_270}}]]
2024-02-09 23:12:49.098523: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_271' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_271}}]]
2024-02-09 23:12:49.098557: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_272' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_272}}]]
2024-02-09 23:12:49.098590: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_273' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_273}}]]
2024-02-09 23:12:49.098624: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_274' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_274}}]]
2024-02-09 23:12:49.098658: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_275' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_275}}]]
2024-02-09 23:12:49.098702: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_276' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_276}}]]
2024-02-09 23:12:49.098736: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_277' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_277}}]]
2024-02-09 23:12:49.098769: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_280' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_280}}]]
2024-02-09 23:12:49.098801: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_281' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_281}}]]
2024-02-09 23:12:49.098833: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_282' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_282}}]]
2024-02-09 23:12:49.098866: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_283' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_283}}]]
2024-02-09 23:12:49.098899: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_284' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_284}}]]
2024-02-09 23:12:49.098933: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_285' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_285}}]]
2024-02-09 23:12:49.098964: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_286' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_286}}]]
2024-02-09 23:12:49.098996: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_287' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_287}}]]
2024-02-09 23:12:49.099029: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_289' with dtype int32 and shape [4,2]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_289}}]]
2024-02-09 23:12:49.099061: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_290' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_290}}]]
2024-02-09 23:12:49.099093: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_293' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_293}}]]
2024-02-09 23:12:49.099125: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_294' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_294}}]]
2024-02-09 23:12:49.099157: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_295' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_295}}]]
2024-02-09 23:12:49.099189: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_296' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_296}}]]
2024-02-09 23:12:49.099222: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_297' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_297}}]]
2024-02-09 23:12:49.099255: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_298' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_298}}]]
2024-02-09 23:12:49.099288: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_299' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_299}}]]
2024-02-09 23:12:49.099320: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_302' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_302}}]]
2024-02-09 23:12:49.099351: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_303' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_303}}]]
2024-02-09 23:12:49.099384: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_304' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_304}}]]
2024-02-09 23:12:49.099416: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_305' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_305}}]]
2024-02-09 23:12:49.099448: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_306' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_306}}]]
2024-02-09 23:12:49.099481: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_307' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_307}}]]
2024-02-09 23:12:49.099514: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_308' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_308}}]]
2024-02-09 23:12:49.099546: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_309' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_309}}]]
2024-02-09 23:12:49.099577: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_310' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_310}}]]
2024-02-09 23:12:49.099610: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_314' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_314}}]]
2024-02-09 23:12:49.099644: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_315' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_315}}]]
2024-02-09 23:12:49.099676: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_316' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_316}}]]
2024-02-09 23:12:49.099708: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_317' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_317}}]]
2024-02-09 23:12:49.099741: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_318' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_318}}]]
2024-02-09 23:12:49.099773: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_319' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_319}}]]
2024-02-09 23:12:49.099805: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_320' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_320}}]]
2024-02-09 23:12:49.099837: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_321' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_321}}]]
2024-02-09 23:12:49.099869: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_324' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_324}}]]
2024-02-09 23:12:49.099901: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_325' with dtype float and shape [32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_325}}]]
2024-02-09 23:12:49.099934: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_326' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_326}}]]
2024-02-09 23:12:49.099966: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_327' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_327}}]]
2024-02-09 23:12:49.099999: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_328' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_328}}]]
2024-02-09 23:12:49.100032: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_329' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_329}}]]
2024-02-09 23:12:49.100064: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_330' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_330}}]]
2024-02-09 23:12:49.100098: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_331' with dtype float and shape [?,?,?,1024]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_331}}]]
2024-02-09 23:12:49.100130: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_332' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_332}}]]
2024-02-09 23:12:49.100163: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_334' with dtype float and shape [?,?,?,512]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_334}}]]
2024-02-09 23:12:49.100195: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_340' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_340}}]]
2024-02-09 23:12:49.100228: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_341' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_341}}]]
2024-02-09 23:12:49.100261: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_342' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_342}}]]
2024-02-09 23:12:49.100293: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_343' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_343}}]]
2024-02-09 23:12:49.100326: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_344' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_344}}]]
2024-02-09 23:12:49.100358: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_345' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_345}}]]
2024-02-09 23:12:49.100391: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_346' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_346}}]]
2024-02-09 23:12:49.100424: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_347' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_347}}]]
2024-02-09 23:12:49.100457: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_352' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_352}}]]
2024-02-09 23:12:49.100490: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_353' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_353}}]]
2024-02-09 23:12:49.100522: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_354' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_354}}]]
2024-02-09 23:12:49.100554: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_355' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_355}}]]
2024-02-09 23:12:49.100587: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_356' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_356}}]]
2024-02-09 23:12:49.100620: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_357' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_357}}]]
2024-02-09 23:12:49.100652: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_358' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_358}}]]
2024-02-09 23:12:49.100685: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_359' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_359}}]]
2024-02-09 23:12:49.100717: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_361' with dtype int32 and shape [4,2]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_361}}]]
2024-02-09 23:12:49.100750: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_362' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_362}}]]
2024-02-09 23:12:49.100782: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_365' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_365}}]]
2024-02-09 23:12:49.100815: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_366' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_366}}]]
2024-02-09 23:12:49.100847: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_367' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_367}}]]
2024-02-09 23:12:49.100880: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_368' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_368}}]]
2024-02-09 23:12:49.100912: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_369' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_369}}]]
2024-02-09 23:12:49.100944: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_370' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_370}}]]
2024-02-09 23:12:49.100977: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_371' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_371}}]]
2024-02-09 23:12:49.101011: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_374' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_374}}]]
2024-02-09 23:12:49.101044: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_375' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_375}}]]
2024-02-09 23:12:49.101076: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_376' with dtype float and shape [32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_376}}]]
2024-02-09 23:12:49.101108: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_377' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_377}}]]
2024-02-09 23:12:49.101141: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_378' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_378}}]]
2024-02-09 23:12:49.101174: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_379' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_379}}]]
2024-02-09 23:12:49.101206: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_380' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_380}}]]
2024-02-09 23:12:49.101240: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_381' with dtype float and shape [?,?,?,256]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_381}}]]
2024-02-09 23:12:49.101272: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_385' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_385}}]]
2024-02-09 23:12:49.101305: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_386' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_386}}]]
2024-02-09 23:12:49.101337: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_387' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_387}}]]
2024-02-09 23:12:49.101369: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_388' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_388}}]]
2024-02-09 23:12:49.101402: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_389' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_389}}]]
2024-02-09 23:12:49.101439: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_390' with dtype float and shape [32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_390}}]]
2024-02-09 23:12:49.101489: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_391' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_391}}]]
2024-02-09 23:12:49.101523: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_392' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_392}}]]
2024-02-09 23:12:49.101556: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_395' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_395}}]]
2024-02-09 23:12:49.101590: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_396' with dtype float and shape [32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_396}}]]
2024-02-09 23:12:49.101624: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_397' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_397}}]]
2024-02-09 23:12:49.101658: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_398' with dtype float
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_398}}]]
2024-02-09 23:12:49.101693: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_399' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_399}}]]
2024-02-09 23:12:49.101726: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_400' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_400}}]]
2024-02-09 23:12:49.101760: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_401' with dtype float and shape [?,?,?,512]
[[{{node gradients/block3/StatefulPartitionedCall_grad/block3/StatefulPartitionedCall_401}}]]
2024-02-09 23:12:50.091563: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall}}]]
2024-02-09 23:12:50.091636: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_1' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_1}}]]
2024-02-09 23:12:50.091671: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_2' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_2}}]]
2024-02-09 23:12:50.091705: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_6' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:50.091737: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_7' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:50.091768: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_8' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_8}}]]
2024-02-09 23:12:50.091800: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_9' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_9}}]]
2024-02-09 23:12:50.091831: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_10' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_10}}]]
2024-02-09 23:12:50.091862: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_11' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_11}}]]
2024-02-09 23:12:50.091893: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_12' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_12}}]]
2024-02-09 23:12:50.091923: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_13' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_13}}]]
2024-02-09 23:12:50.091955: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_16' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_16}}]]
2024-02-09 23:12:50.091986: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_17' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_17}}]]
2024-02-09 23:12:50.092016: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_18' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_18}}]]
2024-02-09 23:12:50.092047: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_19' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_19}}]]
2024-02-09 23:12:50.092078: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_20' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_20}}]]
2024-02-09 23:12:50.092108: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_21' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_21}}]]
2024-02-09 23:12:50.092138: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_22' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_22}}]]
2024-02-09 23:12:50.092168: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_23' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_23}}]]
2024-02-09 23:12:50.092199: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_25' with dtype int32 and shape [4,2]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_25}}]]
2024-02-09 23:12:50.092229: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_26' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_26}}]]
2024-02-09 23:12:50.092259: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_29' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_29}}]]
2024-02-09 23:12:50.092289: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_30' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_30}}]]
2024-02-09 23:12:50.092319: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_31' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_31}}]]
2024-02-09 23:12:50.092350: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_32' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_32}}]]
2024-02-09 23:12:50.092379: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_33' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_33}}]]
2024-02-09 23:12:50.092413: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_34' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_34}}]]
2024-02-09 23:12:50.092445: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_35' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_35}}]]
2024-02-09 23:12:50.092478: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_38' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_38}}]]
2024-02-09 23:12:50.092510: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_39' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_39}}]]
2024-02-09 23:12:50.092542: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_40' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_40}}]]
2024-02-09 23:12:50.092575: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_41' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_41}}]]
2024-02-09 23:12:50.092607: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_42' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_42}}]]
2024-02-09 23:12:50.092640: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_43' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_43}}]]
2024-02-09 23:12:50.092671: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_44' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_44}}]]
2024-02-09 23:12:50.092704: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_45' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_45}}]]
2024-02-09 23:12:50.092736: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_46' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_46}}]]
2024-02-09 23:12:50.092768: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_50' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_50}}]]
2024-02-09 23:12:50.092800: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_51' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_51}}]]
2024-02-09 23:12:50.092832: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_52' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_52}}]]
2024-02-09 23:12:50.092864: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_53' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_53}}]]
2024-02-09 23:12:50.092897: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_54' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_54}}]]
2024-02-09 23:12:50.092929: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_55' with dtype float and shape [32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_55}}]]
2024-02-09 23:12:50.092961: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_56' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_56}}]]
2024-02-09 23:12:50.092993: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_57' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_57}}]]
2024-02-09 23:12:50.093024: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_60' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_60}}]]
2024-02-09 23:12:50.093056: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_61' with dtype float and shape [32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_61}}]]
2024-02-09 23:12:50.093088: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_62' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_62}}]]
2024-02-09 23:12:50.093120: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_63' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_63}}]]
2024-02-09 23:12:50.093152: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_64' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_64}}]]
2024-02-09 23:12:50.093184: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_65' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_65}}]]
2024-02-09 23:12:50.093215: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_66' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_66}}]]
2024-02-09 23:12:50.093247: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_67' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_67}}]]
2024-02-09 23:12:50.093279: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_68' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_68}}]]
2024-02-09 23:12:50.093311: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_72' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_72}}]]
2024-02-09 23:12:50.093343: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_73' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_73}}]]
2024-02-09 23:12:50.093375: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_74' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_74}}]]
2024-02-09 23:12:50.093407: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_75' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_75}}]]
2024-02-09 23:12:50.093444: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_76' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_76}}]]
2024-02-09 23:12:50.093478: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_77' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_77}}]]
2024-02-09 23:12:50.093509: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_78' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_78}}]]
2024-02-09 23:12:50.093541: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_79' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_79}}]]
2024-02-09 23:12:50.093573: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_82' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_82}}]]
2024-02-09 23:12:50.093605: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_83' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_83}}]]
2024-02-09 23:12:50.093637: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_84' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_84}}]]
2024-02-09 23:12:50.093669: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_85' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_85}}]]
2024-02-09 23:12:50.093702: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_86' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_86}}]]
2024-02-09 23:12:50.093734: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_87' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_87}}]]
2024-02-09 23:12:50.093766: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_88' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_88}}]]
2024-02-09 23:12:50.093798: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_89' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_89}}]]
2024-02-09 23:12:50.093830: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_91' with dtype int32 and shape [4,2]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_91}}]]
2024-02-09 23:12:50.093862: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_92' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_92}}]]
2024-02-09 23:12:50.093894: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_95' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_95}}]]
2024-02-09 23:12:50.093926: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_96' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_96}}]]
2024-02-09 23:12:50.093958: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_97' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_97}}]]
2024-02-09 23:12:50.093990: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_98' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_98}}]]
2024-02-09 23:12:50.094022: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_99' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_99}}]]
2024-02-09 23:12:50.094053: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_100' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_100}}]]
2024-02-09 23:12:50.094085: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_101' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_101}}]]
2024-02-09 23:12:50.094117: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_104' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_104}}]]
2024-02-09 23:12:50.094149: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_105' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_105}}]]
2024-02-09 23:12:50.094181: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_106' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_106}}]]
2024-02-09 23:12:50.094213: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_107' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_107}}]]
2024-02-09 23:12:50.094245: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_108' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_108}}]]
2024-02-09 23:12:50.094277: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_109' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_109}}]]
2024-02-09 23:12:50.094309: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_110' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_110}}]]
2024-02-09 23:12:50.094341: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_111' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_111}}]]
2024-02-09 23:12:50.094373: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_112' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_112}}]]
2024-02-09 23:12:50.094406: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_116' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_116}}]]
2024-02-09 23:12:50.094437: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_117' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_117}}]]
2024-02-09 23:12:50.094469: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_118' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_118}}]]
2024-02-09 23:12:50.094501: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_119' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_119}}]]
2024-02-09 23:12:50.094533: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_120' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_120}}]]
2024-02-09 23:12:50.094564: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_121' with dtype float and shape [32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_121}}]]
2024-02-09 23:12:50.094596: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_122' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_122}}]]
2024-02-09 23:12:50.094628: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_123' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_123}}]]
2024-02-09 23:12:50.094659: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_126' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_126}}]]
2024-02-09 23:12:50.094691: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_127' with dtype float and shape [32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_127}}]]
2024-02-09 23:12:50.094723: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_128' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_128}}]]
2024-02-09 23:12:50.094755: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_129' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_129}}]]
2024-02-09 23:12:50.094787: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_130' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_130}}]]
2024-02-09 23:12:50.094819: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_131' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_131}}]]
2024-02-09 23:12:50.094851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_132' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_132}}]]
2024-02-09 23:12:50.094883: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_133' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_133}}]]
2024-02-09 23:12:50.094915: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_134' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_134}}]]
2024-02-09 23:12:50.094947: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_138' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_138}}]]
2024-02-09 23:12:50.094978: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_139' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_139}}]]
2024-02-09 23:12:50.095010: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_140' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_140}}]]
2024-02-09 23:12:50.095042: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_141' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_141}}]]
2024-02-09 23:12:50.095074: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_142' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_142}}]]
2024-02-09 23:12:50.095106: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_143' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_143}}]]
2024-02-09 23:12:50.095138: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_144' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_144}}]]
2024-02-09 23:12:50.095169: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_145' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_145}}]]
2024-02-09 23:12:50.095201: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_148' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_148}}]]
2024-02-09 23:12:50.095233: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_149' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_149}}]]
2024-02-09 23:12:50.095264: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_150' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_150}}]]
2024-02-09 23:12:50.095295: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_151' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_151}}]]
2024-02-09 23:12:50.095327: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_152' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_152}}]]
2024-02-09 23:12:50.095359: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_153' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_153}}]]
2024-02-09 23:12:50.095391: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_154' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_154}}]]
2024-02-09 23:12:50.095423: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_155' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_155}}]]
2024-02-09 23:12:50.095454: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_157' with dtype int32 and shape [4,2]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_157}}]]
2024-02-09 23:12:50.095486: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_158' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_158}}]]
2024-02-09 23:12:50.095518: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_161' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_161}}]]
2024-02-09 23:12:50.095550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_162' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_162}}]]
2024-02-09 23:12:50.095582: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_163' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_163}}]]
2024-02-09 23:12:50.095614: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_164' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_164}}]]
2024-02-09 23:12:50.095645: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_165' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_165}}]]
2024-02-09 23:12:50.095677: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_166' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_166}}]]
2024-02-09 23:12:50.095709: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_167' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_167}}]]
2024-02-09 23:12:50.095741: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_170' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_170}}]]
2024-02-09 23:12:50.095773: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_171' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_171}}]]
2024-02-09 23:12:50.095805: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_172' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_172}}]]
2024-02-09 23:12:50.095836: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_173' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_173}}]]
2024-02-09 23:12:50.095868: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_174' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_174}}]]
2024-02-09 23:12:50.095899: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_175' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_175}}]]
2024-02-09 23:12:50.095931: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_176' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_176}}]]
2024-02-09 23:12:50.095963: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_177' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_177}}]]
2024-02-09 23:12:50.095995: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_178' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_178}}]]
2024-02-09 23:12:50.096027: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_182' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_182}}]]
2024-02-09 23:12:50.096059: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_183' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_183}}]]
2024-02-09 23:12:50.096090: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_184' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_184}}]]
2024-02-09 23:12:50.096122: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_185' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_185}}]]
2024-02-09 23:12:50.096154: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_186' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_186}}]]
2024-02-09 23:12:50.096186: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_187' with dtype float and shape [32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_187}}]]
2024-02-09 23:12:50.096217: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_188' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_188}}]]
2024-02-09 23:12:50.096249: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_189' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_189}}]]
2024-02-09 23:12:50.096280: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_192' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_192}}]]
2024-02-09 23:12:50.096312: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_193' with dtype float and shape [32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_193}}]]
2024-02-09 23:12:50.096343: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_194' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_194}}]]
2024-02-09 23:12:50.096374: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_195' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_195}}]]
2024-02-09 23:12:50.096406: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_196' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_196}}]]
2024-02-09 23:12:50.096438: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_197' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_197}}]]
2024-02-09 23:12:50.096469: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_198' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_198}}]]
2024-02-09 23:12:50.096501: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_199' with dtype float and shape [?,?,?,512]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_199}}]]
2024-02-09 23:12:50.096532: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_200' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_200}}]]
2024-02-09 23:12:50.096564: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_202' with dtype float and shape [?,?,?,256]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_202}}]]
2024-02-09 23:12:50.096596: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_208' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_208}}]]
2024-02-09 23:12:50.096628: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_209' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_209}}]]
2024-02-09 23:12:50.096660: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_210' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_210}}]]
2024-02-09 23:12:50.096692: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_211' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_211}}]]
2024-02-09 23:12:50.096724: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_212' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_212}}]]
2024-02-09 23:12:50.096756: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_213' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_213}}]]
2024-02-09 23:12:50.096788: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_214' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_214}}]]
2024-02-09 23:12:50.096820: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_215' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_215}}]]
2024-02-09 23:12:50.096851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_220' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_220}}]]
2024-02-09 23:12:50.096883: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_221' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_221}}]]
2024-02-09 23:12:50.096915: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_222' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_222}}]]
2024-02-09 23:12:50.096947: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_223' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_223}}]]
2024-02-09 23:12:50.096979: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_224' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_224}}]]
2024-02-09 23:12:50.097012: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_225' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_225}}]]
2024-02-09 23:12:50.097044: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_226' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_226}}]]
2024-02-09 23:12:50.097076: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_227' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_227}}]]
2024-02-09 23:12:50.097108: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_229' with dtype int32 and shape [4,2]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_229}}]]
2024-02-09 23:12:50.097140: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_230' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_230}}]]
2024-02-09 23:12:50.097172: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_233' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_233}}]]
2024-02-09 23:12:50.097204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_234' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_234}}]]
2024-02-09 23:12:50.097236: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_235' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_235}}]]
2024-02-09 23:12:50.097268: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_236' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_236}}]]
2024-02-09 23:12:50.097299: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_237' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_237}}]]
2024-02-09 23:12:50.097332: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_238' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_238}}]]
2024-02-09 23:12:50.097364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_239' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_239}}]]
