Overview of Transformations API¶
OpenVINO Transformation mechanism allows to develop transformation passes to modify ov::Model
. You can use this mechanism to apply additional optimizations to the original Model or transform unsupported subgraphs and operations to new operations which are supported by the plugin. This guide contains all necessary information that you need to start implementing OpenVINO™ transformations.
Working with Model¶
Before the moving to transformation part it is needed to say several words about functions which allow to modify ov::Model
. This chapter extends the model representation guide and shows an API that allows us to manipulate with ov::Model
.
Working with node input and output ports¶
First of all let’s talk about ov::Node
input/output ports. Each OpenVINO™ operation has input and output ports except cases when operation has Parameter
or Constant
type.
Every port belongs to its node, so using a port we can access parent node, get shape and type for particular input/output, get all consumers in case of output port, and get producer node in case of input port. With output port we can set inputs for newly created operations.
Lets look at the code example.
// Let's suppose that node is opset8::Convolution operation
// as we know opset8::Convolution has two input ports (data, weights) and one output port
ov::Input<ov::Node> data = node->input(0);
ov::Input<ov::Node> weights = node->input(1);
ov::Output<ov::Node> output = node->output(0);
// Getting shape and type
auto pshape = data.get_partial_shape();
auto el_type = data.get_element_type();
// Getting parent for input port
ov::Output<ov::Node> parent_output;
parent_output = data.get_source_output();
// Another short way to get partent for output port
parent_output = node->input_value(0);
// Getting all consumers for output port
auto consumers = output.get_target_inputs();
Node replacement¶
OpenVINO™ provides two ways for node replacement: via OpenVINO™ helper function and directly via port methods. We are going to review both of them.
Let’s start with OpenVINO™ helper functions. The most popular function is ov::replace_node(old_node, new_node)
.
We will review real replacement case where Negative operation is replaced with Multiply.
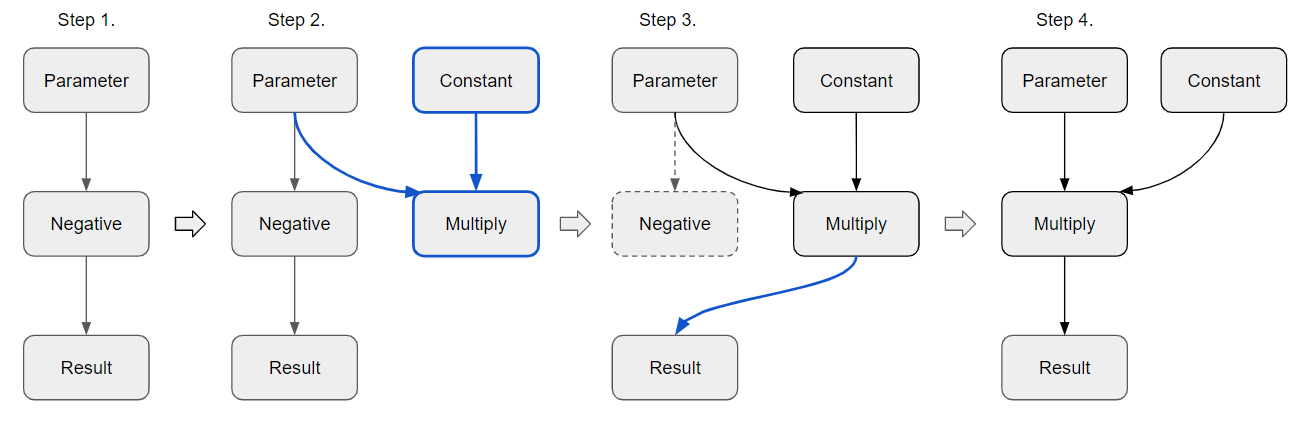
bool ov_replace_node(std::shared_ptr<ov::Node> node) {
// Step 1. Verify that node has opset8::Negative type
auto neg = std::dynamic_pointer_cast<ov::opset8::Negative>(node);
if (!neg) {
return false;
}
// Step 2. Create opset8::Multiply operation where the first input is negative operation input and second as Constant with -1 value
auto mul = std::make_shared<ov::opset8::Multiply>(neg->input_value(0),
ov::opset8::Constant::create(neg->get_element_type(), ov::Shape{1}, {-1}));
mul->set_friendly_name(neg->get_friendly_name());
ov::copy_runtime_info(neg, mul);
// Step 3. Replace Negative operation with Multiply operation
ov::replace_node(neg, mul);
return true;
// Step 4. Negative operation will be removed automatically because all consumers was moved to Multiply operation
}
ov::replace_node
has a constraint that number of output ports for both of ops must be the same; otherwise, it raises an exception.
The alternative way to do the same replacement is the following:
// All neg->output(0) consumers will be moved to mul->output(0) port
neg->output(0).replace(mul->output(0));
Another transformation example is insertion.