2024-02-09 23:12:50.097396: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_242' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_242}}]]
2024-02-09 23:12:50.097428: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_243' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_243}}]]
2024-02-09 23:12:50.097482: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_244' with dtype float and shape [32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_244}}]]
2024-02-09 23:12:50.097515: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_245' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_245}}]]
2024-02-09 23:12:50.097549: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_246' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_246}}]]
2024-02-09 23:12:50.097582: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_247' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_247}}]]
2024-02-09 23:12:50.097616: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_248' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_248}}]]
2024-02-09 23:12:50.097650: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_249' with dtype float and shape [?,?,?,128]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_249}}]]
2024-02-09 23:12:50.097683: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_253' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_253}}]]
2024-02-09 23:12:50.097717: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_254' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_254}}]]
2024-02-09 23:12:50.097750: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_255' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_255}}]]
2024-02-09 23:12:50.097784: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_256' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_256}}]]
2024-02-09 23:12:50.097818: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_257' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_257}}]]
2024-02-09 23:12:50.097851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_258' with dtype float and shape [32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_258}}]]
2024-02-09 23:12:50.097885: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_259' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_259}}]]
2024-02-09 23:12:50.097919: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_260' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_260}}]]
2024-02-09 23:12:50.097953: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_263' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_263}}]]
2024-02-09 23:12:50.097986: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_264' with dtype float and shape [32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_264}}]]
2024-02-09 23:12:50.098020: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_265' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_265}}]]
2024-02-09 23:12:50.098053: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_266' with dtype float
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_266}}]]
2024-02-09 23:12:50.098087: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_267' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_267}}]]
2024-02-09 23:12:50.098120: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_268' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_268}}]]
2024-02-09 23:12:50.098154: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_269' with dtype float and shape [?,?,?,256]
[[{{node gradients/block2/StatefulPartitionedCall_grad/block2/StatefulPartitionedCall_269}}]]
2024-02-09 23:12:50.847316: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall}}]]
2024-02-09 23:12:50.847388: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_1' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_1}}]]
2024-02-09 23:12:50.847426: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_2' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_2}}]]
2024-02-09 23:12:50.847460: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_6' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:50.847493: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_7' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:50.847526: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_8' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_8}}]]
2024-02-09 23:12:50.847559: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_9' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_9}}]]
2024-02-09 23:12:50.847601: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_10' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_10}}]]
2024-02-09 23:12:50.847632: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_11' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_11}}]]
2024-02-09 23:12:50.847663: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_12' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_12}}]]
2024-02-09 23:12:50.847695: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_13' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_13}}]]
2024-02-09 23:12:50.847726: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_16' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_16}}]]
2024-02-09 23:12:50.847756: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_17' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_17}}]]
2024-02-09 23:12:50.847787: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_18' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_18}}]]
2024-02-09 23:12:50.847817: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_19' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_19}}]]
2024-02-09 23:12:50.847848: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_20' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_20}}]]
2024-02-09 23:12:50.847878: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_21' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_21}}]]
2024-02-09 23:12:50.847908: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_22' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_22}}]]
2024-02-09 23:12:50.847947: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_23' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_23}}]]
2024-02-09 23:12:50.847977: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_25' with dtype int32 and shape [4,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_25}}]]
2024-02-09 23:12:50.848007: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_26' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_26}}]]
2024-02-09 23:12:50.848038: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_29' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_29}}]]
2024-02-09 23:12:50.848068: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_30' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_30}}]]
2024-02-09 23:12:50.848098: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_31' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_31}}]]
2024-02-09 23:12:50.848128: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_32' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_32}}]]
2024-02-09 23:12:50.848162: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_33' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_33}}]]
2024-02-09 23:12:50.848194: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_34' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_34}}]]
2024-02-09 23:12:50.848234: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_35' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_35}}]]
2024-02-09 23:12:50.848292: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_38' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_38}}]]
2024-02-09 23:12:50.848330: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_39' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_39}}]]
2024-02-09 23:12:50.848365: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_40' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_40}}]]
2024-02-09 23:12:50.848401: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_41' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_41}}]]
2024-02-09 23:12:50.848435: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_42' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_42}}]]
2024-02-09 23:12:50.848469: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_43' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_43}}]]
2024-02-09 23:12:50.848503: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_44' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_44}}]]
2024-02-09 23:12:50.848537: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_45' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_45}}]]
2024-02-09 23:12:50.848570: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_46' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_46}}]]
2024-02-09 23:12:50.848608: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_50' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_50}}]]
2024-02-09 23:12:50.848645: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_51' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_51}}]]
2024-02-09 23:12:50.848678: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_52' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_52}}]]
2024-02-09 23:12:50.848712: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_53' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_53}}]]
2024-02-09 23:12:50.848746: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_54' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_54}}]]
2024-02-09 23:12:50.848779: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_55' with dtype float and shape [32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_55}}]]
2024-02-09 23:12:50.848814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_56' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_56}}]]
2024-02-09 23:12:50.848848: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_57' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_57}}]]
2024-02-09 23:12:50.848881: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_60' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_60}}]]
2024-02-09 23:12:50.848915: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_61' with dtype float and shape [32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_61}}]]
2024-02-09 23:12:50.848950: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_62' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_62}}]]
2024-02-09 23:12:50.848982: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_63' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_63}}]]
2024-02-09 23:12:50.849017: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_64' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_64}}]]
2024-02-09 23:12:50.849050: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_65' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_65}}]]
2024-02-09 23:12:50.849083: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_66' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_66}}]]
2024-02-09 23:12:50.849117: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_67' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_67}}]]
2024-02-09 23:12:50.849151: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_68' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_68}}]]
2024-02-09 23:12:50.849184: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_72' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_72}}]]
2024-02-09 23:12:50.849218: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_73' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_73}}]]
2024-02-09 23:12:50.849252: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_74' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_74}}]]
2024-02-09 23:12:50.849286: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_75' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_75}}]]
2024-02-09 23:12:50.849319: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_76' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_76}}]]
2024-02-09 23:12:50.849353: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_77' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_77}}]]
2024-02-09 23:12:50.849387: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_78' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_78}}]]
2024-02-09 23:12:50.849420: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_79' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_79}}]]
2024-02-09 23:12:50.849460: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_82' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_82}}]]
2024-02-09 23:12:50.849511: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_83' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_83}}]]
2024-02-09 23:12:50.849544: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_84' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_84}}]]
2024-02-09 23:12:50.849579: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_85' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_85}}]]
2024-02-09 23:12:50.849614: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_86' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_86}}]]
2024-02-09 23:12:50.849648: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_87' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_87}}]]
2024-02-09 23:12:50.849682: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_88' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_88}}]]
2024-02-09 23:12:50.849717: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_89' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_89}}]]
2024-02-09 23:12:50.849757: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_91' with dtype int32 and shape [4,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_91}}]]
2024-02-09 23:12:50.849797: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_92' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_92}}]]
2024-02-09 23:12:50.849833: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_95' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_95}}]]
2024-02-09 23:12:50.849869: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_96' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_96}}]]
2024-02-09 23:12:50.849903: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_97' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_97}}]]
2024-02-09 23:12:50.849937: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_98' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_98}}]]
2024-02-09 23:12:50.849971: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_99' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_99}}]]
2024-02-09 23:12:50.850006: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_100' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_100}}]]
2024-02-09 23:12:50.850040: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_101' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_101}}]]
2024-02-09 23:12:50.850074: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_104' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_104}}]]
2024-02-09 23:12:50.850108: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_105' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_105}}]]
2024-02-09 23:12:50.850150: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_106' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_106}}]]
2024-02-09 23:12:50.850186: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_107' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_107}}]]
2024-02-09 23:12:50.850221: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_108' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_108}}]]
2024-02-09 23:12:50.850255: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_109' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_109}}]]
2024-02-09 23:12:50.850289: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_110' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_110}}]]
2024-02-09 23:12:50.850323: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_111' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_111}}]]
2024-02-09 23:12:50.850357: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_112' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_112}}]]
2024-02-09 23:12:50.850390: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_116' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_116}}]]
2024-02-09 23:12:50.850424: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_117' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_117}}]]
2024-02-09 23:12:50.850458: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_118' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_118}}]]
2024-02-09 23:12:50.850493: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_119' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_119}}]]
2024-02-09 23:12:50.850527: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_120' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_120}}]]
2024-02-09 23:12:50.850561: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_121' with dtype float and shape [32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_121}}]]
2024-02-09 23:12:50.850595: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_122' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_122}}]]
2024-02-09 23:12:50.850629: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_123' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_123}}]]
2024-02-09 23:12:50.850663: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_126' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_126}}]]
2024-02-09 23:12:50.850697: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_127' with dtype float and shape [32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_127}}]]
2024-02-09 23:12:50.850732: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_128' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_128}}]]
2024-02-09 23:12:50.850783: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_129' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_129}}]]
2024-02-09 23:12:50.850828: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_130' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_130}}]]
2024-02-09 23:12:50.850861: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_131' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_131}}]]
2024-02-09 23:12:50.850893: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_132' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_132}}]]
2024-02-09 23:12:50.850926: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_133' with dtype float and shape [?,?,?,256]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_133}}]]
2024-02-09 23:12:50.850957: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_134' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_134}}]]
2024-02-09 23:12:50.850989: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_136' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_136}}]]
2024-02-09 23:12:50.851021: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_142' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_142}}]]
2024-02-09 23:12:50.851053: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_143' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_143}}]]
2024-02-09 23:12:50.851085: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_144' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_144}}]]
2024-02-09 23:12:50.851117: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_145' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_145}}]]
2024-02-09 23:12:50.851149: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_146' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_146}}]]
2024-02-09 23:12:50.851180: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_147' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_147}}]]
2024-02-09 23:12:50.851212: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_148' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_148}}]]
2024-02-09 23:12:50.851243: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_149' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_149}}]]
2024-02-09 23:12:50.851276: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_154' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_154}}]]
2024-02-09 23:12:50.851308: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_155' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_155}}]]
2024-02-09 23:12:50.851340: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_156' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_156}}]]
2024-02-09 23:12:50.851371: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_157' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_157}}]]
2024-02-09 23:12:50.851403: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_158' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_158}}]]
2024-02-09 23:12:50.851435: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_159' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_159}}]]
2024-02-09 23:12:50.851467: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_160' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_160}}]]
2024-02-09 23:12:50.851499: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_161' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_161}}]]
2024-02-09 23:12:50.851531: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_163' with dtype int32 and shape [4,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_163}}]]
2024-02-09 23:12:50.851563: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_164' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_164}}]]
2024-02-09 23:12:50.851595: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_167' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_167}}]]
2024-02-09 23:12:50.851628: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_168' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_168}}]]
2024-02-09 23:12:50.851659: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_169' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_169}}]]
2024-02-09 23:12:50.851691: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_170' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_170}}]]
2024-02-09 23:12:50.851723: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_171' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_171}}]]
2024-02-09 23:12:50.851755: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_172' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_172}}]]
2024-02-09 23:12:50.851787: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_173' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_173}}]]
2024-02-09 23:12:50.851819: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_176' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_176}}]]
2024-02-09 23:12:50.851851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_177' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_177}}]]
2024-02-09 23:12:50.851883: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_178' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_178}}]]
2024-02-09 23:12:50.851915: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_179' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_179}}]]
2024-02-09 23:12:50.851947: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_180' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_180}}]]
2024-02-09 23:12:50.851979: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_181' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_181}}]]
2024-02-09 23:12:50.852011: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_182' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_182}}]]
2024-02-09 23:12:50.852043: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_183' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_183}}]]
2024-02-09 23:12:50.852075: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_187' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_187}}]]
2024-02-09 23:12:50.852107: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_188' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_188}}]]
2024-02-09 23:12:50.852140: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_189' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_189}}]]
2024-02-09 23:12:50.852172: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_190' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_190}}]]
2024-02-09 23:12:50.852204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_191' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_191}}]]
2024-02-09 23:12:50.852236: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_192' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_192}}]]
2024-02-09 23:12:50.852268: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_193' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_193}}]]
2024-02-09 23:12:50.852300: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_194' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_194}}]]
2024-02-09 23:12:50.852332: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_197' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_197}}]]
2024-02-09 23:12:50.852364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_198' with dtype float and shape [32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_198}}]]
2024-02-09 23:12:50.852396: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_199' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_199}}]]
2024-02-09 23:12:50.852428: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_200' with dtype float
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_200}}]]
2024-02-09 23:12:50.852460: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_201' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_201}}]]
2024-02-09 23:12:50.852492: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_202' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_202}}]]
2024-02-09 23:12:50.852525: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_203' with dtype float and shape [?,?,?,64]
[[{{node gradients/block1/StatefulPartitionedCall_grad/block1/StatefulPartitionedCall_203}}]]
2024-02-09 23:12:50.897333: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_2' with dtype int32 and shape [4,2]
[[{{node gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_2}}]]
2024-02-09 23:12:50.897390: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_3' with dtype float and shape [?,?,?,64]
[[{{node gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_3}}]]
2024-02-09 23:12:50.897426: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_4' with dtype float and shape [?,?,?,3]
[[{{node gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_4}}]]
2024-02-09 23:12:50.897480: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_6' with dtype int32 and shape [4,2]
[[{{node gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:50.897514: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_7' with dtype float and shape [?,?,?,3]
[[{{node gradients/root_block/StatefulPartitionedCall_grad/root_block/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:51.373057: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall' with dtype float and shape [?,?,?,?]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall}}]]
2024-02-09 23:12:51.373139: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1' with dtype int32 and shape [2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1}}]]
2024-02-09 23:12:51.373179: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_4' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_4}}]]
2024-02-09 23:12:51.373215: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_5' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_5}}]]
2024-02-09 23:12:51.373254: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_6' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:51.373287: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_7' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:51.373321: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_8' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_8}}]]
2024-02-09 23:12:51.373372: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_9' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_9}}]]
2024-02-09 23:12:51.373406: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_10' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_10}}]]
2024-02-09 23:12:51.373445: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_11' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_11}}]]
2024-02-09 23:12:51.373480: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_12' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_12}}]]
2024-02-09 23:12:51.373516: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_13' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_13}}]]
2024-02-09 23:12:51.373550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_14' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_14}}]]
2024-02-09 23:12:51.373584: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_15' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_15}}]]
2024-02-09 23:12:51.373618: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_16' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_16}}]]
2024-02-09 23:12:51.373651: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_17' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_17}}]]
2024-02-09 23:12:51.373685: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_18' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_18}}]]
2024-02-09 23:12:51.373719: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_19' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_19}}]]
2024-02-09 23:12:51.373751: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_20' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_20}}]]
2024-02-09 23:12:51.373784: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_21' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_21}}]]
2024-02-09 23:12:51.373819: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_25' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_25}}]]
2024-02-09 23:12:51.373852: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_26' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_26}}]]
2024-02-09 23:12:51.373884: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_27' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_27}}]]
2024-02-09 23:12:51.373918: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_28' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_28}}]]
2024-02-09 23:12:51.373951: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_29' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_29}}]]
2024-02-09 23:12:51.373984: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_30' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_30}}]]
2024-02-09 23:12:51.374017: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_31' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_31}}]]
2024-02-09 23:12:51.374054: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_32' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_32}}]]
2024-02-09 23:12:51.374090: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_35' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_35}}]]
2024-02-09 23:12:51.374125: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_36' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_36}}]]
2024-02-09 23:12:51.374162: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_37' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_37}}]]
2024-02-09 23:12:51.374197: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_38' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_38}}]]
2024-02-09 23:12:51.374233: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_39' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_39}}]]
2024-02-09 23:12:51.374268: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_40' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_40}}]]
2024-02-09 23:12:51.374303: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_41' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_41}}]]
2024-02-09 23:12:51.374338: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_42' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_42}}]]
2024-02-09 23:12:51.374373: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_44' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_44}}]]
2024-02-09 23:12:51.374408: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_45' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_45}}]]
2024-02-09 23:12:51.374443: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_48' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_48}}]]
2024-02-09 23:12:51.374478: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_49' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_49}}]]
2024-02-09 23:12:51.374513: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_50' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_50}}]]
2024-02-09 23:12:51.374547: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_51' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_51}}]]
2024-02-09 23:12:51.374582: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_52' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_52}}]]
2024-02-09 23:12:51.374617: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_53' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_53}}]]
2024-02-09 23:12:51.374652: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_54' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_54}}]]
2024-02-09 23:12:51.374687: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_57' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_57}}]]
2024-02-09 23:12:51.374722: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_58' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_58}}]]
2024-02-09 23:12:51.374757: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_59' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_59}}]]
2024-02-09 23:12:51.374792: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_60' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_60}}]]
2024-02-09 23:12:51.374827: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_61' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_61}}]]
2024-02-09 23:12:51.374861: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_62' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_62}}]]
2024-02-09 23:12:51.374895: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_63' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_63}}]]
2024-02-09 23:12:51.374930: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_64' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_64}}]]
2024-02-09 23:12:51.374965: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_65' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_65}}]]
2024-02-09 23:12:51.375010: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_69' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_69}}]]
2024-02-09 23:12:51.375043: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_70' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_70}}]]
2024-02-09 23:12:51.375078: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_71' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_71}}]]
2024-02-09 23:12:51.375111: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_72' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_72}}]]
2024-02-09 23:12:51.375145: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_73' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_73}}]]
2024-02-09 23:12:51.375179: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_74' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_74}}]]
2024-02-09 23:12:51.375212: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_75' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_75}}]]
2024-02-09 23:12:51.375246: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_76' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_76}}]]
2024-02-09 23:12:51.375279: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_79' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_79}}]]
2024-02-09 23:12:51.375313: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_80' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_80}}]]
2024-02-09 23:12:51.375347: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_81' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_81}}]]
2024-02-09 23:12:51.375381: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_82' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_82}}]]
2024-02-09 23:12:51.375415: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_83' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_83}}]]
2024-02-09 23:12:51.375450: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_84' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_84}}]]
2024-02-09 23:12:51.375483: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_85' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_85}}]]
2024-02-09 23:12:51.375517: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_86' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_86}}]]
2024-02-09 23:12:51.375550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_87' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_87}}]]
2024-02-09 23:12:51.375583: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_91' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_91}}]]
2024-02-09 23:12:51.375617: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_92' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_92}}]]
2024-02-09 23:12:51.375650: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_93' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_93}}]]
2024-02-09 23:12:51.375682: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_94' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_94}}]]
2024-02-09 23:12:51.375715: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_95' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_95}}]]
2024-02-09 23:12:51.375749: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_96' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_96}}]]
2024-02-09 23:12:51.375782: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_97' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_97}}]]
2024-02-09 23:12:51.375816: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_98' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_98}}]]
2024-02-09 23:12:51.375849: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_101' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_101}}]]
2024-02-09 23:12:51.375883: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_102' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_102}}]]
2024-02-09 23:12:51.375917: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_103' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_103}}]]
2024-02-09 23:12:51.375950: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_104' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_104}}]]
2024-02-09 23:12:51.375985: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_105' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_105}}]]
2024-02-09 23:12:51.376019: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_106' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_106}}]]
2024-02-09 23:12:51.376052: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_107' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_107}}]]
2024-02-09 23:12:51.376085: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_108' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_108}}]]
2024-02-09 23:12:51.376119: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_110' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_110}}]]
2024-02-09 23:12:51.376152: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_111' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_111}}]]
2024-02-09 23:12:51.376186: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_114' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_114}}]]
2024-02-09 23:12:51.376220: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_115' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_115}}]]
2024-02-09 23:12:51.376253: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_116' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_116}}]]
2024-02-09 23:12:51.376287: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_117' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_117}}]]
2024-02-09 23:12:51.376321: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_118' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_118}}]]
2024-02-09 23:12:51.376354: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_119' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_119}}]]
2024-02-09 23:12:51.376387: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_120' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_120}}]]
2024-02-09 23:12:51.376421: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_123' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_123}}]]
2024-02-09 23:12:51.376454: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_124' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_124}}]]
2024-02-09 23:12:51.376487: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_125' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_125}}]]
2024-02-09 23:12:51.376520: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_126' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_126}}]]
2024-02-09 23:12:51.376554: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_127' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_127}}]]
2024-02-09 23:12:51.376605: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_128' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_128}}]]
2024-02-09 23:12:51.376640: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_129' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_129}}]]
2024-02-09 23:12:51.376675: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_130' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_130}}]]
2024-02-09 23:12:51.376710: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_131' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_131}}]]
2024-02-09 23:12:51.376745: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_135' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_135}}]]
2024-02-09 23:12:51.376780: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_136' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_136}}]]
2024-02-09 23:12:51.376814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_137' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_137}}]]
2024-02-09 23:12:51.376849: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_138' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_138}}]]
2024-02-09 23:12:51.376884: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_139' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_139}}]]
2024-02-09 23:12:51.376919: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_140' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_140}}]]
2024-02-09 23:12:51.376953: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_141' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_141}}]]
2024-02-09 23:12:51.376988: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_142' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_142}}]]
2024-02-09 23:12:51.377023: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_145' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_145}}]]
2024-02-09 23:12:51.377058: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_146' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_146}}]]
2024-02-09 23:12:51.377093: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_147' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_147}}]]
2024-02-09 23:12:51.377128: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_148' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_148}}]]
2024-02-09 23:12:51.377162: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_149' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_149}}]]
2024-02-09 23:12:51.377197: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_150' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_150}}]]
2024-02-09 23:12:51.377233: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_151' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_151}}]]
2024-02-09 23:12:51.377268: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_152' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_152}}]]
2024-02-09 23:12:51.377303: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_153' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_153}}]]
2024-02-09 23:12:51.377338: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_155' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_155}}]]
2024-02-09 23:12:51.377373: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_161' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_161}}]]
2024-02-09 23:12:51.377409: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_162' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_162}}]]
2024-02-09 23:12:51.377449: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_163' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_163}}]]
2024-02-09 23:12:51.377485: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_164' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_164}}]]
2024-02-09 23:12:51.377520: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_165' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_165}}]]
2024-02-09 23:12:51.377555: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_166' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_166}}]]
2024-02-09 23:12:51.377591: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_167' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_167}}]]
2024-02-09 23:12:51.377627: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_168' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_168}}]]
2024-02-09 23:12:51.377662: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_173' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_173}}]]
2024-02-09 23:12:51.377697: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_174' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_174}}]]
2024-02-09 23:12:51.377732: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_175' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_175}}]]
2024-02-09 23:12:51.377767: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_176' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_176}}]]
2024-02-09 23:12:51.377802: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_177' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_177}}]]
2024-02-09 23:12:51.377847: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_178' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_178}}]]
2024-02-09 23:12:51.377881: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_179' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_179}}]]
2024-02-09 23:12:51.377914: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_180' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_180}}]]
2024-02-09 23:12:51.377947: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_182' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_182}}]]
2024-02-09 23:12:51.377981: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_183' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_183}}]]
2024-02-09 23:12:51.378015: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_186' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_186}}]]
2024-02-09 23:12:51.378048: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_187' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_187}}]]
2024-02-09 23:12:51.378082: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_188' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_188}}]]
2024-02-09 23:12:51.378116: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_189' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_189}}]]
2024-02-09 23:12:51.378149: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_190' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_190}}]]
2024-02-09 23:12:51.378182: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_191' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_191}}]]
2024-02-09 23:12:51.378216: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_192' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_192}}]]
2024-02-09 23:12:51.378250: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_195' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_195}}]]
2024-02-09 23:12:51.378283: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_196' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_196}}]]
2024-02-09 23:12:51.378317: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_197' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_197}}]]
2024-02-09 23:12:51.378351: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_198' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_198}}]]
2024-02-09 23:12:51.378385: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_199' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_199}}]]
2024-02-09 23:12:51.378418: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_200' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_200}}]]
2024-02-09 23:12:51.378452: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_201' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_201}}]]
2024-02-09 23:12:51.378485: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_202' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_202}}]]
2024-02-09 23:12:51.378518: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_206' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_206}}]]
2024-02-09 23:12:51.378552: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_207' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_207}}]]
2024-02-09 23:12:51.378585: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_208' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_208}}]]
2024-02-09 23:12:51.378619: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_209' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_209}}]]
2024-02-09 23:12:51.378653: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_210' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_210}}]]
2024-02-09 23:12:51.378686: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_211' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_211}}]]
2024-02-09 23:12:51.378719: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_212' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_212}}]]
2024-02-09 23:12:51.378753: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_213' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_213}}]]
2024-02-09 23:12:51.378787: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_216' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_216}}]]
2024-02-09 23:12:51.378820: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_217' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_217}}]]
2024-02-09 23:12:51.378853: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_218' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_218}}]]
2024-02-09 23:12:51.378886: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_219' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_219}}]]
2024-02-09 23:12:51.378920: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_220' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_220}}]]
2024-02-09 23:12:51.378953: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_221' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_221}}]]
2024-02-09 23:12:51.378988: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_222' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_222}}]]
2024-02-09 23:12:51.379021: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_223' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_223}}]]
2024-02-09 23:12:51.379055: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_224' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_224}}]]
2024-02-09 23:12:51.379088: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_225' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_225}}]]
2024-02-09 23:12:51.379122: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_229' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_229}}]]
2024-02-09 23:12:51.379155: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_230' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_230}}]]
2024-02-09 23:12:51.379190: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_231' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_231}}]]
2024-02-09 23:12:51.379223: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_232' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_232}}]]
2024-02-09 23:12:51.379257: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_233' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_233}}]]
2024-02-09 23:12:51.379291: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_234' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_234}}]]
2024-02-09 23:12:51.379325: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_235' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_235}}]]
2024-02-09 23:12:51.379358: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_236' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_236}}]]
2024-02-09 23:12:51.379392: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_239' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_239}}]]
2024-02-09 23:12:51.379426: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_240' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_240}}]]
2024-02-09 23:12:51.379459: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_241' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_241}}]]
2024-02-09 23:12:51.379492: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_242' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_242}}]]
2024-02-09 23:12:51.379526: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_243' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_243}}]]
2024-02-09 23:12:51.379560: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_244' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_244}}]]
2024-02-09 23:12:51.379593: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_245' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_245}}]]
2024-02-09 23:12:51.379627: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_246' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_246}}]]
2024-02-09 23:12:51.379660: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_248' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_248}}]]
2024-02-09 23:12:51.379694: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_249' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_249}}]]
2024-02-09 23:12:51.379728: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_252' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_252}}]]
2024-02-09 23:12:51.379761: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_253' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_253}}]]
2024-02-09 23:12:51.379794: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_254' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_254}}]]
2024-02-09 23:12:51.379828: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_255' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_255}}]]
2024-02-09 23:12:51.379862: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_256' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_256}}]]
2024-02-09 23:12:51.379903: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_257' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_257}}]]
2024-02-09 23:12:51.379960: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_258' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_258}}]]
2024-02-09 23:12:51.379997: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_261' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_261}}]]
2024-02-09 23:12:51.380032: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_262' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_262}}]]
2024-02-09 23:12:51.380081: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_263' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_263}}]]
2024-02-09 23:12:51.380122: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_264' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_264}}]]
2024-02-09 23:12:51.380162: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_265' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_265}}]]
2024-02-09 23:12:51.380200: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_266' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_266}}]]
2024-02-09 23:12:51.380236: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_267' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_267}}]]
2024-02-09 23:12:51.380277: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_268' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_268}}]]
2024-02-09 23:12:51.380330: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_269' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_269}}]]
2024-02-09 23:12:51.380368: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_273' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_273}}]]
2024-02-09 23:12:51.380404: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_274' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_274}}]]
2024-02-09 23:12:51.380441: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_275' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_275}}]]
2024-02-09 23:12:51.380478: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_276' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_276}}]]
2024-02-09 23:12:51.380515: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_277' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_277}}]]
2024-02-09 23:12:51.380550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_278' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_278}}]]
2024-02-09 23:12:51.380587: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_279' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_279}}]]
2024-02-09 23:12:51.380624: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_280' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_280}}]]
2024-02-09 23:12:51.380660: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_283' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_283}}]]
2024-02-09 23:12:51.380696: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_284' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_284}}]]
2024-02-09 23:12:51.380733: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_285' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_285}}]]
2024-02-09 23:12:51.380769: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_286' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_286}}]]
2024-02-09 23:12:51.380805: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_287' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_287}}]]
2024-02-09 23:12:51.380841: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_288' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_288}}]]
2024-02-09 23:12:51.380877: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_289' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_289}}]]
2024-02-09 23:12:51.380913: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_290' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_290}}]]
2024-02-09 23:12:51.380950: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_291' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_291}}]]
2024-02-09 23:12:51.380986: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_295' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_295}}]]
2024-02-09 23:12:51.381022: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_296' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_296}}]]
2024-02-09 23:12:51.381058: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_297' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_297}}]]
2024-02-09 23:12:51.381093: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_298' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_298}}]]
2024-02-09 23:12:51.381129: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_299' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_299}}]]
2024-02-09 23:12:51.381165: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_300' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_300}}]]
2024-02-09 23:12:51.381204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_301' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_301}}]]
2024-02-09 23:12:51.381241: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_302' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_302}}]]
2024-02-09 23:12:51.381277: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_305' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_305}}]]
2024-02-09 23:12:51.381312: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_306' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_306}}]]
2024-02-09 23:12:51.381348: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_307' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_307}}]]
2024-02-09 23:12:51.381385: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_308' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_308}}]]
2024-02-09 23:12:51.381422: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_309' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_309}}]]
2024-02-09 23:12:51.381463: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_310' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_310}}]]
2024-02-09 23:12:51.381501: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_311' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_311}}]]
2024-02-09 23:12:51.381537: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_312' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_312}}]]
2024-02-09 23:12:51.381573: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_314' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_314}}]]
2024-02-09 23:12:51.381609: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_315' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_315}}]]
2024-02-09 23:12:51.381645: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_318' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_318}}]]
2024-02-09 23:12:51.381682: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_319' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_319}}]]
2024-02-09 23:12:51.381719: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_320' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_320}}]]
2024-02-09 23:12:51.381755: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_321' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_321}}]]
2024-02-09 23:12:51.381791: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_322' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_322}}]]
2024-02-09 23:12:51.381827: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_323' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_323}}]]
2024-02-09 23:12:51.381863: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_324' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_324}}]]
2024-02-09 23:12:51.381917: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_327' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_327}}]]
2024-02-09 23:12:51.381954: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_328' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_328}}]]
2024-02-09 23:12:51.381990: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_329' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_329}}]]
2024-02-09 23:12:51.382027: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_330' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_330}}]]
2024-02-09 23:12:51.382062: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_331' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_331}}]]
2024-02-09 23:12:51.382099: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_332' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_332}}]]
2024-02-09 23:12:51.382135: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_333' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_333}}]]
2024-02-09 23:12:51.382171: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_334' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_334}}]]
2024-02-09 23:12:51.382206: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_335' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_335}}]]
2024-02-09 23:12:51.382241: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_339' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_339}}]]
2024-02-09 23:12:51.382277: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_340' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_340}}]]
2024-02-09 23:12:51.382312: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_341' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_341}}]]
2024-02-09 23:12:51.382347: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_342' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_342}}]]
2024-02-09 23:12:51.382382: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_343' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_343}}]]
2024-02-09 23:12:51.382418: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_344' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_344}}]]
2024-02-09 23:12:51.382453: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_345' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_345}}]]
2024-02-09 23:12:51.382488: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_346' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_346}}]]
2024-02-09 23:12:51.382524: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_349' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_349}}]]
2024-02-09 23:12:51.382559: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_350' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_350}}]]
2024-02-09 23:12:51.382594: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_351' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_351}}]]
2024-02-09 23:12:51.382640: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_352' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_352}}]]
2024-02-09 23:12:51.382674: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_353' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_353}}]]
2024-02-09 23:12:51.382709: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_354' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_354}}]]
2024-02-09 23:12:51.382744: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_355' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_355}}]]
2024-02-09 23:12:51.382778: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_356' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_356}}]]
2024-02-09 23:12:51.382812: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_357' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_357}}]]
2024-02-09 23:12:51.382846: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_361' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_361}}]]
2024-02-09 23:12:51.382904: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_362' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_362}}]]
2024-02-09 23:12:51.382945: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_363' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_363}}]]
2024-02-09 23:12:51.382986: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_364' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_364}}]]
2024-02-09 23:12:51.383023: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_365' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_365}}]]
2024-02-09 23:12:51.383058: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_366' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_366}}]]
2024-02-09 23:12:51.383094: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_367' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_367}}]]
2024-02-09 23:12:51.383129: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_368' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_368}}]]
2024-02-09 23:12:51.383165: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_371' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_371}}]]
2024-02-09 23:12:51.383200: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_372' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_372}}]]
2024-02-09 23:12:51.383235: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_373' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_373}}]]
2024-02-09 23:12:51.383270: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_374' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_374}}]]
2024-02-09 23:12:51.383307: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_375' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_375}}]]
2024-02-09 23:12:51.383342: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_376' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_376}}]]
2024-02-09 23:12:51.383378: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_377' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_377}}]]
2024-02-09 23:12:51.383413: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_378' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_378}}]]
2024-02-09 23:12:51.383448: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_380' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_380}}]]
2024-02-09 23:12:51.383484: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_381' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_381}}]]
2024-02-09 23:12:51.383519: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_384' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_384}}]]
2024-02-09 23:12:51.383554: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_385' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_385}}]]
2024-02-09 23:12:51.383591: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_386' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_386}}]]
2024-02-09 23:12:51.383633: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_387' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_387}}]]
2024-02-09 23:12:51.383669: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_388' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_388}}]]
2024-02-09 23:12:51.383705: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_389' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_389}}]]
2024-02-09 23:12:51.383742: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_390' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_390}}]]
2024-02-09 23:12:51.383777: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_393' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_393}}]]
2024-02-09 23:12:51.383812: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_394' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_394}}]]
2024-02-09 23:12:51.383847: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_395' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_395}}]]
2024-02-09 23:12:51.383883: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_396' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_396}}]]
2024-02-09 23:12:51.383918: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_397' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_397}}]]
2024-02-09 23:12:51.383954: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_398' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_398}}]]
2024-02-09 23:12:51.384006: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_399' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_399}}]]
2024-02-09 23:12:51.384043: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_400' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_400}}]]
2024-02-09 23:12:51.384079: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_401' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_401}}]]
2024-02-09 23:12:51.384116: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_405' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_405}}]]
2024-02-09 23:12:51.384152: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_406' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_406}}]]
2024-02-09 23:12:51.384188: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_407' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_407}}]]
2024-02-09 23:12:51.384224: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_408' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_408}}]]
2024-02-09 23:12:51.384259: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_409' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_409}}]]
2024-02-09 23:12:51.384299: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_410' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_410}}]]
2024-02-09 23:12:51.384336: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_411' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_411}}]]
2024-02-09 23:12:51.384372: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_412' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_412}}]]
2024-02-09 23:12:51.384408: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_415' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_415}}]]
2024-02-09 23:12:51.384444: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_416' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_416}}]]
2024-02-09 23:12:51.384481: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_417' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_417}}]]
2024-02-09 23:12:51.384516: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_418' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_418}}]]
2024-02-09 23:12:51.384552: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_419' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_419}}]]
2024-02-09 23:12:51.384588: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_420' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_420}}]]
2024-02-09 23:12:51.384624: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_421' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_421}}]]
2024-02-09 23:12:51.384661: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_422' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_422}}]]
2024-02-09 23:12:51.384696: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_423' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_423}}]]
2024-02-09 23:12:51.384732: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_427' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_427}}]]
2024-02-09 23:12:51.384768: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_428' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_428}}]]
2024-02-09 23:12:51.384805: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_429' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_429}}]]
2024-02-09 23:12:51.384843: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_430' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_430}}]]
2024-02-09 23:12:51.384884: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_431' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_431}}]]
2024-02-09 23:12:51.384921: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_432' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_432}}]]
2024-02-09 23:12:51.384958: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_433' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_433}}]]
2024-02-09 23:12:51.384994: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_434' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_434}}]]
2024-02-09 23:12:51.385031: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_437' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_437}}]]
2024-02-09 23:12:51.385067: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_438' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_438}}]]
2024-02-09 23:12:51.385104: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_439' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_439}}]]
2024-02-09 23:12:51.385150: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_440' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_440}}]]
2024-02-09 23:12:51.385186: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_441' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_441}}]]
2024-02-09 23:12:51.385221: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_442' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_442}}]]
2024-02-09 23:12:51.385257: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_443' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_443}}]]
2024-02-09 23:12:51.385292: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_444' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_444}}]]
2024-02-09 23:12:51.385328: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_446' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_446}}]]
2024-02-09 23:12:51.385364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_447' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_447}}]]
2024-02-09 23:12:51.385399: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_450' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_450}}]]
2024-02-09 23:12:51.385450: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_451' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_451}}]]
2024-02-09 23:12:51.385503: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_452' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_452}}]]
2024-02-09 23:12:51.385539: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_453' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_453}}]]
2024-02-09 23:12:51.385575: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_454' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_454}}]]
2024-02-09 23:12:51.385610: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_455' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_455}}]]
2024-02-09 23:12:51.385646: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_456' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_456}}]]
2024-02-09 23:12:51.385682: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_459' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_459}}]]
2024-02-09 23:12:51.385718: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_460' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_460}}]]
2024-02-09 23:12:51.385753: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_461' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_461}}]]
2024-02-09 23:12:51.385790: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_462' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_462}}]]
2024-02-09 23:12:51.385825: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_463' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_463}}]]
2024-02-09 23:12:51.385868: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_464' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_464}}]]
2024-02-09 23:12:51.385910: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_465' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_465}}]]
2024-02-09 23:12:51.385948: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_466' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_466}}]]
2024-02-09 23:12:51.385987: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_467' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_467}}]]
2024-02-09 23:12:51.386023: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_471' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_471}}]]
2024-02-09 23:12:51.386059: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_472' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_472}}]]
2024-02-09 23:12:51.386095: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_473' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_473}}]]
2024-02-09 23:12:51.386132: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_474' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_474}}]]
2024-02-09 23:12:51.386167: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_475' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_475}}]]
2024-02-09 23:12:51.386203: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_476' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_476}}]]
2024-02-09 23:12:51.386239: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_477' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_477}}]]
2024-02-09 23:12:51.386275: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_478' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_478}}]]
2024-02-09 23:12:51.386311: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_481' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_481}}]]
2024-02-09 23:12:51.386347: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_482' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_482}}]]
2024-02-09 23:12:51.386383: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_483' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_483}}]]
2024-02-09 23:12:51.386419: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_484' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_484}}]]
2024-02-09 23:12:51.386455: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_485' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_485}}]]
2024-02-09 23:12:51.386490: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_486' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_486}}]]
2024-02-09 23:12:51.386526: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_487' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_487}}]]
2024-02-09 23:12:51.386562: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_488' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_488}}]]
2024-02-09 23:12:51.386597: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_489' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_489}}]]
2024-02-09 23:12:51.386633: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_493' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_493}}]]
2024-02-09 23:12:51.386669: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_494' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_494}}]]
2024-02-09 23:12:51.386704: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_495' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_495}}]]
2024-02-09 23:12:51.386741: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_496' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_496}}]]
2024-02-09 23:12:51.386777: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_497' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_497}}]]
2024-02-09 23:12:51.386813: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_498' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_498}}]]
2024-02-09 23:12:51.386850: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_499' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_499}}]]
2024-02-09 23:12:51.386886: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_500' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_500}}]]