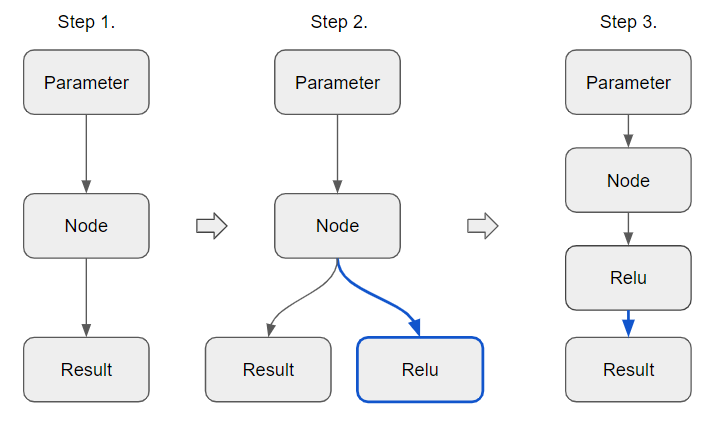
// Step 1. Lets suppose that we have a node with single output port and we want to insert additional operation new_node after it
void insert_example(std::shared_ptr<ov::Node> node) {
// Get all consumers for node
auto consumers = node->output(0).get_target_inputs();
// Step 2. Create new node. Let it be opset8::Relu.
auto new_node = std::make_shared<ov::opset8::Relu>(node);
// Step 3. Reconnect all consumers to new_node
for (auto input : consumers) {
input.replace_source_output(new_node);
}
}
The alternative way to the insert operation is to make a node copy and use ov::replace_node()
:
void insert_example_with_copy(std::shared_ptr<ov::Node> node) {
// Make a node copy
auto node_copy = node->clone_with_new_inputs(node->input_values());
// Create new node
auto new_node = std::make_shared<ov::opset8::Relu>(node_copy);
ov::replace_node(node, new_node);
}
Node elimination¶
Another type of node replacement is its elimination.
To eliminate operation, OpenVINO™ has special method that considers all limitations related to OpenVINO™ Runtime.
// Suppose we have a node that we want to remove
bool success = ov::replace_output_update_name(node->output(0), node->input_value(0));
ov::replace_output_update_name()
in case of successful replacement it automatically preserves friendly name and runtime info.
Transformations types¶
OpenVINO™ Runtime has three main transformation types:
Model pass - straightforward way to work with
ov::Model
directlyMatcher pass - pattern-based transformation approach
Graph rewrite pass - container for matcher passes needed for efficient execution
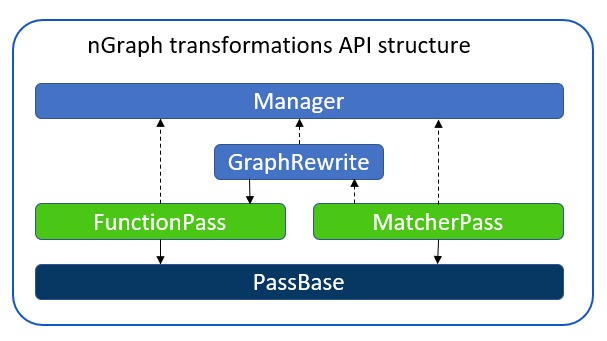
Transformation conditional compilation¶
Transformation library has two internal macros to support conditional compilation feature.
MATCHER_SCOPE(region)
- allows to disable the MatcherPass if matcher isn’t used. The region name should be unique. This macro creates a local variablematcher_name
which you should use as a matcher name.RUN_ON_MODEL_SCOPE(region)
- allows to disable run_on_model pass if it isn’t used. The region name should be unique.
Transformation writing essentials¶
When developing a transformation, you need to follow these transformation rules:
1. Friendly Names¶
Each ov::Node
has an unique name and a friendly name. In transformations we care only about friendly name because it represents the name from the model. To avoid losing friendly name when replacing node with other node or subgraph, set the original friendly name to the latest node in replacing subgraph. See the example below.
// Replace Div operation with Power and Multiply sub-graph and set original friendly name to Multiply operation
auto pow = std::make_shared<ov::opset8::Power>(div->input(1).get_source_output(),
ov::op::v0::Constant::create(div->get_input_element_type(1), ov::Shape{1}, {-1}));
auto mul = std::make_shared<ov::opset8::Multiply>(div->input(0).get_source_output(), pow);
mul->set_friendly_name(div->get_friendly_name());
ngraph::replace_node(div, mul);
In more advanced cases, when replaced operation has several outputs and we add additional consumers to its outputs, we make a decision how to set friendly name by arrangement.