2024-02-09 23:12:51.386928: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_503' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_503}}]]
2024-02-09 23:12:51.386966: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_504' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_504}}]]
2024-02-09 23:12:51.387002: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_505' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_505}}]]
2024-02-09 23:12:51.387039: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_506' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_506}}]]
2024-02-09 23:12:51.387075: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_507' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_507}}]]
2024-02-09 23:12:51.387111: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_508' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_508}}]]
2024-02-09 23:12:51.387147: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_509' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_509}}]]
2024-02-09 23:12:51.387184: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_510' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_510}}]]
2024-02-09 23:12:51.387220: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_512' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_512}}]]
2024-02-09 23:12:51.387256: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_513' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_513}}]]
2024-02-09 23:12:51.387308: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_516' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_516}}]]
2024-02-09 23:12:51.387344: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_517' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_517}}]]
2024-02-09 23:12:51.387380: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_518' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_518}}]]
2024-02-09 23:12:51.387416: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_519' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_519}}]]
2024-02-09 23:12:51.387453: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_520' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_520}}]]
2024-02-09 23:12:51.387490: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_521' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_521}}]]
2024-02-09 23:12:51.387528: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_522' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_522}}]]
2024-02-09 23:12:51.387565: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_525' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_525}}]]
2024-02-09 23:12:51.387603: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_526' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_526}}]]
2024-02-09 23:12:51.387640: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_527' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_527}}]]
2024-02-09 23:12:51.387677: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_528' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_528}}]]
2024-02-09 23:12:51.387714: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_529' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_529}}]]
2024-02-09 23:12:51.387753: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_530' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_530}}]]
2024-02-09 23:12:51.387790: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_531' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_531}}]]
2024-02-09 23:12:51.387827: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_532' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_532}}]]
2024-02-09 23:12:51.387864: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_533' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_533}}]]
2024-02-09 23:12:51.387902: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_537' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_537}}]]
2024-02-09 23:12:51.387939: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_538' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_538}}]]
2024-02-09 23:12:51.387980: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_539' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_539}}]]
2024-02-09 23:12:51.388018: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_540' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_540}}]]
2024-02-09 23:12:51.388055: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_541' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_541}}]]
2024-02-09 23:12:51.388092: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_542' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_542}}]]
2024-02-09 23:12:51.388129: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_543' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_543}}]]
2024-02-09 23:12:51.388167: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_544' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_544}}]]
2024-02-09 23:12:51.388212: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_547' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_547}}]]
2024-02-09 23:12:51.388250: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_548' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_548}}]]
2024-02-09 23:12:51.388287: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_549' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_549}}]]
2024-02-09 23:12:51.388324: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_550' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_550}}]]
2024-02-09 23:12:51.388364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_551' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_551}}]]
2024-02-09 23:12:51.388402: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_552' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_552}}]]
2024-02-09 23:12:51.388449: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_553' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_553}}]]
2024-02-09 23:12:51.388485: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_554' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_554}}]]
2024-02-09 23:12:51.388522: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_555' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_555}}]]
2024-02-09 23:12:51.388558: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_557' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_557}}]]
2024-02-09 23:12:51.388594: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_563' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_563}}]]
2024-02-09 23:12:51.388638: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_564' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_564}}]]
2024-02-09 23:12:51.388679: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_565' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_565}}]]
2024-02-09 23:12:51.388715: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_566' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_566}}]]
2024-02-09 23:12:51.388751: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_567' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_567}}]]
2024-02-09 23:12:51.388788: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_568' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_568}}]]
2024-02-09 23:12:51.388824: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_569' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_569}}]]
2024-02-09 23:12:51.388860: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_570' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_570}}]]
2024-02-09 23:12:51.388897: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_575' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_575}}]]
2024-02-09 23:12:51.388932: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_576' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_576}}]]
2024-02-09 23:12:51.388968: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_577' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_577}}]]
2024-02-09 23:12:51.389008: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_578' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_578}}]]
2024-02-09 23:12:51.389045: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_579' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_579}}]]
2024-02-09 23:12:51.389082: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_580' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_580}}]]
2024-02-09 23:12:51.389119: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_581' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_581}}]]
2024-02-09 23:12:51.389154: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_582' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_582}}]]
2024-02-09 23:12:51.389190: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_584' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_584}}]]
2024-02-09 23:12:51.389227: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_585' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_585}}]]
2024-02-09 23:12:51.389271: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_588' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_588}}]]
2024-02-09 23:12:51.389309: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_589' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_589}}]]
2024-02-09 23:12:51.389345: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_590' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_590}}]]
2024-02-09 23:12:51.389381: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_591' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_591}}]]
2024-02-09 23:12:51.389418: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_592' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_592}}]]
2024-02-09 23:12:51.389459: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_593' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_593}}]]
2024-02-09 23:12:51.389513: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_594' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_594}}]]
2024-02-09 23:12:51.389550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_597' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_597}}]]
2024-02-09 23:12:51.389586: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_598' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_598}}]]
2024-02-09 23:12:51.389627: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_599' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_599}}]]
2024-02-09 23:12:51.389665: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_600' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_600}}]]
2024-02-09 23:12:51.389701: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_601' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_601}}]]
2024-02-09 23:12:51.389739: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_602' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_602}}]]
2024-02-09 23:12:51.389777: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_603' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_603}}]]
2024-02-09 23:12:51.389814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_604' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_604}}]]
2024-02-09 23:12:51.389851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_608' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_608}}]]
2024-02-09 23:12:51.389889: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_609' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_609}}]]
2024-02-09 23:12:51.389926: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_610' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_610}}]]
2024-02-09 23:12:51.389963: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_611' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_611}}]]
2024-02-09 23:12:51.390001: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_612' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_612}}]]
2024-02-09 23:12:51.390038: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_613' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_613}}]]
2024-02-09 23:12:51.390075: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_614' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_614}}]]
2024-02-09 23:12:51.390112: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_615' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_615}}]]
2024-02-09 23:12:51.390149: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_618' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_618}}]]
2024-02-09 23:12:51.390186: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_619' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_619}}]]
2024-02-09 23:12:51.390223: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_620' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_620}}]]
2024-02-09 23:12:51.390260: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_621' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_621}}]]
2024-02-09 23:12:51.390297: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_622' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_622}}]]
2024-02-09 23:12:51.390333: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_623' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_623}}]]
2024-02-09 23:12:51.390370: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_624' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_624}}]]
2024-02-09 23:12:51.390407: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_625' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_625}}]]
2024-02-09 23:12:51.390443: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_626' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_626}}]]
2024-02-09 23:12:51.390480: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_627' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_627}}]]
2024-02-09 23:12:51.390516: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_631' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_631}}]]
2024-02-09 23:12:51.390553: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_632' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_632}}]]
2024-02-09 23:12:51.390590: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_633' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_633}}]]
2024-02-09 23:12:51.390636: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_634' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_634}}]]
2024-02-09 23:12:51.390672: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_635' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_635}}]]
2024-02-09 23:12:51.390708: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_636' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_636}}]]
2024-02-09 23:12:51.390743: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_637' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_637}}]]
2024-02-09 23:12:51.390778: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_638' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_638}}]]
2024-02-09 23:12:51.390814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_641' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_641}}]]
2024-02-09 23:12:51.390857: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_642' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_642}}]]
2024-02-09 23:12:51.390900: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_643' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_643}}]]
2024-02-09 23:12:51.390938: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_644' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_644}}]]
2024-02-09 23:12:51.390974: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_645' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_645}}]]
2024-02-09 23:12:51.391010: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_646' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_646}}]]
2024-02-09 23:12:51.391047: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_647' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_647}}]]
2024-02-09 23:12:51.391083: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_648' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_648}}]]
2024-02-09 23:12:51.391123: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_650' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_650}}]]
2024-02-09 23:12:51.391160: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_651' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_651}}]]
2024-02-09 23:12:51.391197: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_654' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_654}}]]
2024-02-09 23:12:51.391232: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_655' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_655}}]]
2024-02-09 23:12:51.391268: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_656' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_656}}]]
2024-02-09 23:12:51.391303: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_657' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_657}}]]
2024-02-09 23:12:51.391340: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_658' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_658}}]]
2024-02-09 23:12:51.391375: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_659' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_659}}]]
2024-02-09 23:12:51.391411: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_660' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_660}}]]
2024-02-09 23:12:51.391447: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_663' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_663}}]]
2024-02-09 23:12:51.391483: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_664' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_664}}]]
2024-02-09 23:12:51.391522: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_665' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_665}}]]
2024-02-09 23:12:51.391559: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_666' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_666}}]]
2024-02-09 23:12:51.391595: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_667' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_667}}]]
2024-02-09 23:12:51.391634: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_668' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_668}}]]
2024-02-09 23:12:51.391671: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_669' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_669}}]]
2024-02-09 23:12:51.391706: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_670' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_670}}]]
2024-02-09 23:12:51.391743: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_671' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_671}}]]
2024-02-09 23:12:51.391779: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_675' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_675}}]]
2024-02-09 23:12:51.391814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_676' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_676}}]]
2024-02-09 23:12:51.391850: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_677' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_677}}]]
2024-02-09 23:12:51.391889: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_678' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_678}}]]
2024-02-09 23:12:51.391926: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_679' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_679}}]]
2024-02-09 23:12:51.391961: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_680' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_680}}]]
2024-02-09 23:12:51.391997: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_681' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_681}}]]
2024-02-09 23:12:51.392034: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_682' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_682}}]]
2024-02-09 23:12:51.392069: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_685' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_685}}]]
2024-02-09 23:12:51.392106: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_686' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_686}}]]
2024-02-09 23:12:51.392141: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_687' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_687}}]]
2024-02-09 23:12:51.392177: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_688' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_688}}]]
2024-02-09 23:12:51.392214: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_689' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_689}}]]
2024-02-09 23:12:51.392250: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_690' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_690}}]]
2024-02-09 23:12:51.392288: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_691' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_691}}]]
2024-02-09 23:12:51.392324: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_692' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_692}}]]
2024-02-09 23:12:51.392360: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_693' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_693}}]]
2024-02-09 23:12:51.392396: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_697' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_697}}]]
2024-02-09 23:12:51.392432: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_698' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_698}}]]
2024-02-09 23:12:51.392468: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_699' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_699}}]]
2024-02-09 23:12:51.392503: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_700' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_700}}]]
2024-02-09 23:12:51.392539: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_701' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_701}}]]
2024-02-09 23:12:51.392575: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_702' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_702}}]]
2024-02-09 23:12:51.392612: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_703' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_703}}]]
2024-02-09 23:12:51.392647: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_704' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_704}}]]
2024-02-09 23:12:51.392683: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_707' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_707}}]]
2024-02-09 23:12:51.392718: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_708' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_708}}]]
2024-02-09 23:12:51.392761: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_709' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_709}}]]
2024-02-09 23:12:51.392798: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_710' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_710}}]]
2024-02-09 23:12:51.392835: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_711' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_711}}]]
2024-02-09 23:12:51.392871: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_712' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_712}}]]
2024-02-09 23:12:51.392907: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_713' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_713}}]]
2024-02-09 23:12:51.392943: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_714' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_714}}]]
2024-02-09 23:12:51.392978: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_716' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_716}}]]
2024-02-09 23:12:51.393014: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_717' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_717}}]]
2024-02-09 23:12:51.393051: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_720' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_720}}]]
2024-02-09 23:12:51.393087: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_721' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_721}}]]
2024-02-09 23:12:51.393123: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_722' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_722}}]]
2024-02-09 23:12:51.393160: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_723' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_723}}]]
2024-02-09 23:12:51.393196: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_724' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_724}}]]
2024-02-09 23:12:51.393232: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_725' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_725}}]]
2024-02-09 23:12:51.393268: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_726' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_726}}]]
2024-02-09 23:12:51.393305: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_729' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_729}}]]
2024-02-09 23:12:51.393341: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_730' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_730}}]]
2024-02-09 23:12:51.393378: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_731' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_731}}]]
2024-02-09 23:12:51.393414: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_732' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_732}}]]
2024-02-09 23:12:51.393456: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_733' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_733}}]]
2024-02-09 23:12:51.393494: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_734' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_734}}]]
2024-02-09 23:12:51.393529: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_735' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_735}}]]
2024-02-09 23:12:51.393565: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_736' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_736}}]]
2024-02-09 23:12:51.393601: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_737' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_737}}]]
2024-02-09 23:12:51.393637: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_741' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_741}}]]
2024-02-09 23:12:51.393673: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_742' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_742}}]]
2024-02-09 23:12:51.393710: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_743' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_743}}]]
2024-02-09 23:12:51.393746: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_744' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_744}}]]
2024-02-09 23:12:51.393783: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_745' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_745}}]]
2024-02-09 23:12:51.393819: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_746' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_746}}]]
2024-02-09 23:12:51.393855: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_747' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_747}}]]
2024-02-09 23:12:51.393891: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_748' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_748}}]]
2024-02-09 23:12:51.393928: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_751' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_751}}]]
2024-02-09 23:12:51.393964: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_752' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_752}}]]
2024-02-09 23:12:51.394000: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_753' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_753}}]]
2024-02-09 23:12:51.394037: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_754' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_754}}]]
2024-02-09 23:12:51.394073: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_755' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_755}}]]
2024-02-09 23:12:51.394110: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_756' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_756}}]]
2024-02-09 23:12:51.394146: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_757' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_757}}]]
2024-02-09 23:12:51.394182: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_758' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_758}}]]
2024-02-09 23:12:51.394217: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_759' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_759}}]]
2024-02-09 23:12:51.394253: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_763' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_763}}]]
2024-02-09 23:12:51.394289: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_764' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_764}}]]
2024-02-09 23:12:51.394325: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_765' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_765}}]]
2024-02-09 23:12:51.394362: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_766' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_766}}]]
2024-02-09 23:12:51.394398: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_767' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_767}}]]
2024-02-09 23:12:51.394434: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_768' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_768}}]]
2024-02-09 23:12:51.394471: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_769' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_769}}]]
2024-02-09 23:12:51.394508: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_770' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_770}}]]
2024-02-09 23:12:51.394545: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_773' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_773}}]]
2024-02-09 23:12:51.394582: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_774' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_774}}]]
2024-02-09 23:12:51.394618: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_775' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_775}}]]
2024-02-09 23:12:51.394655: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_776' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_776}}]]
2024-02-09 23:12:51.394692: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_777' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_777}}]]
2024-02-09 23:12:51.394729: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_778' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_778}}]]
2024-02-09 23:12:51.394765: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_779' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_779}}]]
2024-02-09 23:12:51.394802: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_780' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_780}}]]
2024-02-09 23:12:51.394838: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_782' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_782}}]]
2024-02-09 23:12:51.394874: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_783' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_783}}]]
2024-02-09 23:12:51.394911: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_786' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_786}}]]
2024-02-09 23:12:51.394948: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_787' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_787}}]]
2024-02-09 23:12:51.394984: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_788' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_788}}]]
2024-02-09 23:12:51.395021: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_789' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_789}}]]
2024-02-09 23:12:51.395057: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_790' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_790}}]]
2024-02-09 23:12:51.395094: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_791' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_791}}]]
2024-02-09 23:12:51.395131: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_792' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_792}}]]
2024-02-09 23:12:51.395168: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_795' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_795}}]]
2024-02-09 23:12:51.395204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_796' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_796}}]]
2024-02-09 23:12:51.395241: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_797' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_797}}]]
2024-02-09 23:12:51.395277: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_798' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_798}}]]
2024-02-09 23:12:51.395314: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_799' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_799}}]]
2024-02-09 23:12:51.395351: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_800' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_800}}]]
2024-02-09 23:12:51.395388: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_801' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_801}}]]
2024-02-09 23:12:51.395424: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_802' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_802}}]]
2024-02-09 23:12:51.395461: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_803' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_803}}]]
2024-02-09 23:12:51.395498: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_807' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_807}}]]
2024-02-09 23:12:51.395534: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_808' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_808}}]]
2024-02-09 23:12:51.395571: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_809' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_809}}]]
2024-02-09 23:12:51.395607: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_810' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_810}}]]
2024-02-09 23:12:51.395644: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_811' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_811}}]]
2024-02-09 23:12:51.395680: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_812' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_812}}]]
2024-02-09 23:12:51.395715: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_813' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_813}}]]
2024-02-09 23:12:51.395752: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_814' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_814}}]]
2024-02-09 23:12:51.395788: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_817' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_817}}]]
2024-02-09 23:12:51.395824: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_818' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_818}}]]
2024-02-09 23:12:51.395861: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_819' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_819}}]]
2024-02-09 23:12:51.395897: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_820' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_820}}]]
2024-02-09 23:12:51.395933: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_821' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_821}}]]
2024-02-09 23:12:51.395969: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_822' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_822}}]]
2024-02-09 23:12:51.396006: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_823' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_823}}]]
2024-02-09 23:12:51.396042: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_824' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_824}}]]
2024-02-09 23:12:51.396078: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_825' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_825}}]]
2024-02-09 23:12:51.396115: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_827' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_827}}]]
2024-02-09 23:12:51.396151: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_833' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_833}}]]
2024-02-09 23:12:51.396188: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_834' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_834}}]]
2024-02-09 23:12:51.396224: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_835' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_835}}]]
2024-02-09 23:12:51.396260: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_836' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_836}}]]
2024-02-09 23:12:51.396296: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_837' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_837}}]]
2024-02-09 23:12:51.396332: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_838' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_838}}]]
2024-02-09 23:12:51.396368: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_839' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_839}}]]
2024-02-09 23:12:51.396405: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_840' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_840}}]]
2024-02-09 23:12:51.396441: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_845' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_845}}]]
2024-02-09 23:12:51.396478: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_846' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_846}}]]
2024-02-09 23:12:51.396514: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_847' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_847}}]]
2024-02-09 23:12:51.396550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_848' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_848}}]]
2024-02-09 23:12:51.396587: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_849' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_849}}]]
2024-02-09 23:12:51.396624: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_850' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_850}}]]
2024-02-09 23:12:51.396660: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_851' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_851}}]]
2024-02-09 23:12:51.396696: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_852' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_852}}]]
2024-02-09 23:12:51.396732: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_854' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_854}}]]
2024-02-09 23:12:51.396769: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_855' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_855}}]]
2024-02-09 23:12:51.396806: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_858' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_858}}]]
2024-02-09 23:12:51.396843: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_859' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_859}}]]
2024-02-09 23:12:51.396879: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_860' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_860}}]]
2024-02-09 23:12:51.396915: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_861' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_861}}]]
2024-02-09 23:12:51.396951: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_862' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_862}}]]
2024-02-09 23:12:51.396988: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_863' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_863}}]]
2024-02-09 23:12:51.397024: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_864' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_864}}]]
2024-02-09 23:12:51.397061: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_867' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_867}}]]
2024-02-09 23:12:51.397096: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_868' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_868}}]]
2024-02-09 23:12:51.397132: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_869' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_869}}]]
2024-02-09 23:12:51.397168: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_870' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_870}}]]
2024-02-09 23:12:51.397204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_871' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_871}}]]
2024-02-09 23:12:51.397240: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_872' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_872}}]]
2024-02-09 23:12:51.397276: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_873' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_873}}]]
2024-02-09 23:12:51.397312: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_874' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_874}}]]
2024-02-09 23:12:51.397349: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_878' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_878}}]]
2024-02-09 23:12:51.397385: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_879' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_879}}]]
2024-02-09 23:12:51.397422: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_880' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_880}}]]
2024-02-09 23:12:51.397481: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_881' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_881}}]]
2024-02-09 23:12:51.397520: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_882' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_882}}]]
2024-02-09 23:12:51.397557: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_883' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_883}}]]
2024-02-09 23:12:51.397593: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_884' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_884}}]]
2024-02-09 23:12:51.397631: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_885' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_885}}]]
2024-02-09 23:12:51.397669: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_888' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_888}}]]
2024-02-09 23:12:51.397707: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_889' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_889}}]]
2024-02-09 23:12:51.397744: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_890' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_890}}]]
2024-02-09 23:12:51.397780: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_891' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_891}}]]
2024-02-09 23:12:51.397817: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_892' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_892}}]]
2024-02-09 23:12:51.397855: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_893' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_893}}]]
2024-02-09 23:12:51.397892: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_894' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_894}}]]
2024-02-09 23:12:51.397929: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_895' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_895}}]]
2024-02-09 23:12:51.397967: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_896' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_896}}]]
2024-02-09 23:12:51.398004: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_897' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_897}}]]
2024-02-09 23:12:51.398040: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_901' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_901}}]]
2024-02-09 23:12:51.398077: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_902' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_902}}]]
2024-02-09 23:12:51.398115: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_903' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_903}}]]
2024-02-09 23:12:51.398152: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_904' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_904}}]]
2024-02-09 23:12:51.398190: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_905' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_905}}]]
2024-02-09 23:12:51.398226: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_906' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_906}}]]
2024-02-09 23:12:51.398263: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_907' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_907}}]]
2024-02-09 23:12:51.398300: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_908' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_908}}]]
2024-02-09 23:12:51.398337: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_911' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_911}}]]
2024-02-09 23:12:51.398373: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_912' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_912}}]]
2024-02-09 23:12:51.398410: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_913' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_913}}]]
2024-02-09 23:12:51.398447: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_914' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_914}}]]
2024-02-09 23:12:51.398484: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_915' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_915}}]]
2024-02-09 23:12:51.398521: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_916' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_916}}]]
2024-02-09 23:12:51.398558: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_917' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_917}}]]
2024-02-09 23:12:51.398595: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_918' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_918}}]]
2024-02-09 23:12:51.398643: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_920' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_920}}]]
2024-02-09 23:12:51.398680: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_921' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_921}}]]
2024-02-09 23:12:51.398726: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_924' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_924}}]]
2024-02-09 23:12:51.398761: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_925' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_925}}]]
2024-02-09 23:12:51.398796: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_926' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_926}}]]
2024-02-09 23:12:51.398831: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_927' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_927}}]]
2024-02-09 23:12:51.398867: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_928' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_928}}]]
2024-02-09 23:12:51.398902: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_929' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_929}}]]
2024-02-09 23:12:51.398937: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_930' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_930}}]]
2024-02-09 23:12:51.398971: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_933' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_933}}]]
2024-02-09 23:12:51.399005: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_934' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_934}}]]
2024-02-09 23:12:51.399040: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_935' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_935}}]]
2024-02-09 23:12:51.399075: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_936' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_936}}]]
2024-02-09 23:12:51.399110: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_937' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_937}}]]
2024-02-09 23:12:51.399145: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_938' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_938}}]]
2024-02-09 23:12:51.399179: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_939' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_939}}]]
2024-02-09 23:12:51.399213: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_940' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_940}}]]
2024-02-09 23:12:51.399248: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_941' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_941}}]]
2024-02-09 23:12:51.399284: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_945' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_945}}]]
2024-02-09 23:12:51.399318: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_946' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_946}}]]
2024-02-09 23:12:51.399353: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_947' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_947}}]]
2024-02-09 23:12:51.399387: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_948' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_948}}]]
2024-02-09 23:12:51.399422: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_949' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_949}}]]
2024-02-09 23:12:51.399457: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_950' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_950}}]]
2024-02-09 23:12:51.399492: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_951' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_951}}]]
2024-02-09 23:12:51.399527: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_952' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_952}}]]
2024-02-09 23:12:51.399561: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_955' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_955}}]]
2024-02-09 23:12:51.399595: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_956' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_956}}]]
2024-02-09 23:12:51.399630: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_957' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_957}}]]
2024-02-09 23:12:51.399664: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_958' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_958}}]]
2024-02-09 23:12:51.399699: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_959' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_959}}]]
2024-02-09 23:12:51.399734: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_960' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_960}}]]
2024-02-09 23:12:51.399769: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_961' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_961}}]]
2024-02-09 23:12:51.399804: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_962' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_962}}]]
2024-02-09 23:12:51.399839: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_963' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_963}}]]
2024-02-09 23:12:51.399874: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_967' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_967}}]]
2024-02-09 23:12:51.399909: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_968' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_968}}]]
2024-02-09 23:12:51.399944: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_969' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_969}}]]
2024-02-09 23:12:51.399978: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_970' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_970}}]]
2024-02-09 23:12:51.400013: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_971' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_971}}]]
2024-02-09 23:12:51.400047: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_972' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_972}}]]
2024-02-09 23:12:51.400081: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_973' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_973}}]]
2024-02-09 23:12:51.400116: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_974' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_974}}]]
2024-02-09 23:12:51.400150: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_977' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_977}}]]
2024-02-09 23:12:51.400185: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_978' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_978}}]]
2024-02-09 23:12:51.400220: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_979' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_979}}]]
2024-02-09 23:12:51.400254: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_980' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_980}}]]
2024-02-09 23:12:51.400289: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_981' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_981}}]]
2024-02-09 23:12:51.400323: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_982' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_982}}]]
2024-02-09 23:12:51.400359: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_983' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_983}}]]
2024-02-09 23:12:51.400393: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_984' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_984}}]]
2024-02-09 23:12:51.400428: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_986' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_986}}]]
2024-02-09 23:12:51.400463: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_987' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_987}}]]
2024-02-09 23:12:51.400498: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_990' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_990}}]]
2024-02-09 23:12:51.400533: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_991' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_991}}]]
2024-02-09 23:12:51.400569: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_992' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_992}}]]
2024-02-09 23:12:51.400603: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_993' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_993}}]]
2024-02-09 23:12:51.400638: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_994' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_994}}]]
2024-02-09 23:12:51.400672: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_995' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_995}}]]
2024-02-09 23:12:51.400707: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_996' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_996}}]]
2024-02-09 23:12:51.400742: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_999' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_999}}]]
2024-02-09 23:12:51.400777: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1000' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1000}}]]
2024-02-09 23:12:51.400811: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1001' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1001}}]]
2024-02-09 23:12:51.400846: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1002' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1002}}]]
2024-02-09 23:12:51.400881: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1003' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1003}}]]
2024-02-09 23:12:51.400916: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1004' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1004}}]]
2024-02-09 23:12:51.400950: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1005' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1005}}]]
2024-02-09 23:12:51.400985: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1006' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1006}}]]
2024-02-09 23:12:51.401020: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1007' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1007}}]]
2024-02-09 23:12:51.401054: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1011' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1011}}]]
2024-02-09 23:12:51.401090: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1012' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1012}}]]
2024-02-09 23:12:51.401125: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1013' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1013}}]]
2024-02-09 23:12:51.401159: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1014' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1014}}]]
2024-02-09 23:12:51.401194: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1015' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1015}}]]
2024-02-09 23:12:51.401228: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1016' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1016}}]]
2024-02-09 23:12:51.401263: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1017' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1017}}]]
2024-02-09 23:12:51.401298: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1018' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1018}}]]
2024-02-09 23:12:51.401333: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1021' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1021}}]]
2024-02-09 23:12:51.401367: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1022' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1022}}]]
2024-02-09 23:12:51.401402: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1023' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1023}}]]
2024-02-09 23:12:51.401442: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1024' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1024}}]]
2024-02-09 23:12:51.401495: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1025' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1025}}]]
2024-02-09 23:12:51.401531: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1026' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1026}}]]
2024-02-09 23:12:51.401568: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1027' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1027}}]]
2024-02-09 23:12:51.401604: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1028' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1028}}]]
2024-02-09 23:12:51.401641: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1029' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1029}}]]
2024-02-09 23:12:51.401678: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1031' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1031}}]]
2024-02-09 23:12:51.401714: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1037' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1037}}]]
2024-02-09 23:12:51.401751: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1038' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1038}}]]
2024-02-09 23:12:51.401787: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1039' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1039}}]]
2024-02-09 23:12:51.401825: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1040' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1040}}]]
2024-02-09 23:12:51.401861: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1041' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1041}}]]
2024-02-09 23:12:51.401898: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1042' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1042}}]]
2024-02-09 23:12:51.401935: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1043' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1043}}]]
2024-02-09 23:12:51.401974: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1044' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1044}}]]
2024-02-09 23:12:51.402011: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1049' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1049}}]]
2024-02-09 23:12:51.402047: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1050' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1050}}]]
2024-02-09 23:12:51.402084: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1051' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1051}}]]
2024-02-09 23:12:51.402122: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1052' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1052}}]]
2024-02-09 23:12:51.402159: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1053' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1053}}]]
2024-02-09 23:12:51.402196: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1054' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1054}}]]
2024-02-09 23:12:51.402233: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1055' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1055}}]]
2024-02-09 23:12:51.402270: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1056' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1056}}]]
2024-02-09 23:12:51.402307: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1058' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1058}}]]
2024-02-09 23:12:51.402345: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1059' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1059}}]]
2024-02-09 23:12:51.402381: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1062' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1062}}]]
2024-02-09 23:12:51.402418: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1063' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1063}}]]
2024-02-09 23:12:51.402454: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1064' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1064}}]]
2024-02-09 23:12:51.402490: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1065' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1065}}]]
2024-02-09 23:12:51.402527: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1066' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1066}}]]
2024-02-09 23:12:51.402564: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1067' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1067}}]]
2024-02-09 23:12:51.402601: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1068' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1068}}]]
2024-02-09 23:12:51.402637: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1071' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1071}}]]
2024-02-09 23:12:51.402673: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1072' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1072}}]]
2024-02-09 23:12:51.402719: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1073' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1073}}]]
2024-02-09 23:12:51.402755: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1074' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1074}}]]
2024-02-09 23:12:51.402790: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1075' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1075}}]]
2024-02-09 23:12:51.402826: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1076' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1076}}]]
2024-02-09 23:12:51.402861: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1077' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1077}}]]
2024-02-09 23:12:51.402897: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1078' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1078}}]]
2024-02-09 23:12:51.402933: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1082' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1082}}]]
2024-02-09 23:12:51.402969: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1083' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1083}}]]
2024-02-09 23:12:51.403004: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1084' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1084}}]]
2024-02-09 23:12:51.403039: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1085' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1085}}]]
2024-02-09 23:12:51.403074: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1086' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1086}}]]
2024-02-09 23:12:51.403109: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1087' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1087}}]]
2024-02-09 23:12:51.403145: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1088' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1088}}]]
2024-02-09 23:12:51.403180: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1089' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1089}}]]
2024-02-09 23:12:51.403216: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1092' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1092}}]]
2024-02-09 23:12:51.403251: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1093' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1093}}]]
2024-02-09 23:12:51.403287: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1094' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1094}}]]
2024-02-09 23:12:51.403322: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1095' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1095}}]]
2024-02-09 23:12:51.403357: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1096' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1096}}]]
2024-02-09 23:12:51.403392: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1097' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1097}}]]
2024-02-09 23:12:51.403428: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1098' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1098}}]]
2024-02-09 23:12:51.403462: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1101' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1101}}]]
2024-02-09 23:12:51.403497: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1102' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1102}}]]
2024-02-09 23:12:51.403533: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1103' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1103}}]]
2024-02-09 23:12:51.403569: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1105' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1105}}]]
2024-02-09 23:12:51.403605: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1106' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1106}}]]
2024-02-09 23:12:51.403642: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1111' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1111}}]]
2024-02-09 23:12:51.403678: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1112' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1112}}]]
2024-02-09 23:12:51.403713: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1113' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1113}}]]
2024-02-09 23:12:51.403749: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1114' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1114}}]]
2024-02-09 23:12:51.910039: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall' with dtype float and shape [?,?,?,?]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall}}]]
2024-02-09 23:12:51.910118: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1' with dtype int32 and shape [2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1}}]]
2024-02-09 23:12:51.910159: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_4' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_4}}]]
2024-02-09 23:12:51.910197: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_5' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_5}}]]
2024-02-09 23:12:51.910233: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_6' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_6}}]]
2024-02-09 23:12:51.910269: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_7' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_7}}]]
2024-02-09 23:12:51.910319: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_8' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_8}}]]
2024-02-09 23:12:51.910355: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_9' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_9}}]]
2024-02-09 23:12:51.910390: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_10' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_10}}]]
2024-02-09 23:12:51.910425: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_11' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_11}}]]
2024-02-09 23:12:51.910461: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_12' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_12}}]]
2024-02-09 23:12:51.910498: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_13' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_13}}]]
2024-02-09 23:12:51.910535: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_14' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_14}}]]
2024-02-09 23:12:51.910572: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_15' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_15}}]]
2024-02-09 23:12:51.910608: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_16' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_16}}]]
2024-02-09 23:12:51.910644: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_17' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_17}}]]
2024-02-09 23:12:51.910679: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_18' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_18}}]]
2024-02-09 23:12:51.910714: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_19' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_19}}]]
2024-02-09 23:12:51.910749: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_20' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_20}}]]
2024-02-09 23:12:51.910783: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_21' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_21}}]]
2024-02-09 23:12:51.910819: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_25' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_25}}]]
2024-02-09 23:12:51.910854: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_26' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_26}}]]
2024-02-09 23:12:51.910891: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_27' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_27}}]]
2024-02-09 23:12:51.910926: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_28' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_28}}]]
2024-02-09 23:12:51.910965: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_29' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_29}}]]
2024-02-09 23:12:51.911002: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_30' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_30}}]]
2024-02-09 23:12:51.911039: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_31' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_31}}]]
2024-02-09 23:12:51.911077: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_32' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_32}}]]
2024-02-09 23:12:51.911114: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_35' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_35}}]]
2024-02-09 23:12:51.911152: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_36' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_36}}]]
2024-02-09 23:12:51.911190: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_37' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_37}}]]
2024-02-09 23:12:51.911228: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_38' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_38}}]]
2024-02-09 23:12:51.911266: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_39' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_39}}]]
2024-02-09 23:12:51.911303: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_40' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_40}}]]
2024-02-09 23:12:51.911340: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_41' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_41}}]]
2024-02-09 23:12:51.911377: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_42' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_42}}]]
2024-02-09 23:12:51.911414: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_44' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_44}}]]
2024-02-09 23:12:51.911450: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_45' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_45}}]]
2024-02-09 23:12:51.911488: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_48' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_48}}]]
2024-02-09 23:12:51.911525: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_49' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_49}}]]
2024-02-09 23:12:51.911561: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_50' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_50}}]]
2024-02-09 23:12:51.911597: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_51' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_51}}]]
2024-02-09 23:12:51.911634: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_52' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_52}}]]
2024-02-09 23:12:51.911670: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_53' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_53}}]]
2024-02-09 23:12:51.911707: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_54' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_54}}]]
2024-02-09 23:12:51.911744: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_57' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_57}}]]
2024-02-09 23:12:51.911780: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_58' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_58}}]]
2024-02-09 23:12:51.911817: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_59' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_59}}]]
2024-02-09 23:12:51.911854: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_60' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_60}}]]
2024-02-09 23:12:51.911891: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_61' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_61}}]]
2024-02-09 23:12:51.911940: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_62' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_62}}]]
2024-02-09 23:12:51.911976: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_63' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_63}}]]
2024-02-09 23:12:51.912011: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_64' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_64}}]]
2024-02-09 23:12:51.912047: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_65' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_65}}]]
2024-02-09 23:12:51.912083: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_69' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_69}}]]
2024-02-09 23:12:51.912119: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_70' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_70}}]]
2024-02-09 23:12:51.912154: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_71' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_71}}]]
2024-02-09 23:12:51.912190: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_72' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_72}}]]
2024-02-09 23:12:51.912225: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_73' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_73}}]]
2024-02-09 23:12:51.912262: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_74' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_74}}]]
2024-02-09 23:12:51.912298: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_75' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_75}}]]
2024-02-09 23:12:51.912333: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_76' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_76}}]]
2024-02-09 23:12:51.912368: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_79' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_79}}]]
2024-02-09 23:12:51.912403: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_80' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_80}}]]
2024-02-09 23:12:51.912439: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_81' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_81}}]]
2024-02-09 23:12:51.912474: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_82' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_82}}]]
2024-02-09 23:12:51.912510: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_83' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_83}}]]
2024-02-09 23:12:51.912545: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_84' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_84}}]]
2024-02-09 23:12:51.912580: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_85' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_85}}]]
2024-02-09 23:12:51.912615: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_86' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_86}}]]
2024-02-09 23:12:51.912651: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_87' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_87}}]]
2024-02-09 23:12:51.912686: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_91' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_91}}]]
2024-02-09 23:12:51.912721: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_92' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_92}}]]
2024-02-09 23:12:51.912756: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_93' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_93}}]]
2024-02-09 23:12:51.912792: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_94' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_94}}]]
2024-02-09 23:12:51.912827: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_95' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_95}}]]
2024-02-09 23:12:51.912862: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_96' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_96}}]]
2024-02-09 23:12:51.912897: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_97' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_97}}]]
2024-02-09 23:12:51.912932: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_98' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_98}}]]
2024-02-09 23:12:51.912968: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_101' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_101}}]]
2024-02-09 23:12:51.913003: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_102' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_102}}]]
2024-02-09 23:12:51.913039: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_103' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_103}}]]
2024-02-09 23:12:51.913074: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_104' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_104}}]]
2024-02-09 23:12:51.913110: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_105' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_105}}]]
2024-02-09 23:12:51.913146: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_106' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_106}}]]
2024-02-09 23:12:51.913181: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_107' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_107}}]]
2024-02-09 23:12:51.913216: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_108' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_108}}]]
2024-02-09 23:12:51.913251: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_110' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_110}}]]
2024-02-09 23:12:51.913287: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_111' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_111}}]]
2024-02-09 23:12:51.913322: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_114' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_114}}]]
2024-02-09 23:12:51.913357: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_115' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_115}}]]
2024-02-09 23:12:51.913392: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_116' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_116}}]]
2024-02-09 23:12:51.913427: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_117' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_117}}]]
2024-02-09 23:12:51.913486: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_118' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_118}}]]
2024-02-09 23:12:51.913523: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_119' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_119}}]]
2024-02-09 23:12:51.913560: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_120' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_120}}]]
2024-02-09 23:12:51.913598: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_123' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_123}}]]
2024-02-09 23:12:51.913634: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_124' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_124}}]]
2024-02-09 23:12:51.913671: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_125' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_125}}]]
2024-02-09 23:12:51.913707: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_126' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_126}}]]
2024-02-09 23:12:51.913744: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_127' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_127}}]]
2024-02-09 23:12:51.913781: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_128' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_128}}]]
2024-02-09 23:12:51.913818: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_129' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_129}}]]
2024-02-09 23:12:51.913854: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_130' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_130}}]]
2024-02-09 23:12:51.913891: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_131' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_131}}]]
2024-02-09 23:12:51.913927: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_135' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_135}}]]
2024-02-09 23:12:51.913964: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_136' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_136}}]]
2024-02-09 23:12:51.914000: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_137' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_137}}]]
2024-02-09 23:12:51.914037: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_138' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_138}}]]
2024-02-09 23:12:51.914073: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_139' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_139}}]]
2024-02-09 23:12:51.914110: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_140' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_140}}]]