2. Runtime Info¶
Runtime info is a map std::map<std::string, ov::Any>
located inside ov::Node
class. It represents additional attributes in ov::Node
. These attributes can be set by users or by plugins and when executing transformation that changes ov::Model
we need to preserve these attributes as they will not be automatically propagated. In most cases, transformations have the following types: 1:1 (replace node with another node), 1:N (replace node with a sub-graph), N:1 (fuse sub-graph into a single node), N:M (any other transformation). Currently, there is no mechanism that automatically detects transformation types, so we need to propagate this runtime information manually. See the examples below.
// Replace Transpose with Reshape operation (1:1)
ov::copy_runtime_info(transpose, reshape);
// Replace Div operation with Power and Multiply sub-graph (1:N)
ov::copy_runtime_info(div, {pow, mul});
// Fuse Convolution with Add operation (N:1)
ov::copy_runtime_info({conv, bias}, {conv_fused});
// Any other transformation that replaces one sub-graph with another sub-graph (N:M)
ov::copy_runtime_info({a, b, c}, {e, f});
When transformation has multiple fusions or decompositions, ov::copy_runtime_info
must be called multiple times for each case.
Note : copy_runtime_info removes rt_info from destination nodes. If you want to keep it, you need to specify them in source nodes like this: copy_runtime_info({a, b, c}, {a, b})
3. Constant Folding¶
If your transformation inserts constant sub-graphs that need to be folded, do not forget to use ov::pass::ConstantFolding()
after your transformation or call constant folding directly for operation. The example below shows how constant subgraph can be constructed.
// After ConstantFolding pass Power will be replaced with Constant
auto input = std::make_shared<ov::opset8::Parameter>(ov::element::f32, ov::Shape{1});
auto pow = std::make_shared<ov::opset8::Power>(ov::opset8::Constant::create(ov::element::f32, ov::Shape{1}, {2}),
ov::opset8::Constant::create(ov::element::f32, ov::Shape{1}, {3}));
auto mul = std::make_shared<ov::opset8::Multiply>(input /\* not constant input \*/, pow);
Manual constant folding is more preferable than ov::pass::ConstantFolding()
because it is much faster.
Below you can find an example of manual constant folding:
template <class T>
ov::Output<ov::Node> eltwise_fold(const ov::Output<ov::Node>& input0, const ov::Output<ov::Node>& input1) {
auto eltwise = std::make_shared<T>(input0, input1);
ov::OutputVector output(eltwise->get_output_size());
// If constant folding wasn't successful return eltwise output
if (!eltwise->constant_fold(output, {input0, input1})) {
return eltwise->output(0);
}
return output[0];
}
Common mistakes in transformations¶
In transformation development process:
Do not use deprecated OpenVINO™ API. Deprecated methods has the
OPENVINO_DEPRECATED
macros in its definition.Do not pass
shared_ptr<Node>
as an input for other node if type of node is unknown or it has multiple outputs. Use explicit output port.If you replace node with another node that produces different shape, remember that new shape will not be propagated until the first
validate_nodes_and_infer_types
call forov::Model
. If you are usingov::pass::Manager
, it will automatically call this method after each transformation execution.Do not forget to call the
ov::pass::ConstantFolding
pass if your transformation creates constant subgraphs.Use latest OpSet if you are not developing downgrade transformation pass.
When developing a callback for
ov::pass::MatcherPass
, do not change nodes that come after the root node in topological order.
Using pass manager¶
ov::pass::Manager
is a container class that can store the list of transformations and execute them. The main idea of this class is to have high-level representation for grouped list of transformations. It can register and apply any transformation pass on model. In addition, ov::pass::Manager
has extended debug capabilities (find more information in the how to debug transformations section).
The example below shows basic usage of ov::pass::Manager
ov::pass::Manager manager;
manager.register_pass<ov::pass::MyModelTransformation>();
// Two matchers will run independently (two independent graph traversals)
// pass::Manager automatically creates GraphRewrite container for each MatcherPass
manager.register_pass<ov::pass::DecomposeDivideMatcher>();
manager.register_pass<ov::pass::ReluReluFusionMatcher>();
manager.run_passes(f);
Another example shows how multiple matcher passes can be united into single GraphRewrite.
// Register anchor GraphRewrite pass inside manager that will execute two matchers simultaneously
ov::pass::Manager manager;
auto anchor = manager.register_pass<ov::pass::GraphRewrite>();
anchor->add_matcher<ov::pass::DecomposeDivideMatcher>();
anchor->add_matcher<ov::pass::ReluReluFusionMatcher>();
manager.run_passes(f);
How to debug transformations¶
If you are using ngraph::pass::Manager
to run sequence of transformations, you can get additional debug capabilities by using the following environment variables:
OV_PROFILE_PASS_ENABLE=1 - enables performance measurement for each transformation and prints execution status
OV_ENABLE_VISUALIZE_TRACING=1 - enables visualization after each transformation. By default, it saves dot and svg files.
Note : Make sure that you have dot installed on your machine; otherwise, it will silently save only dot file without svg file.