2024-02-09 23:12:51.914146: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_141' with dtype float and shape [?,1,1,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_141}}]]
2024-02-09 23:12:51.914183: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_142' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_142}}]]
2024-02-09 23:12:51.914220: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_145' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_145}}]]
2024-02-09 23:12:51.914257: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_146' with dtype float and shape [32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_146}}]]
2024-02-09 23:12:51.914294: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_147' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_147}}]]
2024-02-09 23:12:51.914330: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_148' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_148}}]]
2024-02-09 23:12:51.914368: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_149' with dtype float and shape [?,?,?,32,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_149}}]]
2024-02-09 23:12:51.914404: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_150' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_150}}]]
2024-02-09 23:12:51.914441: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_151' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_151}}]]
2024-02-09 23:12:51.914477: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_152' with dtype float and shape [?,?,?,2048]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_152}}]]
2024-02-09 23:12:51.914514: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_153' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_153}}]]
2024-02-09 23:12:51.914550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_155' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_155}}]]
2024-02-09 23:12:51.914586: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_161' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_161}}]]
2024-02-09 23:12:51.914623: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_162' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_162}}]]
2024-02-09 23:12:51.914660: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_163' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_163}}]]
2024-02-09 23:12:51.914696: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_164' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_164}}]]
2024-02-09 23:12:51.914744: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_165' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_165}}]]
2024-02-09 23:12:51.914779: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_166' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_166}}]]
2024-02-09 23:12:51.914814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_167' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_167}}]]
2024-02-09 23:12:51.914849: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_168' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_168}}]]
2024-02-09 23:12:51.914884: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_173' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_173}}]]
2024-02-09 23:12:51.914920: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_174' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_174}}]]
2024-02-09 23:12:51.914954: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_175' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_175}}]]
2024-02-09 23:12:51.914990: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_176' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_176}}]]
2024-02-09 23:12:51.915026: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_177' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_177}}]]
2024-02-09 23:12:51.915061: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_178' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_178}}]]
2024-02-09 23:12:51.915097: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_179' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_179}}]]
2024-02-09 23:12:51.915133: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_180' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_180}}]]
2024-02-09 23:12:51.915168: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_182' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_182}}]]
2024-02-09 23:12:51.915204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_183' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_183}}]]
2024-02-09 23:12:51.915239: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_186' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_186}}]]
2024-02-09 23:12:51.915274: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_187' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_187}}]]
2024-02-09 23:12:51.915310: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_188' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_188}}]]
2024-02-09 23:12:51.915345: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_189' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_189}}]]
2024-02-09 23:12:51.915381: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_190' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_190}}]]
2024-02-09 23:12:51.915433: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_191' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_191}}]]
2024-02-09 23:12:51.915470: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_192' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_192}}]]
2024-02-09 23:12:51.915511: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_195' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_195}}]]
2024-02-09 23:12:51.915547: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_196' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_196}}]]
2024-02-09 23:12:51.915583: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_197' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_197}}]]
2024-02-09 23:12:51.915620: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_198' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_198}}]]
2024-02-09 23:12:51.915657: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_199' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_199}}]]
2024-02-09 23:12:51.915694: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_200' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_200}}]]
2024-02-09 23:12:51.915731: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_201' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_201}}]]
2024-02-09 23:12:51.915767: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_202' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_202}}]]
2024-02-09 23:12:51.915804: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_206' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_206}}]]
2024-02-09 23:12:51.915840: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_207' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_207}}]]
2024-02-09 23:12:51.915878: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_208' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_208}}]]
2024-02-09 23:12:51.915914: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_209' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_209}}]]
2024-02-09 23:12:51.915950: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_210' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_210}}]]
2024-02-09 23:12:51.915987: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_211' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_211}}]]
2024-02-09 23:12:51.916024: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_212' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_212}}]]
2024-02-09 23:12:51.916061: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_213' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_213}}]]
2024-02-09 23:12:51.916098: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_216' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_216}}]]
2024-02-09 23:12:51.916135: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_217' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_217}}]]
2024-02-09 23:12:51.916171: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_218' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_218}}]]
2024-02-09 23:12:51.916208: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_219' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_219}}]]
2024-02-09 23:12:51.916246: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_220' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_220}}]]
2024-02-09 23:12:51.916282: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_221' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_221}}]]
2024-02-09 23:12:51.916319: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_222' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_222}}]]
2024-02-09 23:12:51.916355: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_223' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_223}}]]
2024-02-09 23:12:51.916393: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_224' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_224}}]]
2024-02-09 23:12:51.916429: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_225' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_225}}]]
2024-02-09 23:12:51.916466: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_229' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_229}}]]
2024-02-09 23:12:51.916503: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_230' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_230}}]]
2024-02-09 23:12:51.916540: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_231' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_231}}]]
2024-02-09 23:12:51.916576: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_232' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_232}}]]
2024-02-09 23:12:51.916623: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_233' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_233}}]]
2024-02-09 23:12:51.916659: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_234' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_234}}]]
2024-02-09 23:12:51.916695: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_235' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_235}}]]
2024-02-09 23:12:51.916730: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_236' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_236}}]]
2024-02-09 23:12:51.916766: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_239' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_239}}]]
2024-02-09 23:12:51.916801: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_240' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_240}}]]
2024-02-09 23:12:51.916838: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_241' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_241}}]]
2024-02-09 23:12:51.916873: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_242' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_242}}]]
2024-02-09 23:12:51.916909: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_243' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_243}}]]
2024-02-09 23:12:51.916944: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_244' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_244}}]]
2024-02-09 23:12:51.916979: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_245' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_245}}]]
2024-02-09 23:12:51.917015: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_246' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_246}}]]
2024-02-09 23:12:51.917051: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_248' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_248}}]]
2024-02-09 23:12:51.917088: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_249' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_249}}]]
2024-02-09 23:12:51.917123: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_252' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_252}}]]
2024-02-09 23:12:51.917159: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_253' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_253}}]]
2024-02-09 23:12:51.917195: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_254' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_254}}]]
2024-02-09 23:12:51.917231: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_255' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_255}}]]
2024-02-09 23:12:51.917266: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_256' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_256}}]]
2024-02-09 23:12:51.917302: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_257' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_257}}]]
2024-02-09 23:12:51.917338: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_258' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_258}}]]
2024-02-09 23:12:51.917374: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_261' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_261}}]]
2024-02-09 23:12:51.917410: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_262' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_262}}]]
2024-02-09 23:12:51.917450: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_263' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_263}}]]
2024-02-09 23:12:51.917505: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_264' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_264}}]]
2024-02-09 23:12:51.917542: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_265' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_265}}]]
2024-02-09 23:12:51.917580: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_266' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_266}}]]
2024-02-09 23:12:51.917617: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_267' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_267}}]]
2024-02-09 23:12:51.917655: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_268' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_268}}]]
2024-02-09 23:12:51.917693: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_269' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_269}}]]
2024-02-09 23:12:51.917731: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_273' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_273}}]]
2024-02-09 23:12:51.917768: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_274' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_274}}]]
2024-02-09 23:12:51.917805: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_275' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_275}}]]
2024-02-09 23:12:51.917843: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_276' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_276}}]]
2024-02-09 23:12:51.917880: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_277' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_277}}]]
2024-02-09 23:12:51.917916: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_278' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_278}}]]
2024-02-09 23:12:51.917953: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_279' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_279}}]]
2024-02-09 23:12:51.917991: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_280' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_280}}]]
2024-02-09 23:12:51.918028: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_283' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_283}}]]
2024-02-09 23:12:51.918064: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_284' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_284}}]]
2024-02-09 23:12:51.918102: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_285' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_285}}]]
2024-02-09 23:12:51.918139: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_286' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_286}}]]
2024-02-09 23:12:51.918176: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_287' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_287}}]]
2024-02-09 23:12:51.918213: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_288' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_288}}]]
2024-02-09 23:12:51.918250: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_289' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_289}}]]
2024-02-09 23:12:51.918287: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_290' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_290}}]]
2024-02-09 23:12:51.918324: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_291' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_291}}]]
2024-02-09 23:12:51.918360: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_295' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_295}}]]
2024-02-09 23:12:51.918397: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_296' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_296}}]]
2024-02-09 23:12:51.918434: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_297' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_297}}]]
2024-02-09 23:12:51.918472: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_298' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_298}}]]
2024-02-09 23:12:51.918509: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_299' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_299}}]]
2024-02-09 23:12:51.918547: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_300' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_300}}]]
2024-02-09 23:12:51.918585: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_301' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_301}}]]
2024-02-09 23:12:51.918622: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_302' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_302}}]]
2024-02-09 23:12:51.918659: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_305' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_305}}]]
2024-02-09 23:12:51.918706: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_306' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_306}}]]
2024-02-09 23:12:51.918741: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_307' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_307}}]]
2024-02-09 23:12:51.918778: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_308' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_308}}]]
2024-02-09 23:12:51.918814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_309' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_309}}]]
2024-02-09 23:12:51.918850: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_310' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_310}}]]
2024-02-09 23:12:51.918885: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_311' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_311}}]]
2024-02-09 23:12:51.918920: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_312' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_312}}]]
2024-02-09 23:12:51.918956: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_314' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_314}}]]
2024-02-09 23:12:51.918991: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_315' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_315}}]]
2024-02-09 23:12:51.919026: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_318' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_318}}]]
2024-02-09 23:12:51.919062: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_319' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_319}}]]
2024-02-09 23:12:51.919097: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_320' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_320}}]]
2024-02-09 23:12:51.919133: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_321' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_321}}]]
2024-02-09 23:12:51.919169: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_322' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_322}}]]
2024-02-09 23:12:51.919204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_323' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_323}}]]
2024-02-09 23:12:51.919240: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_324' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_324}}]]
2024-02-09 23:12:51.919275: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_327' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_327}}]]
2024-02-09 23:12:51.919311: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_328' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_328}}]]
2024-02-09 23:12:51.919347: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_329' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_329}}]]
2024-02-09 23:12:51.919383: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_330' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_330}}]]
2024-02-09 23:12:51.919418: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_331' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_331}}]]
2024-02-09 23:12:51.919456: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_332' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_332}}]]
2024-02-09 23:12:51.919492: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_333' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_333}}]]
2024-02-09 23:12:51.919527: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_334' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_334}}]]
2024-02-09 23:12:51.919563: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_335' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_335}}]]
2024-02-09 23:12:51.919599: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_339' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_339}}]]
2024-02-09 23:12:51.919634: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_340' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_340}}]]
2024-02-09 23:12:51.919671: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_341' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_341}}]]
2024-02-09 23:12:51.919707: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_342' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_342}}]]
2024-02-09 23:12:51.919743: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_343' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_343}}]]
2024-02-09 23:12:51.919779: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_344' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_344}}]]
2024-02-09 23:12:51.919814: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_345' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_345}}]]
2024-02-09 23:12:51.919849: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_346' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_346}}]]
2024-02-09 23:12:51.919884: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_349' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_349}}]]
2024-02-09 23:12:51.919918: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_350' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_350}}]]
2024-02-09 23:12:51.919954: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_351' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_351}}]]
2024-02-09 23:12:51.919989: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_352' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_352}}]]
2024-02-09 23:12:51.920025: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_353' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_353}}]]
2024-02-09 23:12:51.920061: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_354' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_354}}]]
2024-02-09 23:12:51.920096: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_355' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_355}}]]
2024-02-09 23:12:51.920132: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_356' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_356}}]]
2024-02-09 23:12:51.920167: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_357' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_357}}]]
2024-02-09 23:12:51.920203: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_361' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_361}}]]
2024-02-09 23:12:51.920239: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_362' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_362}}]]
2024-02-09 23:12:51.920274: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_363' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_363}}]]
2024-02-09 23:12:51.920310: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_364' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_364}}]]
2024-02-09 23:12:51.920346: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_365' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_365}}]]
2024-02-09 23:12:51.920381: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_366' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_366}}]]
2024-02-09 23:12:51.920415: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_367' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_367}}]]
2024-02-09 23:12:51.920451: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_368' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_368}}]]
2024-02-09 23:12:51.920487: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_371' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_371}}]]
2024-02-09 23:12:51.920522: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_372' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_372}}]]
2024-02-09 23:12:51.920557: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_373' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_373}}]]
2024-02-09 23:12:51.920592: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_374' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_374}}]]
2024-02-09 23:12:51.920628: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_375' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_375}}]]
2024-02-09 23:12:51.920663: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_376' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_376}}]]
2024-02-09 23:12:51.920699: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_377' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_377}}]]
2024-02-09 23:12:51.920735: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_378' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_378}}]]
2024-02-09 23:12:51.920771: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_380' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_380}}]]
2024-02-09 23:12:51.920807: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_381' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_381}}]]
2024-02-09 23:12:51.920842: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_384' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_384}}]]
2024-02-09 23:12:51.920878: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_385' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_385}}]]
2024-02-09 23:12:51.920914: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_386' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_386}}]]
2024-02-09 23:12:51.920950: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_387' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_387}}]]
2024-02-09 23:12:51.920985: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_388' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_388}}]]
2024-02-09 23:12:51.921022: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_389' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_389}}]]
2024-02-09 23:12:51.921057: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_390' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_390}}]]
2024-02-09 23:12:51.921092: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_393' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_393}}]]
2024-02-09 23:12:51.921128: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_394' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_394}}]]
2024-02-09 23:12:51.921164: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_395' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_395}}]]
2024-02-09 23:12:51.921199: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_396' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_396}}]]
2024-02-09 23:12:51.921235: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_397' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_397}}]]
2024-02-09 23:12:51.921271: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_398' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_398}}]]
2024-02-09 23:12:51.921306: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_399' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_399}}]]
2024-02-09 23:12:51.921341: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_400' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_400}}]]
2024-02-09 23:12:51.921376: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_401' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_401}}]]
2024-02-09 23:12:51.921412: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_405' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_405}}]]
2024-02-09 23:12:51.921453: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_406' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_406}}]]
2024-02-09 23:12:51.921507: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_407' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_407}}]]
2024-02-09 23:12:51.921545: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_408' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_408}}]]
2024-02-09 23:12:51.921582: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_409' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_409}}]]
2024-02-09 23:12:51.921619: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_410' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_410}}]]
2024-02-09 23:12:51.921657: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_411' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_411}}]]
2024-02-09 23:12:51.921694: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_412' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_412}}]]
2024-02-09 23:12:51.921731: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_415' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_415}}]]
2024-02-09 23:12:51.921768: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_416' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_416}}]]
2024-02-09 23:12:51.921806: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_417' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_417}}]]
2024-02-09 23:12:51.921843: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_418' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_418}}]]
2024-02-09 23:12:51.921881: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_419' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_419}}]]
2024-02-09 23:12:51.921918: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_420' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_420}}]]
2024-02-09 23:12:51.921956: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_421' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_421}}]]
2024-02-09 23:12:51.921993: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_422' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_422}}]]
2024-02-09 23:12:51.922030: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_423' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_423}}]]
2024-02-09 23:12:51.922067: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_427' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_427}}]]
2024-02-09 23:12:51.922104: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_428' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_428}}]]
2024-02-09 23:12:51.922141: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_429' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_429}}]]
2024-02-09 23:12:51.922178: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_430' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_430}}]]
2024-02-09 23:12:51.922216: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_431' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_431}}]]
2024-02-09 23:12:51.922252: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_432' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_432}}]]
2024-02-09 23:12:51.922289: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_433' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_433}}]]
2024-02-09 23:12:51.922325: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_434' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_434}}]]
2024-02-09 23:12:51.922363: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_437' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_437}}]]
2024-02-09 23:12:51.922399: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_438' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_438}}]]
2024-02-09 23:12:51.922437: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_439' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_439}}]]
2024-02-09 23:12:51.922473: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_440' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_440}}]]
2024-02-09 23:12:51.922511: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_441' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_441}}]]
2024-02-09 23:12:51.922550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_442' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_442}}]]
2024-02-09 23:12:51.922587: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_443' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_443}}]]
2024-02-09 23:12:51.922624: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_444' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_444}}]]
2024-02-09 23:12:51.922661: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_446' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_446}}]]
2024-02-09 23:12:51.922698: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_447' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_447}}]]
2024-02-09 23:12:51.922736: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_450' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_450}}]]
2024-02-09 23:12:51.922774: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_451' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_451}}]]
2024-02-09 23:12:51.922821: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_452' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_452}}]]
2024-02-09 23:12:51.922858: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_453' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_453}}]]
2024-02-09 23:12:51.922893: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_454' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_454}}]]
2024-02-09 23:12:51.922928: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_455' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_455}}]]
2024-02-09 23:12:51.922964: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_456' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_456}}]]
2024-02-09 23:12:51.922999: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_459' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_459}}]]
2024-02-09 23:12:51.923035: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_460' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_460}}]]
2024-02-09 23:12:51.923071: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_461' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_461}}]]
2024-02-09 23:12:51.923106: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_462' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_462}}]]
2024-02-09 23:12:51.923142: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_463' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_463}}]]
2024-02-09 23:12:51.923179: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_464' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_464}}]]
2024-02-09 23:12:51.923214: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_465' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_465}}]]
2024-02-09 23:12:51.923251: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_466' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_466}}]]
2024-02-09 23:12:51.923286: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_467' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_467}}]]
2024-02-09 23:12:51.923322: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_471' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_471}}]]
2024-02-09 23:12:51.923358: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_472' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_472}}]]
2024-02-09 23:12:51.923394: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_473' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_473}}]]
2024-02-09 23:12:51.923429: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_474' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_474}}]]
2024-02-09 23:12:51.923465: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_475' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_475}}]]
2024-02-09 23:12:51.923501: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_476' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_476}}]]
2024-02-09 23:12:51.923537: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_477' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_477}}]]
2024-02-09 23:12:51.923573: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_478' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_478}}]]
2024-02-09 23:12:51.923609: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_481' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_481}}]]
2024-02-09 23:12:51.923645: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_482' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_482}}]]
2024-02-09 23:12:51.923680: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_483' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_483}}]]
2024-02-09 23:12:51.923716: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_484' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_484}}]]
2024-02-09 23:12:51.923752: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_485' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_485}}]]
2024-02-09 23:12:51.923788: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_486' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_486}}]]
2024-02-09 23:12:51.923824: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_487' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_487}}]]
2024-02-09 23:12:51.923860: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_488' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_488}}]]
2024-02-09 23:12:51.923896: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_489' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_489}}]]
2024-02-09 23:12:51.923932: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_493' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_493}}]]
2024-02-09 23:12:51.923968: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_494' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_494}}]]
2024-02-09 23:12:51.924004: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_495' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_495}}]]
2024-02-09 23:12:51.924040: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_496' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_496}}]]
2024-02-09 23:12:51.924076: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_497' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_497}}]]
2024-02-09 23:12:51.924112: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_498' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_498}}]]
2024-02-09 23:12:51.924148: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_499' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_499}}]]
2024-02-09 23:12:51.924185: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_500' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_500}}]]
2024-02-09 23:12:51.924221: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_503' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_503}}]]
2024-02-09 23:12:51.924256: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_504' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_504}}]]
2024-02-09 23:12:51.924292: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_505' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_505}}]]
2024-02-09 23:12:51.924328: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_506' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_506}}]]
2024-02-09 23:12:51.924365: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_507' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_507}}]]
2024-02-09 23:12:51.924402: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_508' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_508}}]]
2024-02-09 23:12:51.924438: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_509' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_509}}]]
2024-02-09 23:12:51.924474: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_510' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_510}}]]
2024-02-09 23:12:51.924510: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_512' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_512}}]]
2024-02-09 23:12:51.924546: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_513' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_513}}]]
2024-02-09 23:12:51.924581: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_516' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_516}}]]
2024-02-09 23:12:51.924618: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_517' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_517}}]]
2024-02-09 23:12:51.924654: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_518' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_518}}]]
2024-02-09 23:12:51.924690: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_519' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_519}}]]
2024-02-09 23:12:51.924726: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_520' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_520}}]]
2024-02-09 23:12:51.924764: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_521' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_521}}]]
2024-02-09 23:12:51.924800: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_522' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_522}}]]
2024-02-09 23:12:51.924836: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_525' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_525}}]]
2024-02-09 23:12:51.924872: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_526' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_526}}]]
2024-02-09 23:12:51.924908: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_527' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_527}}]]
2024-02-09 23:12:51.924945: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_528' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_528}}]]
2024-02-09 23:12:51.924982: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_529' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_529}}]]
2024-02-09 23:12:51.925019: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_530' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_530}}]]
2024-02-09 23:12:51.925054: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_531' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_531}}]]
2024-02-09 23:12:51.925091: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_532' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_532}}]]
2024-02-09 23:12:51.925127: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_533' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_533}}]]
2024-02-09 23:12:51.925164: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_537' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_537}}]]
2024-02-09 23:12:51.925201: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_538' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_538}}]]
2024-02-09 23:12:51.925237: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_539' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_539}}]]
2024-02-09 23:12:51.925273: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_540' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_540}}]]
2024-02-09 23:12:51.925309: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_541' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_541}}]]
2024-02-09 23:12:51.925345: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_542' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_542}}]]
2024-02-09 23:12:51.925381: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_543' with dtype float and shape [?,1,1,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_543}}]]
2024-02-09 23:12:51.925418: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_544' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_544}}]]
2024-02-09 23:12:51.925475: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_547' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_547}}]]
2024-02-09 23:12:51.925514: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_548' with dtype float and shape [32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_548}}]]
2024-02-09 23:12:51.925552: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_549' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_549}}]]
2024-02-09 23:12:51.925590: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_550' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_550}}]]
2024-02-09 23:12:51.925628: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_551' with dtype float and shape [?,?,?,32,32]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_551}}]]
2024-02-09 23:12:51.925666: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_552' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_552}}]]
2024-02-09 23:12:51.925704: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_553' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_553}}]]
2024-02-09 23:12:51.925744: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_554' with dtype float and shape [?,?,?,1024]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_554}}]]
2024-02-09 23:12:51.925781: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_555' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_555}}]]
2024-02-09 23:12:51.925818: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_557' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_557}}]]
2024-02-09 23:12:51.925855: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_563' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_563}}]]
2024-02-09 23:12:51.925893: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_564' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_564}}]]
2024-02-09 23:12:51.925929: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_565' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_565}}]]
2024-02-09 23:12:51.925967: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_566' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_566}}]]
2024-02-09 23:12:51.926005: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_567' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_567}}]]
2024-02-09 23:12:51.926043: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_568' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_568}}]]
2024-02-09 23:12:51.926081: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_569' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_569}}]]
2024-02-09 23:12:51.926119: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_570' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_570}}]]
2024-02-09 23:12:51.926156: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_575' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_575}}]]
2024-02-09 23:12:51.926194: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_576' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_576}}]]
2024-02-09 23:12:51.926232: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_577' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_577}}]]
2024-02-09 23:12:51.926270: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_578' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_578}}]]
2024-02-09 23:12:51.926307: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_579' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_579}}]]
2024-02-09 23:12:51.926344: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_580' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_580}}]]
2024-02-09 23:12:51.926382: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_581' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_581}}]]
2024-02-09 23:12:51.926419: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_582' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_582}}]]
2024-02-09 23:12:51.926456: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_584' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_584}}]]
2024-02-09 23:12:51.926493: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_585' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_585}}]]
2024-02-09 23:12:51.926531: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_588' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_588}}]]
2024-02-09 23:12:51.926568: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_589' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_589}}]]
2024-02-09 23:12:51.926606: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_590' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_590}}]]
2024-02-09 23:12:51.926643: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_591' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_591}}]]
2024-02-09 23:12:51.926691: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_592' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_592}}]]
2024-02-09 23:12:51.926728: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_593' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_593}}]]
2024-02-09 23:12:51.926764: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_594' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_594}}]]
2024-02-09 23:12:51.926800: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_597' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_597}}]]
2024-02-09 23:12:51.926836: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_598' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_598}}]]
2024-02-09 23:12:51.926872: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_599' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_599}}]]
2024-02-09 23:12:51.926909: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_600' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_600}}]]
2024-02-09 23:12:51.926945: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_601' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_601}}]]
2024-02-09 23:12:51.926981: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_602' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_602}}]]
2024-02-09 23:12:51.927017: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_603' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_603}}]]
2024-02-09 23:12:51.927053: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_604' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_604}}]]
2024-02-09 23:12:51.927090: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_608' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_608}}]]
2024-02-09 23:12:51.927127: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_609' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_609}}]]
2024-02-09 23:12:51.927163: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_610' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_610}}]]
2024-02-09 23:12:51.927199: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_611' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_611}}]]
2024-02-09 23:12:51.927234: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_612' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_612}}]]
2024-02-09 23:12:51.927270: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_613' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_613}}]]
2024-02-09 23:12:51.927306: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_614' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_614}}]]
2024-02-09 23:12:51.927343: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_615' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_615}}]]
2024-02-09 23:12:51.927379: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_618' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_618}}]]
2024-02-09 23:12:51.927415: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_619' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_619}}]]
2024-02-09 23:12:51.927450: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_620' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_620}}]]
2024-02-09 23:12:51.927486: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_621' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_621}}]]
2024-02-09 23:12:51.927523: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_622' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_622}}]]
2024-02-09 23:12:51.927559: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_623' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_623}}]]
2024-02-09 23:12:51.927595: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_624' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_624}}]]
2024-02-09 23:12:51.927631: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_625' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_625}}]]
2024-02-09 23:12:51.927667: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_626' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_626}}]]
2024-02-09 23:12:51.927703: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_627' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_627}}]]
2024-02-09 23:12:51.927739: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_631' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_631}}]]
2024-02-09 23:12:51.927775: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_632' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_632}}]]
2024-02-09 23:12:51.927811: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_633' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_633}}]]
2024-02-09 23:12:51.927847: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_634' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_634}}]]
2024-02-09 23:12:51.927884: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_635' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_635}}]]
2024-02-09 23:12:51.927919: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_636' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_636}}]]
2024-02-09 23:12:51.927955: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_637' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_637}}]]
2024-02-09 23:12:51.927990: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_638' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_638}}]]
2024-02-09 23:12:51.928026: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_641' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_641}}]]
2024-02-09 23:12:51.928062: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_642' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_642}}]]
2024-02-09 23:12:51.928097: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_643' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_643}}]]
2024-02-09 23:12:51.928133: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_644' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_644}}]]
2024-02-09 23:12:51.928169: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_645' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_645}}]]
2024-02-09 23:12:51.928204: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_646' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_646}}]]
2024-02-09 23:12:51.928258: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_647' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_647}}]]
2024-02-09 23:12:51.928296: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_648' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_648}}]]
2024-02-09 23:12:51.928332: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_650' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_650}}]]
2024-02-09 23:12:51.928370: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_651' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_651}}]]
2024-02-09 23:12:51.928406: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_654' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_654}}]]
2024-02-09 23:12:51.928444: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_655' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_655}}]]
2024-02-09 23:12:51.928482: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_656' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_656}}]]
2024-02-09 23:12:51.928519: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_657' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_657}}]]
2024-02-09 23:12:51.928557: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_658' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_658}}]]
2024-02-09 23:12:51.928593: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_659' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_659}}]]
2024-02-09 23:12:51.928630: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_660' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_660}}]]
2024-02-09 23:12:51.928668: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_663' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_663}}]]
2024-02-09 23:12:51.928706: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_664' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_664}}]]
2024-02-09 23:12:51.928743: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_665' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_665}}]]
2024-02-09 23:12:51.928780: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_666' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_666}}]]
2024-02-09 23:12:51.928818: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_667' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_667}}]]
2024-02-09 23:12:51.928855: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_668' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_668}}]]
2024-02-09 23:12:51.928893: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_669' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_669}}]]
2024-02-09 23:12:51.928930: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_670' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_670}}]]
2024-02-09 23:12:51.928967: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_671' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_671}}]]
2024-02-09 23:12:51.929004: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_675' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_675}}]]
2024-02-09 23:12:51.929041: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_676' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_676}}]]
2024-02-09 23:12:51.929079: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_677' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_677}}]]
2024-02-09 23:12:51.929116: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_678' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_678}}]]
2024-02-09 23:12:51.929154: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_679' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_679}}]]
2024-02-09 23:12:51.929191: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_680' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_680}}]]
2024-02-09 23:12:51.929228: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_681' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_681}}]]
2024-02-09 23:12:51.929265: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_682' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_682}}]]
2024-02-09 23:12:51.929303: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_685' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_685}}]]
2024-02-09 23:12:51.929341: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_686' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_686}}]]
2024-02-09 23:12:51.929379: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_687' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_687}}]]
2024-02-09 23:12:51.929416: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_688' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_688}}]]
2024-02-09 23:12:51.929459: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_689' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_689}}]]
2024-02-09 23:12:51.929497: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_690' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_690}}]]
2024-02-09 23:12:51.929534: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_691' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_691}}]]
2024-02-09 23:12:51.929571: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_692' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_692}}]]
2024-02-09 23:12:51.929608: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_693' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_693}}]]
2024-02-09 23:12:51.929645: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_697' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_697}}]]
2024-02-09 23:12:51.929683: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_698' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_698}}]]
2024-02-09 23:12:51.929721: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_699' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_699}}]]
2024-02-09 23:12:51.929758: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_700' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_700}}]]
2024-02-09 23:12:51.929794: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_701' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_701}}]]
2024-02-09 23:12:51.929833: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_702' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_702}}]]
2024-02-09 23:12:51.929870: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_703' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_703}}]]
2024-02-09 23:12:51.929908: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_704' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_704}}]]
2024-02-09 23:12:51.929945: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_707' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_707}}]]
2024-02-09 23:12:51.929983: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_708' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_708}}]]
2024-02-09 23:12:51.930020: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_709' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_709}}]]
2024-02-09 23:12:51.930058: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_710' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_710}}]]
2024-02-09 23:12:51.930097: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_711' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_711}}]]
2024-02-09 23:12:51.930134: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_712' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_712}}]]
2024-02-09 23:12:51.930172: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_713' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_713}}]]
2024-02-09 23:12:51.930209: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_714' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_714}}]]
2024-02-09 23:12:51.930246: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_716' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_716}}]]
2024-02-09 23:12:51.930283: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_717' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_717}}]]
2024-02-09 23:12:51.930321: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_720' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_720}}]]
2024-02-09 23:12:51.930358: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_721' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_721}}]]
2024-02-09 23:12:51.930395: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_722' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_722}}]]
2024-02-09 23:12:51.930433: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_723' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_723}}]]
2024-02-09 23:12:51.930470: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_724' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_724}}]]
2024-02-09 23:12:51.930508: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_725' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_725}}]]
2024-02-09 23:12:51.930546: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_726' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_726}}]]
2024-02-09 23:12:51.930584: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_729' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_729}}]]
2024-02-09 23:12:51.930621: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_730' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_730}}]]
2024-02-09 23:12:51.930659: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_731' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_731}}]]
2024-02-09 23:12:51.930706: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_732' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_732}}]]
2024-02-09 23:12:51.930743: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_733' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_733}}]]
2024-02-09 23:12:51.930780: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_734' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_734}}]]
2024-02-09 23:12:51.930817: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_735' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_735}}]]
2024-02-09 23:12:51.930853: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_736' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_736}}]]
2024-02-09 23:12:51.930889: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_737' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_737}}]]
2024-02-09 23:12:51.930926: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_741' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_741}}]]
2024-02-09 23:12:51.930963: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_742' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_742}}]]
2024-02-09 23:12:51.931000: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_743' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_743}}]]
2024-02-09 23:12:51.931037: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_744' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_744}}]]
2024-02-09 23:12:51.931073: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_745' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_745}}]]
2024-02-09 23:12:51.931110: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_746' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_746}}]]
2024-02-09 23:12:51.931146: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_747' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_747}}]]
2024-02-09 23:12:51.931183: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_748' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_748}}]]
2024-02-09 23:12:51.931219: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_751' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_751}}]]
2024-02-09 23:12:51.931256: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_752' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_752}}]]
2024-02-09 23:12:51.931292: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_753' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_753}}]]
2024-02-09 23:12:51.931328: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_754' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_754}}]]
2024-02-09 23:12:51.931364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_755' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_755}}]]
2024-02-09 23:12:51.931400: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_756' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_756}}]]
2024-02-09 23:12:51.931437: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_757' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_757}}]]
2024-02-09 23:12:51.931473: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_758' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_758}}]]
2024-02-09 23:12:51.931510: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_759' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_759}}]]
2024-02-09 23:12:51.931546: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_763' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_763}}]]
2024-02-09 23:12:51.931582: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_764' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_764}}]]
2024-02-09 23:12:51.931619: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_765' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_765}}]]
2024-02-09 23:12:51.931656: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_766' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_766}}]]
2024-02-09 23:12:51.931694: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_767' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_767}}]]
2024-02-09 23:12:51.931730: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_768' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_768}}]]
2024-02-09 23:12:51.931766: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_769' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_769}}]]
2024-02-09 23:12:51.931802: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_770' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_770}}]]
2024-02-09 23:12:51.931838: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_773' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_773}}]]
2024-02-09 23:12:51.931875: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_774' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_774}}]]
2024-02-09 23:12:51.931911: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_775' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_775}}]]
2024-02-09 23:12:51.931948: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_776' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_776}}]]
2024-02-09 23:12:51.931984: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_777' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_777}}]]
2024-02-09 23:12:51.932021: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_778' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_778}}]]
2024-02-09 23:12:51.932058: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_779' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_779}}]]
2024-02-09 23:12:51.932095: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_780' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_780}}]]
2024-02-09 23:12:51.932129: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_782' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_782}}]]
2024-02-09 23:12:51.932165: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_783' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_783}}]]
2024-02-09 23:12:51.932202: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_786' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_786}}]]
2024-02-09 23:12:51.932239: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_787' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_787}}]]
2024-02-09 23:12:51.932275: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_788' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_788}}]]
2024-02-09 23:12:51.932308: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_789' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_789}}]]
2024-02-09 23:12:51.932342: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_790' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_790}}]]
2024-02-09 23:12:51.932378: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_791' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_791}}]]
2024-02-09 23:12:51.932414: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_792' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_792}}]]
2024-02-09 23:12:51.932451: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_795' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_795}}]]
2024-02-09 23:12:51.932488: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_796' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_796}}]]
2024-02-09 23:12:51.932524: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_797' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_797}}]]
2024-02-09 23:12:51.932557: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_798' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_798}}]]
2024-02-09 23:12:51.932594: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_799' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_799}}]]
2024-02-09 23:12:51.932631: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_800' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_800}}]]
2024-02-09 23:12:51.932667: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_801' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_801}}]]
2024-02-09 23:12:51.932703: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_802' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_802}}]]
2024-02-09 23:12:51.932739: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_803' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_803}}]]
2024-02-09 23:12:51.932776: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_807' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_807}}]]
2024-02-09 23:12:51.932812: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_808' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_808}}]]
2024-02-09 23:12:51.932848: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_809' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_809}}]]
2024-02-09 23:12:51.932885: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_810' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_810}}]]
2024-02-09 23:12:51.932921: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_811' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_811}}]]
2024-02-09 23:12:51.932959: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_812' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_812}}]]
2024-02-09 23:12:51.932992: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_813' with dtype float and shape [?,1,1,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_813}}]]
2024-02-09 23:12:51.933029: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_814' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_814}}]]
2024-02-09 23:12:51.933066: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_817' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_817}}]]
2024-02-09 23:12:51.933102: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_818' with dtype float and shape [32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_818}}]]
2024-02-09 23:12:51.933137: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_819' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_819}}]]
2024-02-09 23:12:51.933175: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_820' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_820}}]]
2024-02-09 23:12:51.933212: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_821' with dtype float and shape [?,?,?,32,16]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_821}}]]
2024-02-09 23:12:51.933245: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_822' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_822}}]]
2024-02-09 23:12:51.933279: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_823' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_823}}]]
2024-02-09 23:12:51.933315: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_824' with dtype float and shape [?,?,?,512]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_824}}]]
2024-02-09 23:12:51.933352: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_825' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_825}}]]
2024-02-09 23:12:51.933389: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_827' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_827}}]]
2024-02-09 23:12:51.933426: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_833' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_833}}]]
2024-02-09 23:12:51.933485: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_834' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_834}}]]
2024-02-09 23:12:51.933524: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_835' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_835}}]]
2024-02-09 23:12:51.933563: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_836' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_836}}]]
2024-02-09 23:12:51.933598: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_837' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_837}}]]
2024-02-09 23:12:51.933632: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_838' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_838}}]]
2024-02-09 23:12:51.933667: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_839' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_839}}]]
2024-02-09 23:12:51.933704: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_840' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_840}}]]
2024-02-09 23:12:51.933739: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_845' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_845}}]]
2024-02-09 23:12:51.933774: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_846' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_846}}]]
2024-02-09 23:12:51.933809: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_847' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_847}}]]
2024-02-09 23:12:51.933847: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_848' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_848}}]]
2024-02-09 23:12:51.933886: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_849' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_849}}]]
2024-02-09 23:12:51.933923: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_850' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_850}}]]
2024-02-09 23:12:51.933962: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_851' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_851}}]]
2024-02-09 23:12:51.933999: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_852' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_852}}]]
2024-02-09 23:12:51.934034: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_854' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_854}}]]
2024-02-09 23:12:51.934069: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_855' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_855}}]]
2024-02-09 23:12:51.934107: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_858' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_858}}]]
2024-02-09 23:12:51.934145: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_859' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_859}}]]
2024-02-09 23:12:51.934183: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_860' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_860}}]]
2024-02-09 23:12:51.934217: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_861' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_861}}]]
2024-02-09 23:12:51.934253: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_862' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_862}}]]
2024-02-09 23:12:51.934289: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_863' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_863}}]]
2024-02-09 23:12:51.934326: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_864' with dtype float and shape [?,1,1,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_864}}]]
2024-02-09 23:12:51.934364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_867' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_867}}]]
2024-02-09 23:12:51.934402: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_868' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_868}}]]
2024-02-09 23:12:51.934441: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_869' with dtype float and shape [32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_869}}]]
2024-02-09 23:12:51.934476: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_870' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_870}}]]
2024-02-09 23:12:51.934511: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_871' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_871}}]]
2024-02-09 23:12:51.934549: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_872' with dtype float and shape [?,?,?,32,4]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_872}}]]
2024-02-09 23:12:51.934586: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_873' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_873}}]]
2024-02-09 23:12:51.934625: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_874' with dtype float and shape [?,?,?,128]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_874}}]]
2024-02-09 23:12:51.934660: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_878' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_878}}]]
2024-02-09 23:12:51.934705: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_879' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_879}}]]
2024-02-09 23:12:51.934741: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_880' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_880}}]]
2024-02-09 23:12:51.934778: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_881' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_881}}]]
2024-02-09 23:12:51.934815: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_882' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_882}}]]
2024-02-09 23:12:51.934851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_883' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_883}}]]
2024-02-09 23:12:51.934887: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_884' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_884}}]]
2024-02-09 23:12:51.934923: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_885' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_885}}]]
2024-02-09 23:12:51.934960: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_888' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_888}}]]
2024-02-09 23:12:51.934996: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_889' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_889}}]]
2024-02-09 23:12:51.935032: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_890' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_890}}]]
2024-02-09 23:12:51.935069: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_891' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_891}}]]
2024-02-09 23:12:51.935106: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_892' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_892}}]]
2024-02-09 23:12:51.935140: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_893' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_893}}]]
2024-02-09 23:12:51.935174: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_894' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_894}}]]
2024-02-09 23:12:51.935210: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_895' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_895}}]]
2024-02-09 23:12:51.935246: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_896' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_896}}]]
2024-02-09 23:12:51.935283: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_897' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_897}}]]
2024-02-09 23:12:51.935316: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_901' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_901}}]]
2024-02-09 23:12:51.935351: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_902' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_902}}]]
2024-02-09 23:12:51.935386: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_903' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_903}}]]
2024-02-09 23:12:51.935423: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_904' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_904}}]]
2024-02-09 23:12:51.935458: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_905' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_905}}]]
2024-02-09 23:12:51.935494: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_906' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_906}}]]
2024-02-09 23:12:51.935530: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_907' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_907}}]]
2024-02-09 23:12:51.935567: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_908' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_908}}]]
2024-02-09 23:12:51.935603: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_911' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_911}}]]
2024-02-09 23:12:51.935639: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_912' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_912}}]]
2024-02-09 23:12:51.935676: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_913' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_913}}]]
2024-02-09 23:12:51.935712: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_914' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_914}}]]
2024-02-09 23:12:51.935748: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_915' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_915}}]]
2024-02-09 23:12:51.935784: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_916' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_916}}]]
2024-02-09 23:12:51.935821: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_917' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_917}}]]
2024-02-09 23:12:51.935854: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_918' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_918}}]]
2024-02-09 23:12:51.935891: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_920' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_920}}]]
2024-02-09 23:12:51.935927: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_921' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_921}}]]
2024-02-09 23:12:51.935963: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_924' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_924}}]]
2024-02-09 23:12:51.935999: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_925' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_925}}]]
2024-02-09 23:12:51.936036: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_926' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_926}}]]
2024-02-09 23:12:51.936072: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_927' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_927}}]]
2024-02-09 23:12:51.936108: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_928' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_928}}]]
2024-02-09 23:12:51.936145: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_929' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_929}}]]
2024-02-09 23:12:51.936182: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_930' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_930}}]]
2024-02-09 23:12:51.936218: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_933' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_933}}]]
2024-02-09 23:12:51.936252: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_934' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_934}}]]
2024-02-09 23:12:51.936289: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_935' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_935}}]]
2024-02-09 23:12:51.936325: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_936' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_936}}]]
2024-02-09 23:12:51.936361: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_937' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_937}}]]
2024-02-09 23:12:51.936398: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_938' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_938}}]]
2024-02-09 23:12:51.936435: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_939' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_939}}]]
2024-02-09 23:12:51.936471: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_940' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_940}}]]
2024-02-09 23:12:51.936504: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_941' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_941}}]]
2024-02-09 23:12:51.936541: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_945' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_945}}]]
2024-02-09 23:12:51.936576: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_946' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_946}}]]
2024-02-09 23:12:51.936613: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_947' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_947}}]]
2024-02-09 23:12:51.936649: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_948' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_948}}]]
2024-02-09 23:12:51.936686: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_949' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_949}}]]
2024-02-09 23:12:51.936722: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_950' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_950}}]]
2024-02-09 23:12:51.936758: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_951' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_951}}]]
2024-02-09 23:12:51.936794: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_952' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_952}}]]
2024-02-09 23:12:51.936830: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_955' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_955}}]]
2024-02-09 23:12:51.936867: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_956' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_956}}]]
2024-02-09 23:12:51.936904: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_957' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_957}}]]
2024-02-09 23:12:51.936941: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_958' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_958}}]]
2024-02-09 23:12:51.936977: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_959' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_959}}]]
2024-02-09 23:12:51.937014: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_960' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_960}}]]
2024-02-09 23:12:51.937051: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_961' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_961}}]]
2024-02-09 23:12:51.937088: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_962' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_962}}]]
2024-02-09 23:12:51.937125: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_963' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_963}}]]
2024-02-09 23:12:51.937161: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_967' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_967}}]]
2024-02-09 23:12:51.937196: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_968' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_968}}]]
2024-02-09 23:12:51.937232: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_969' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_969}}]]
2024-02-09 23:12:51.937268: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_970' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_970}}]]
2024-02-09 23:12:51.937305: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_971' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_971}}]]
2024-02-09 23:12:51.937341: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_972' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_972}}]]
2024-02-09 23:12:51.937377: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_973' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_973}}]]
2024-02-09 23:12:51.937413: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_974' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_974}}]]
2024-02-09 23:12:51.937454: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_977' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_977}}]]
2024-02-09 23:12:51.937509: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_978' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_978}}]]
2024-02-09 23:12:51.937548: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_979' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_979}}]]
2024-02-09 23:12:51.937585: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_980' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_980}}]]
2024-02-09 23:12:51.937624: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_981' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_981}}]]
2024-02-09 23:12:51.937662: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_982' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_982}}]]
2024-02-09 23:12:51.937700: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_983' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_983}}]]
2024-02-09 23:12:51.937738: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_984' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_984}}]]
2024-02-09 23:12:51.937775: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_986' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_986}}]]
2024-02-09 23:12:51.937813: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_987' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_987}}]]
2024-02-09 23:12:51.937851: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_990' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_990}}]]
2024-02-09 23:12:51.937889: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_991' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_991}}]]
2024-02-09 23:12:51.937928: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_992' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_992}}]]
2024-02-09 23:12:51.937966: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_993' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_993}}]]
2024-02-09 23:12:51.938004: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_994' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_994}}]]
2024-02-09 23:12:51.938042: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_995' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_995}}]]
2024-02-09 23:12:51.938080: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_996' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_996}}]]
2024-02-09 23:12:51.938117: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_999' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_999}}]]
2024-02-09 23:12:51.938154: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1000' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1000}}]]
2024-02-09 23:12:51.938193: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1001' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1001}}]]
2024-02-09 23:12:51.938231: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1002' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1002}}]]
2024-02-09 23:12:51.938270: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1003' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1003}}]]
2024-02-09 23:12:51.938308: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1004' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1004}}]]
2024-02-09 23:12:51.938346: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1005' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1005}}]]
2024-02-09 23:12:51.938384: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1006' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1006}}]]
2024-02-09 23:12:51.938421: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1007' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1007}}]]
2024-02-09 23:12:51.938459: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1011' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1011}}]]
2024-02-09 23:12:51.938498: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1012' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1012}}]]
2024-02-09 23:12:51.938536: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1013' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1013}}]]
2024-02-09 23:12:51.938574: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1014' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1014}}]]
2024-02-09 23:12:51.938612: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1015' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1015}}]]
2024-02-09 23:12:51.938650: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1016' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1016}}]]
2024-02-09 23:12:51.938697: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1017' with dtype float and shape [?,1,1,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1017}}]]
2024-02-09 23:12:51.938734: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1018' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1018}}]]
2024-02-09 23:12:51.938770: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1021' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1021}}]]
2024-02-09 23:12:51.938807: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1022' with dtype float and shape [32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1022}}]]
2024-02-09 23:12:51.938843: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1023' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1023}}]]
2024-02-09 23:12:51.938880: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1024' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1024}}]]
2024-02-09 23:12:51.938918: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1025' with dtype float and shape [?,?,?,32,8]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1025}}]]
2024-02-09 23:12:51.938954: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1026' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1026}}]]
2024-02-09 23:12:51.938991: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1027' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1027}}]]
2024-02-09 23:12:51.939025: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1028' with dtype float and shape [?,?,?,256]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1028}}]]
2024-02-09 23:12:51.939059: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1029' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1029}}]]
2024-02-09 23:12:51.939094: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1031' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1031}}]]
2024-02-09 23:12:51.939128: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1037' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1037}}]]
2024-02-09 23:12:51.939162: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1038' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1038}}]]
2024-02-09 23:12:51.939196: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1039' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1039}}]]
2024-02-09 23:12:51.939230: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1040' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1040}}]]
2024-02-09 23:12:51.939264: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1041' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1041}}]]
2024-02-09 23:12:51.939298: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1042' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1042}}]]
2024-02-09 23:12:51.939331: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1043' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1043}}]]
2024-02-09 23:12:51.939364: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1044' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1044}}]]
2024-02-09 23:12:51.939399: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1049' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1049}}]]
2024-02-09 23:12:51.939432: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1050' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1050}}]]
2024-02-09 23:12:51.939466: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1051' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1051}}]]
2024-02-09 23:12:51.939499: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1052' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1052}}]]
2024-02-09 23:12:51.939534: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1053' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1053}}]]
2024-02-09 23:12:51.939568: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1054' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1054}}]]
2024-02-09 23:12:51.939602: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1055' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1055}}]]
2024-02-09 23:12:51.939636: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1056' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1056}}]]
2024-02-09 23:12:51.939671: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1058' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1058}}]]
2024-02-09 23:12:51.939704: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1059' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1059}}]]
2024-02-09 23:12:51.939738: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1062' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1062}}]]
2024-02-09 23:12:51.939772: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1063' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1063}}]]
2024-02-09 23:12:51.939806: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1064' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1064}}]]
2024-02-09 23:12:51.939839: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1065' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1065}}]]
2024-02-09 23:12:51.939873: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1066' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1066}}]]
2024-02-09 23:12:51.939907: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1067' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1067}}]]
2024-02-09 23:12:51.939940: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1068' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1068}}]]
2024-02-09 23:12:51.939975: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1071' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1071}}]]
2024-02-09 23:12:51.940008: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1072' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1072}}]]
2024-02-09 23:12:51.940046: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1073' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1073}}]]
2024-02-09 23:12:51.940079: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1074' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1074}}]]
2024-02-09 23:12:51.940113: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1075' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1075}}]]
2024-02-09 23:12:51.940146: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1076' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1076}}]]
2024-02-09 23:12:51.940180: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1077' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1077}}]]
2024-02-09 23:12:51.940214: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1078' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1078}}]]
2024-02-09 23:12:51.940248: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1082' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1082}}]]
2024-02-09 23:12:51.940281: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1083' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1083}}]]
2024-02-09 23:12:51.940315: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1084' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1084}}]]
2024-02-09 23:12:51.940348: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1085' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1085}}]]
2024-02-09 23:12:51.940382: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1086' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1086}}]]
2024-02-09 23:12:51.940415: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1087' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1087}}]]
2024-02-09 23:12:51.940449: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1088' with dtype float and shape [?,1,1,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1088}}]]
2024-02-09 23:12:51.940483: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1089' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1089}}]]
2024-02-09 23:12:51.940516: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1092' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1092}}]]
2024-02-09 23:12:51.940550: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1093' with dtype float and shape [32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1093}}]]
2024-02-09 23:12:51.940584: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1094' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1094}}]]
2024-02-09 23:12:51.940618: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1095' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1095}}]]
2024-02-09 23:12:51.940652: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1096' with dtype float and shape [?,?,?,32,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1096}}]]
2024-02-09 23:12:51.940689: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1097' with dtype float and shape [?,1,1,32,1]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1097}}]]
2024-02-09 23:12:51.940723: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1098' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1098}}]]
2024-02-09 23:12:51.940757: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1101' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1101}}]]
2024-02-09 23:12:51.940791: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1102' with dtype float and shape [?,?,?,64]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1102}}]]
2024-02-09 23:12:51.940825: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1103' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1103}}]]
2024-02-09 23:12:51.940859: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1105' with dtype int32 and shape [4,2]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1105}}]]
2024-02-09 23:12:51.940893: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1106' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1106}}]]
2024-02-09 23:12:51.940927: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1111' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1111}}]]
2024-02-09 23:12:51.940961: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1112' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1112}}]]
2024-02-09 23:12:51.940994: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1113' with dtype float
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1113}}]]
2024-02-09 23:12:51.941028: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1114' with dtype float and shape [?,?,?,3]
[[{{node gradients/StatefulPartitionedCall_grad/StatefulPartitionedCall_1114}}]]
1/101 [..............................] - ETA: 45:51 - loss: 5.7132 - accuracy: 0.1797
2/101 [..............................] - ETA: 15:19 - loss: 5.3230 - accuracy: 0.1953
3/101 [..............................] - ETA: 15:06 - loss: 4.8895 - accuracy: 0.1875
4/101 [>.............................] - ETA: 14:56 - loss: 4.6401 - accuracy: 0.2012
5/101 [>.............................] - ETA: 14:47 - loss: 4.3587 - accuracy: 0.2188
6/101 [>.............................] - ETA: 14:38 - loss: 4.0658 - accuracy: 0.2409
7/101 [=>............................] - ETA: 14:29 - loss: 3.8257 - accuracy: 0.2623
8/101 [=>............................] - ETA: 14:19 - loss: 3.5840 - accuracy: 0.2920
9/101 [=>............................] - ETA: 14:10 - loss: 3.3894 - accuracy: 0.3142
10/101 [=>............................] - ETA: 14:00 - loss: 3.1832 - accuracy: 0.3422
11/101 [==>...........................] - ETA: 13:51 - loss: 2.9984 - accuracy: 0.3700
12/101 [==>...........................] - ETA: 13:42 - loss: 2.8426 - accuracy: 0.3952
13/101 [==>...........................] - ETA: 13:34 - loss: 2.6978 - accuracy: 0.4165
14/101 [===>..........................] - ETA: 13:24 - loss: 2.5742 - accuracy: 0.4358
15/101 [===>..........................] - ETA: 13:15 - loss: 2.4525 - accuracy: 0.4604
16/101 [===>..........................] - ETA: 13:06 - loss: 2.3284 - accuracy: 0.4868
17/101 [====>.........................] - ETA: 12:57 - loss: 2.2201 - accuracy: 0.5097
18/101 [====>.........................] - ETA: 12:47 - loss: 2.1165 - accuracy: 0.5295
19/101 [====>.........................] - ETA: 12:38 - loss: 2.0292 - accuracy: 0.5473
20/101 [====>.........................] - ETA: 12:29 - loss: 1.9488 - accuracy: 0.5645
21/101 [=====>........................] - ETA: 12:19 - loss: 1.8767 - accuracy: 0.5800
22/101 [=====>........................] - ETA: 12:10 - loss: 1.8060 - accuracy: 0.5955
23/101 [=====>........................] - ETA: 12:01 - loss: 1.7449 - accuracy: 0.6070
24/101 [======>.......................] - ETA: 11:52 - loss: 1.6832 - accuracy: 0.6204
25/101 [======>.......................] - ETA: 11:42 - loss: 1.6343 - accuracy: 0.6300
26/101 [======>.......................] - ETA: 11:33 - loss: 1.5874 - accuracy: 0.6391
27/101 [=======>......................] - ETA: 11:24 - loss: 1.5395 - accuracy: 0.6496
28/101 [=======>......................] - ETA: 11:15 - loss: 1.4937 - accuracy: 0.6593
29/101 [=======>......................] - ETA: 11:05 - loss: 1.4515 - accuracy: 0.6678
30/101 [=======>......................] - ETA: 10:56 - loss: 1.4099 - accuracy: 0.6768
31/101 [========>.....................] - ETA: 10:47 - loss: 1.3711 - accuracy: 0.6850
32/101 [========>.....................] - ETA: 10:38 - loss: 1.3381 - accuracy: 0.6924
33/101 [========>.....................] - ETA: 10:28 - loss: 1.3028 - accuracy: 0.7003
34/101 [=========>....................] - ETA: 10:19 - loss: 1.2755 - accuracy: 0.7066
35/101 [=========>....................] - ETA: 10:10 - loss: 1.2452 - accuracy: 0.7129
36/101 [=========>....................] - ETA: 10:01 - loss: 1.2186 - accuracy: 0.7183
37/101 [=========>....................] - ETA: 9:51 - loss: 1.1913 - accuracy: 0.7245
38/101 [==========>...................] - ETA: 9:42 - loss: 1.1668 - accuracy: 0.7299
39/101 [==========>...................] - ETA: 9:33 - loss: 1.1378 - accuracy: 0.7368
40/101 [==========>...................] - ETA: 9:24 - loss: 1.1141 - accuracy: 0.7420
41/101 [===========>..................] - ETA: 9:14 - loss: 1.0892 - accuracy: 0.7473
42/101 [===========>..................] - ETA: 9:05 - loss: 1.0653 - accuracy: 0.7526
43/101 [===========>..................] - ETA: 8:56 - loss: 1.0430 - accuracy: 0.7576
44/101 [============>.................] - ETA: 8:47 - loss: 1.0243 - accuracy: 0.7617
45/101 [============>.................] - ETA: 8:37 - loss: 1.0074 - accuracy: 0.7648
46/101 [============>.................] - ETA: 8:28 - loss: 0.9881 - accuracy: 0.7689
47/101 [============>.................] - ETA: 8:19 - loss: 0.9711 - accuracy: 0.7723
48/101 [=============>................] - ETA: 8:10 - loss: 0.9529 - accuracy: 0.7765
49/101 [=============>................] - ETA: 8:00 - loss: 0.9375 - accuracy: 0.7798
50/101 [=============>................] - ETA: 7:51 - loss: 0.9236 - accuracy: 0.7823
51/101 [==============>...............] - ETA: 7:42 - loss: 0.9096 - accuracy: 0.7854
52/101 [==============>...............] - ETA: 7:33 - loss: 0.8955 - accuracy: 0.7880
53/101 [==============>...............] - ETA: 7:23 - loss: 0.8823 - accuracy: 0.7913
54/101 [===============>..............] - ETA: 7:14 - loss: 0.8676 - accuracy: 0.7948
55/101 [===============>..............] - ETA: 7:05 - loss: 0.8529 - accuracy: 0.7983
56/101 [===============>..............] - ETA: 6:56 - loss: 0.8392 - accuracy: 0.8011
57/101 [===============>..............] - ETA: 6:46 - loss: 0.8309 - accuracy: 0.8025
58/101 [================>.............] - ETA: 6:37 - loss: 0.8184 - accuracy: 0.8051
59/101 [================>.............] - ETA: 6:28 - loss: 0.8062 - accuracy: 0.8079
60/101 [================>.............] - ETA: 6:19 - loss: 0.7953 - accuracy: 0.8102
61/101 [=================>............] - ETA: 6:09 - loss: 0.7830 - accuracy: 0.8130
62/101 [=================>............] - ETA: 6:00 - loss: 0.7715 - accuracy: 0.8157
63/101 [=================>............] - ETA: 5:51 - loss: 0.7624 - accuracy: 0.8177
64/101 [==================>...........] - ETA: 5:42 - loss: 0.7541 - accuracy: 0.8201
65/101 [==================>...........] - ETA: 5:32 - loss: 0.7481 - accuracy: 0.8214
66/101 [==================>...........] - ETA: 5:23 - loss: 0.7391 - accuracy: 0.8235
67/101 [==================>...........] - ETA: 5:14 - loss: 0.7309 - accuracy: 0.8250
68/101 [===================>..........] - ETA: 5:05 - loss: 0.7222 - accuracy: 0.8267
69/101 [===================>..........] - ETA: 4:55 - loss: 0.7136 - accuracy: 0.8287
70/101 [===================>..........] - ETA: 4:46 - loss: 0.7052 - accuracy: 0.8307
71/101 [====================>.........] - ETA: 4:37 - loss: 0.6974 - accuracy: 0.8325
72/101 [====================>.........] - ETA: 4:28 - loss: 0.6889 - accuracy: 0.8345
73/101 [====================>.........] - ETA: 4:18 - loss: 0.6810 - accuracy: 0.8362
74/101 [====================>.........] - ETA: 4:09 - loss: 0.6729 - accuracy: 0.8378
75/101 [=====================>........] - ETA: 4:00 - loss: 0.6658 - accuracy: 0.8392
76/101 [=====================>........] - ETA: 3:51 - loss: 0.6589 - accuracy: 0.8408
77/101 [=====================>........] - ETA: 3:41 - loss: 0.6537 - accuracy: 0.8422
78/101 [======================>.......] - ETA: 3:32 - loss: 0.6460 - accuracy: 0.8442
79/101 [======================>.......] - ETA: 3:23 - loss: 0.6388 - accuracy: 0.8458
80/101 [======================>.......] - ETA: 3:14 - loss: 0.6318 - accuracy: 0.8474
81/101 [=======================>......] - ETA: 3:04 - loss: 0.6246 - accuracy: 0.8491
82/101 [=======================>......] - ETA: 2:55 - loss: 0.6179 - accuracy: 0.8506
83/101 [=======================>......] - ETA: 2:46 - loss: 0.6120 - accuracy: 0.8519
84/101 [=======================>......] - ETA: 2:37 - loss: 0.6050 - accuracy: 0.8536
85/101 [========================>.....] - ETA: 2:27 - loss: 0.5985 - accuracy: 0.8551
86/101 [========================>.....] - ETA: 2:18 - loss: 0.5934 - accuracy: 0.8564
87/101 [========================>.....] - ETA: 2:09 - loss: 0.5879 - accuracy: 0.8575
88/101 [=========================>....] - ETA: 2:00 - loss: 0.5820 - accuracy: 0.8588
89/101 [=========================>....] - ETA: 1:50 - loss: 0.5760 - accuracy: 0.8601
90/101 [=========================>....] - ETA: 1:41 - loss: 0.5715 - accuracy: 0.8612
91/101 [==========================>...] - ETA: 1:32 - loss: 0.5654 - accuracy: 0.8626
92/101 [==========================>...] - ETA: 1:23 - loss: 0.5595 - accuracy: 0.8640
93/101 [==========================>...] - ETA: 1:13 - loss: 0.5544 - accuracy: 0.8653
94/101 [==========================>...] - ETA: 1:04 - loss: 0.5514 - accuracy: 0.8661
95/101 [===========================>..] - ETA: 55s - loss: 0.5462 - accuracy: 0.8673
96/101 [===========================>..] - ETA: 46s - loss: 0.5411 - accuracy: 0.8685
97/101 [===========================>..] - ETA: 36s - loss: 0.5361 - accuracy: 0.8696
98/101 [============================>.] - ETA: 27s - loss: 0.5317 - accuracy: 0.8705
99/101 [============================>.] - ETA: 18s - loss: 0.5282 - accuracy: 0.8714
100/101 [============================>.] - ETA: 9s - loss: 0.5242 - accuracy: 0.8723
101/101 [==============================] - ETA: 0s - loss: 0.5211 - accuracy: 0.8730
2024-02-09 23:28:36.227849: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_0' with dtype string and shape [1]
[[{{node Placeholder/_0}}]]
2024-02-09 23:28:36.228303: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_1' with dtype string and shape [1]
[[{{node Placeholder/_1}}]]
101/101 [==============================] - 966s 9s/step - loss: 0.5211 - accuracy: 0.8730 - val_loss: 0.0842 - val_accuracy: 0.9700
WARNING:absl:Found untraced functions such as _update_step_xla while saving (showing 1 of 1). These functions will not be directly callable after loading.
Perform model optimization (IR) step¶
ir_path = Path("./bit_ov_model/bit_m_r50x1_1.xml")
if not ir_path.exists():
print("Initiating model optimization..!!!")
ov_model = ov.convert_model("./bit_tf_model")
ov.save_model(ov_model, ir_path)
else:
print(f"IR model {ir_path} already exists.")
Initiating model optimization..!!!
Compute accuracy of the TF model¶
tf_model = tf.keras.models.load_model("./bit_tf_model/")
tf_predictions = []
gt_label = []
for _, label in validation_dataset:
for cls_label in label:
l_list = cls_label.numpy().tolist()
gt_label.append(l_list.index(1))
for img_batch, label_batch in validation_dataset:
tf_result_batch = tf_model.predict(img_batch, verbose=0)
for i in range(len(img_batch)):
tf_result = tf_result_batch[i]
tf_result = tf.reshape(tf_result, [-1])
top5_label_idx = np.argsort(tf_result)[-MAX_PREDS::][::-1]
tf_predictions.append(top5_label_idx)
# Convert the lists to NumPy arrays for accuracy calculation
tf_predictions = np.array(tf_predictions)
gt_label = np.array(gt_label)
tf_acc_score = accuracy_score(tf_predictions, gt_label)
2024-02-09 23:29:14.482279: W tensorflow/core/common_runtime/graph_constructor.cc:812] Node 're_lu_48/PartitionedCall' has 1 outputs but the _output_shapes attribute specifies shapes for 2 outputs. Output shapes may be inaccurate.
2024-02-09 23:29:14.482323: W tensorflow/core/common_runtime/graph_constructor.cc:812] Node 'global_average_pooling2d/PartitionedCall' has 1 outputs but the _output_shapes attribute specifies shapes for 4 outputs. Output shapes may be inaccurate.
2024-02-09 23:29:16.618466: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_1' with dtype string and shape [1]
[[{{node Placeholder/_1}}]]
2024-02-09 23:29:16.618975: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1]
[[{{node Placeholder/_4}}]]
Compute accuracy of the OpenVINO model¶
ov_fp32_model = core.read_model("./bit_ov_model/bit_m_r50x1_1.xml")
ov_fp32_model.reshape([1, IMG_SIZE[0], IMG_SIZE[1], 3])
# Target device set to CPU (Other options Ex: AUTO/GPU/dGPU/)
compiled_model = ov.compile_model(ov_fp32_model)
output = compiled_model.outputs[0]
ov_predictions = []
for img_batch, _ in validation_dataset:
for image in img_batch:
image = tf.expand_dims(image, axis=0)
pred = compiled_model(image)[output]
ov_result = tf.reshape(pred, [-1])
top_label_idx = np.argsort(ov_result)[-MAX_PREDS::][::-1]
ov_predictions.append(top_label_idx)
fp32_acc_score = accuracy_score(ov_predictions, gt_label)
Quantize OpenVINO model using NNCF¶
Model Quantization using NNCF
Preprocessing and preparing validation samples for NNCF calibration
Perform NNCF Quantization on OpenVINO FP32 model
Serialize Quantized OpenVINO INT8 model
def nncf_preprocessing(image, label):
image = tf.image.resize(image, IMG_SIZE)
image = image - MEAN_RGB
image = image / STDDEV_RGB
return image
val_ds = (validation_ds.map(nncf_preprocessing, num_parallel_calls=tf.data.experimental.AUTOTUNE)
.batch(1)
.prefetch(tf.data.experimental.AUTOTUNE))
calibration_dataset = nncf.Dataset(val_ds)
ov_fp32_model = core.read_model("./bit_ov_model/bit_m_r50x1_1.xml")
ov_int8_model = nncf.quantize(ov_fp32_model, calibration_dataset, fast_bias_correction=False)
ov.serialize(ov_int8_model, "./bit_ov_int8_model/bit_m_r50x1_1_ov_int8.xml")
Output()
2024-02-09 23:29:49.936057: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_4' with dtype int64 and shape [1]
[[{{node Placeholder/_4}}]]
2024-02-09 23:29:49.937027: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_2' with dtype string and shape [1]
[[{{node Placeholder/_2}}]]
Output()
Compute accuracy of the quantized model¶
nncf_quantized_model = core.read_model("./bit_ov_int8_model/bit_m_r50x1_1_ov_int8.xml")
nncf_quantized_model.reshape([1, IMG_SIZE[0], IMG_SIZE[1], 3])
# Target device set to CPU by default
compiled_model = ov.compile_model(nncf_quantized_model)
output = compiled_model.outputs[0]
ov_predictions = []
inp_tensor = nncf_quantized_model.inputs[0]
out_tensor = nncf_quantized_model.outputs[0]
for img_batch, _ in validation_dataset:
for image in img_batch:
image = tf.expand_dims(image, axis=0)
pred = compiled_model(image)[output]
ov_result = tf.reshape(pred, [-1])
top_label_idx = np.argsort(ov_result)[-MAX_PREDS::][::-1]
ov_predictions.append(top_label_idx)
int8_acc_score = accuracy_score(ov_predictions, gt_label)
Compare FP32 and INT8 accuracy¶
print(f"Accuracy of the tensorflow model (fp32): {tf_acc_score * 100: .2f}%")
print(f"Accuracy of the OpenVINO optimized model (fp32): {fp32_acc_score * 100: .2f}%")
print(f"Accuracy of the OpenVINO quantized model (int8): {int8_acc_score * 100: .2f}%")
accuracy_drop = fp32_acc_score - int8_acc_score
print(f"Accuracy drop between OV FP32 and INT8 model: {accuracy_drop * 100:.1f}% ")
Accuracy of the tensorflow model (fp32): 97.00%
Accuracy of the OpenVINO optimized model (fp32): 97.00%
Accuracy of the OpenVINO quantized model (int8): 97.00%
Accuracy drop between OV FP32 and INT8 model: 0.0%
Compare inference results on one picture¶
# Accessing validation sample
sample_idx = 50
vds = datasets['validation']
if len(vds) > sample_idx:
sample = vds.take(sample_idx + 1).skip(sample_idx).as_numpy_iterator().next()
else:
print("Dataset does not have enough samples...!!!")
# Image data
sample_data = sample[0]
# Label info
sample_label = sample[1]
# Image data pre-processing
image = tf.image.resize(sample_data, IMG_SIZE)
image = tf.expand_dims(image, axis=0)
image = tf.cast(image, tf.float32) / 255.0
# OpenVINO inference
def ov_inference(model: ov.Model, image) -> str:
compiled_model = ov.compile_model(model)
output = compiled_model.outputs[0]
pred = compiled_model(image)[output]
ov_result = tf.reshape(pred, [-1])
pred_label = np.argsort(ov_result)[-MAX_PREDS::][::-1]
return pred_label
# OpenVINO FP32 model
ov_fp32_model = core.read_model("./bit_ov_model/bit_m_r50x1_1.xml")
ov_fp32_model.reshape([1, IMG_SIZE[0], IMG_SIZE[1], 3])
# OpenVINO INT8 model
ov_int8_model = core.read_model("./bit_ov_int8_model/bit_m_r50x1_1_ov_int8.xml")
ov_int8_model.reshape([1, IMG_SIZE[0], IMG_SIZE[1], 3])
# OpenVINO FP32 model inference
ov_fp32_pred_label = ov_inference(ov_fp32_model, image)
print(f"Predicted label for the sample picture by float (fp32) model: {label_func(class_idx_dict[int(ov_fp32_pred_label)])}\n")
# OpenVINO FP32 model inference
ov_int8_pred_label = ov_inference(ov_int8_model, image)
print(f"Predicted label for the sample picture by qunatized (int8) model: {label_func(class_idx_dict[int(ov_int8_pred_label)])}\n")
# Plotting the image sample with ground truth
plt.figure()
plt.imshow(sample_data)
plt.title(f"Ground truth: {label_func(class_idx_dict[sample_label])}")
plt.axis('off')
plt.show()
2024-02-09 23:30:17.827051: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_1' with dtype string and shape [1]
[[{{node Placeholder/_1}}]]
2024-02-09 23:30:17.827641: I tensorflow/core/common_runtime/executor.cc:1197] [/device:CPU:0] (DEBUG INFO) Executor start aborting (this does not indicate an error and you can ignore this message): INVALID_ARGUMENT: You must feed a value for placeholder tensor 'Placeholder/_0' with dtype string and shape [1]
[[{{node Placeholder/_0}}]]
Predicted label for the sample picture by float (fp32) model: gas pump
Predicted label for the sample picture by qunatized (int8) model: gas pump
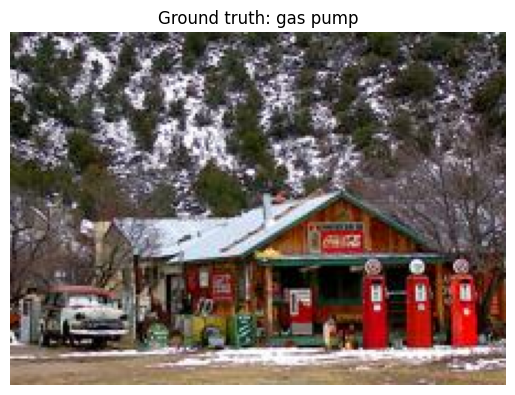